mirror of
https://gitee.com/zoujingli/WeChatDeveloper.git
synced 2025-04-05 19:41:44 +08:00
commit
038dde16ac
8
.gitignore
vendored
8
.gitignore
vendored
@ -1,5 +1,9 @@
|
||||
/.idea
|
||||
/.git
|
||||
/.idea
|
||||
/.DS_Store
|
||||
/vendor
|
||||
/WeChat/Cache
|
||||
/Cache
|
||||
/Test/cert
|
||||
/nbproject
|
||||
/composer.lock
|
||||
/_test/cert
|
48
AliPay/App.php
Normal file
48
AliPay/App.php
Normal file
@ -0,0 +1,48 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
namespace AliPay;
|
||||
|
||||
use WeChat\Contracts\BasicAliPay;
|
||||
|
||||
/**
|
||||
* 支付宝App支付网关
|
||||
* Class App
|
||||
* @package AliPay
|
||||
*/
|
||||
class App extends BasicAliPay
|
||||
{
|
||||
|
||||
/**
|
||||
* App constructor.
|
||||
* @param array $options
|
||||
*/
|
||||
public function __construct(array $options)
|
||||
{
|
||||
parent::__construct($options);
|
||||
$this->options->set('method', 'alipay.trade.app.pay');
|
||||
$this->params->set('product_code', 'QUICK_MSECURITY_PAY');
|
||||
}
|
||||
|
||||
/**
|
||||
* 创建数据操作
|
||||
* @param array $options
|
||||
* @return string
|
||||
*/
|
||||
public function apply($options)
|
||||
{
|
||||
$this->applyData($options);
|
||||
return http_build_query($this->options->get());
|
||||
}
|
||||
}
|
47
AliPay/Bill.php
Normal file
47
AliPay/Bill.php
Normal file
@ -0,0 +1,47 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
namespace AliPay;
|
||||
|
||||
use WeChat\Contracts\BasicAliPay;
|
||||
|
||||
/**
|
||||
* 支付宝电子面单下载
|
||||
* Class Bill
|
||||
* @package AliPay
|
||||
*/
|
||||
class Bill extends BasicAliPay
|
||||
{
|
||||
/**
|
||||
* Bill constructor.
|
||||
* @param array $options
|
||||
*/
|
||||
public function __construct(array $options)
|
||||
{
|
||||
parent::__construct($options);
|
||||
$this->options->set('method', 'alipay.data.dataservice.bill.downloadurl.query');
|
||||
}
|
||||
|
||||
/**
|
||||
* 创建数据操作
|
||||
* @param array $options
|
||||
* @return mixed
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function apply($options)
|
||||
{
|
||||
return $this->getResult($options);
|
||||
}
|
||||
}
|
48
AliPay/Pos.php
Normal file
48
AliPay/Pos.php
Normal file
@ -0,0 +1,48 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
namespace AliPay;
|
||||
|
||||
use WeChat\Contracts\BasicAliPay;
|
||||
|
||||
/**
|
||||
* 支付宝刷卡支付
|
||||
* Class Pos
|
||||
* @package AliPay
|
||||
*/
|
||||
class Pos extends BasicAliPay
|
||||
{
|
||||
/**
|
||||
* Pos constructor.
|
||||
* @param array $options
|
||||
*/
|
||||
public function __construct(array $options)
|
||||
{
|
||||
parent::__construct($options);
|
||||
$this->options->set('method', 'alipay.trade.pay');
|
||||
$this->params->set('product_code', 'FACE_TO_FACE_PAYMENT');
|
||||
}
|
||||
|
||||
/**
|
||||
* 创建数据操作
|
||||
* @param array $options
|
||||
* @return mixed
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function apply($options)
|
||||
{
|
||||
return $this->getResult($options);
|
||||
}
|
||||
}
|
47
AliPay/Scan.php
Normal file
47
AliPay/Scan.php
Normal file
@ -0,0 +1,47 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
namespace AliPay;
|
||||
|
||||
use WeChat\Contracts\BasicAliPay;
|
||||
|
||||
/**
|
||||
* 支付宝扫码支付
|
||||
* Class Scan
|
||||
* @package AliPay
|
||||
*/
|
||||
class Scan extends BasicAliPay
|
||||
{
|
||||
/**
|
||||
* Scan constructor.
|
||||
* @param array $options
|
||||
*/
|
||||
public function __construct(array $options)
|
||||
{
|
||||
parent::__construct($options);
|
||||
$this->options->set('method', 'alipay.trade.precreate');
|
||||
}
|
||||
|
||||
/**
|
||||
* 创建数据操作
|
||||
* @param array $options
|
||||
* @return mixed
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function apply($options)
|
||||
{
|
||||
return $this->getResult($options);
|
||||
}
|
||||
}
|
48
AliPay/Transfer.php
Normal file
48
AliPay/Transfer.php
Normal file
@ -0,0 +1,48 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
namespace AliPay;
|
||||
|
||||
use WeChat\Contracts\BasicAliPay;
|
||||
|
||||
/**
|
||||
* 支付宝转账到账户
|
||||
* Class Transfer
|
||||
* @package AliPay
|
||||
*/
|
||||
class Transfer extends BasicAliPay
|
||||
{
|
||||
|
||||
/**
|
||||
* Transfer constructor.
|
||||
* @param array $options
|
||||
*/
|
||||
public function __construct(array $options)
|
||||
{
|
||||
parent::__construct($options);
|
||||
$this->options->set('method', 'alipay.fund.trans.toaccount.transfer');
|
||||
}
|
||||
|
||||
/**
|
||||
* 创建数据操作
|
||||
* @param array $options
|
||||
* @return mixed
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function apply($options)
|
||||
{
|
||||
return $this->getResult($options);
|
||||
}
|
||||
}
|
47
AliPay/Wap.php
Normal file
47
AliPay/Wap.php
Normal file
@ -0,0 +1,47 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
namespace AliPay;
|
||||
|
||||
use WeChat\Contracts\BasicAliPay;
|
||||
|
||||
/**
|
||||
* 手机WAP网站支付支持
|
||||
* Class Wap
|
||||
* @package AliPay
|
||||
*/
|
||||
class Wap extends BasicAliPay
|
||||
{
|
||||
/**
|
||||
* Wap constructor.
|
||||
* @param array $options
|
||||
*/
|
||||
public function __construct(array $options)
|
||||
{
|
||||
parent::__construct($options);
|
||||
$this->options->set('method', 'alipay.trade.wap.pay');
|
||||
$this->params->set('product_code', 'QUICK_WAP_WAY');
|
||||
}
|
||||
|
||||
/**
|
||||
* 创建数据操作
|
||||
* @param array $options
|
||||
* @return string
|
||||
*/
|
||||
public function apply($options)
|
||||
{
|
||||
parent::applyData($options);
|
||||
return $this->buildPayHtml();
|
||||
}
|
||||
}
|
47
AliPay/Web.php
Normal file
47
AliPay/Web.php
Normal file
@ -0,0 +1,47 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
namespace AliPay;
|
||||
|
||||
use WeChat\Contracts\BasicAliPay;
|
||||
|
||||
/**
|
||||
* 支付宝网站支付
|
||||
* Class Web
|
||||
* @package AliPay
|
||||
*/
|
||||
class Web extends BasicAliPay
|
||||
{
|
||||
/**
|
||||
* Web constructor.
|
||||
* @param array $options
|
||||
*/
|
||||
public function __construct(array $options)
|
||||
{
|
||||
parent::__construct($options);
|
||||
$this->options->set('method', 'alipay.trade.page.pay');
|
||||
$this->params->set('product_code', 'FAST_INSTANT_TRADE_PAY');
|
||||
}
|
||||
|
||||
/**
|
||||
* 创建数据操作
|
||||
* @param array $options
|
||||
* @return string
|
||||
*/
|
||||
public function apply($options)
|
||||
{
|
||||
parent::applyData($options);
|
||||
return $this->buildPayHtml();
|
||||
}
|
||||
}
|
136
README.md
136
README.md
@ -1,136 +0,0 @@
|
||||
[](https://packagist.org/packages/wechat-developer) [](https://packagist.org/packages/zoujingli/wechat-developer) [](https://packagist.org/packages/wechat-developer) [](https://packagist.org/packages/wechat-developer)
|
||||
|
||||
WeChatDeveloper for PHP
|
||||
--
|
||||
* WeChatDeveloper 是基于 [wechat-php-sdk](https://github.com/zoujingli/wechat-php-sdk) 重构,优化并完善;
|
||||
* 运行最底要求 PHP 版本 5.4 , 建议在 PHP7 上运行以获取最佳性能;
|
||||
* WeChatDeveloper 针对 access_token 失效增加了自动刷新机制;
|
||||
* 微信的部分接口需要缓存数据在本地,因此对目录需要有写权限;
|
||||
* 我们鼓励大家使用 composer 来管理您的第三方库,方便后期更新操作;
|
||||
* WeChatDeveloper 已历经数个线上项目考验,欢迎 fork 或 star 此项目。
|
||||
|
||||
|
||||
Documentation
|
||||
--
|
||||
PHP开发技术交流(QQ群 513350915)
|
||||
|
||||
[](http://shang.qq.com/wpa/qunwpa?idkey=ae25cf789dafbef62e50a980ffc31242f150bc61a61164458216dd98c411832a)
|
||||
|
||||
> WeChatDeveloper 是基于官方接口封装,在做微信开发前,必需先阅读微信官方文档。
|
||||
>* 微信官方文档:http://mp.weixin.qq.com/wiki
|
||||
>* 商户支付文档:https://pay.weixin.qq.com/wiki/doc/api/index.html
|
||||
|
||||
> 针对 WeChatDeveloper 也有一准备了帮助资料可供参考。
|
||||
>* 开发文档地址:http://www.kancloud.cn/zoujingli/wechat-developer
|
||||
>* Think.Admin:https://github.com/zoujingli/Think.Admin
|
||||
|
||||
|
||||
Repositorie
|
||||
--
|
||||
WeChatDeveloper 为开源项目,允许把它用于任何地方,不受任何约束,欢迎 fork 项目。
|
||||
>* GitHub 托管地址:https://github.com/zoujingli/WeChatDeveloper
|
||||
>* OSChina 托管地址:http://git.oschina.net/zoujingli/WeChatDeveloper
|
||||
|
||||
|
||||
Install
|
||||
--
|
||||
1.1 通过 Composer 来管理安装
|
||||
```shell
|
||||
# 首次安装 线上版本(稳定)
|
||||
composer require zoujingli/wechat-developer
|
||||
|
||||
# 首次安装 开发版本
|
||||
composer require zoujingli/wechat-developer dev-master
|
||||
|
||||
# 更新 WeChatDeveloper
|
||||
composer update zoujingli/wechat-developer
|
||||
```
|
||||
|
||||
1.2 如果不使用 Composer, 可以下载 WeChatDeveloper 并解压到项目中
|
||||
```php
|
||||
# 在项目中加载初始化文件
|
||||
include "您的目录/WeChatDeveloper/include.php";
|
||||
```
|
||||
|
||||
2.1 接口实例所需参数
|
||||
```php
|
||||
$config = [
|
||||
'token' => 'test',
|
||||
'appid' => 'wx60a43dd8161666d4',
|
||||
'appsecret' => '71308e96a204296c57d7cd4b21b883e8',
|
||||
'encodingaeskey' => 'BJIUzE0gqlWy0GxfPp4J1oPTBmOrNDIGPNav1YFH5Z5',
|
||||
// 配置商户支付参数(可选,在使用支付功能时需要)
|
||||
'mch_id' => "1235704602",
|
||||
'mch_key' => 'IKI4kpHjU94ji3oqre5zYaQMwLHuZPmj',
|
||||
// 配置商户支付双向证书目录(可选,在使用退款|打款|红包时需要)
|
||||
'ssl_key' => '',
|
||||
'ssl_cer' => '',
|
||||
// 缓存目录配置(可选,需拥有读写权限)
|
||||
'cache_path' => '',
|
||||
];
|
||||
```
|
||||
|
||||
3.1 实例指定接口
|
||||
```php
|
||||
try {
|
||||
|
||||
// 实例对应的接口对象
|
||||
$user = new \WeChat\User($config);
|
||||
|
||||
// 调用接口对象方法
|
||||
$list = $user->getUserList();
|
||||
|
||||
// 处理返回的结果
|
||||
echo '<pre>';
|
||||
var_export($list);
|
||||
|
||||
} catch (Exception $e) {
|
||||
|
||||
// 出错啦,处理下吧
|
||||
echo $e->getMessage() . PHP_EOL;
|
||||
|
||||
}
|
||||
```
|
||||
|
||||
Encapsulation
|
||||
--
|
||||
* 接入验证 (初级权限)
|
||||
* 自动回复(文本、图片、语音、视频、音乐、图文) (初级权限)
|
||||
* 菜单操作(查询、创建、删除) (菜单权限)
|
||||
* 客服消息(文本、图片、语音、视频、音乐、图文) (认证权限)
|
||||
* 二维码(创建临时、永久二维码,获取二维码URL) (服务号、认证权限)
|
||||
* 长链接转短链接接口 (服务号、认证权限)
|
||||
* 标签操作(查询、创建、修改、移动用户到标签) (认证权限)
|
||||
* 网页授权(基本授权,用户信息授权) (服务号、认证权限)
|
||||
* 用户信息(查询用户基本信息、获取关注者列表) (认证权限)
|
||||
* 多客服功能(客服管理、获取客服记录、客服会话管理) (认证权限)
|
||||
* 媒体文件(上传、获取) (认证权限)
|
||||
* 高级群发 (认证权限)
|
||||
* 模板消息(设置所属行业、添加模板、发送模板消息) (服务号、认证权限)
|
||||
* 卡券管理(创建、修改、删除、发放、门店管理等) (认证权限)
|
||||
* 语义理解 (服务号、认证权限)
|
||||
* 获取微信服务器IP列表 (初级权限)
|
||||
* 微信JSAPI授权(获取ticket、获取签名) (初级权限)
|
||||
* 数据统计(用户、图文、消息、接口分析数据) (认证权限)
|
||||
* 微信支付(网页支付、扫码支付、交易退款、给粉丝打款)(认证服务号并开通支付功能)
|
||||
|
||||
|
||||
Permission
|
||||
--
|
||||
* 初级权限:基本权限,任何正常的公众号都有此权限
|
||||
* 菜单权限:正常的服务号、认证后的订阅号拥有此权限
|
||||
* 认证权限:分为订阅号、服务号认证,如前缀服务号则仅认证的服务号有此权限
|
||||
* 支付权限:仅认证后的服务号可以申请此权限
|
||||
|
||||
|
||||
Copyright
|
||||
--
|
||||
* WeChatDeveloper 基于`MIT`协议发布,任何人可以用在任何地方,不受约束
|
||||
* WeChatDeveloper 部分代码来自互联网,若有异议,可以联系作者进行删除
|
||||
|
||||
|
||||
Sponsor
|
||||
--
|
||||

|
||||
|
||||
|
134
We.php
Normal file
134
We.php
Normal file
@ -0,0 +1,134 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
use WeChat\Contracts\DataArray;
|
||||
use WeChat\Exceptions\InvalidInstanceException;
|
||||
|
||||
/**
|
||||
* 加载缓存器
|
||||
*
|
||||
* Class We
|
||||
* @library WeChatDeveloper
|
||||
* @author Anyon<zoujingli@qq.com>
|
||||
* @date 2018/05/24 13:23
|
||||
*
|
||||
* ----- WeChat -----
|
||||
* @method \WeChat\Card WeChatCard($options = []) static 微信卡券管理
|
||||
* @method \WeChat\Custom WeChatCustom($options = []) static 微信客服消息
|
||||
* @method \WeChat\Limit WeChatLimit($options = []) static 接口调用频次限制
|
||||
* @method \WeChat\Media WeChatMedia($options = []) static 微信素材管理
|
||||
* @method \WeChat\Menu WeChatMenu($options = []) static 微信菜单管理
|
||||
* @method \WeChat\Oauth WeChatOauth($options = []) static 微信网页授权
|
||||
* @method \WeChat\Pay WeChatPay($options = []) static 微信支付商户
|
||||
* @method \WeChat\Product WeChatProduct($options = []) static 微信商店管理
|
||||
* @method \WeChat\Qrcode WeChatQrcode($options = []) static 微信二维码管理
|
||||
* @method \WeChat\Receive WeChatReceive($options = []) static 微信推送管理
|
||||
* @method \WeChat\Scan WeChatScan($options = []) static 微信扫一扫接入管理
|
||||
* @method \WeChat\Script WeChatScript($options = []) static 微信前端支持
|
||||
* @method \WeChat\Shake WeChatShake($options = []) static 微信揺一揺周边
|
||||
* @method \WeChat\Tags WeChatTags($options = []) static 微信用户标签管理
|
||||
* @method \WeChat\Template WeChatTemplate($options = []) static 微信模板消息
|
||||
* @method \WeChat\User WeChatUser($options = []) static 微信粉丝管理
|
||||
* @method \WeChat\Wifi WeChatWifi($options = []) static 微信门店WIFI管理
|
||||
*
|
||||
* ----- WeMini -----
|
||||
* @method \WeMini\Crypt WeMiniCrypt($options = []) static 小程序数据加密处理
|
||||
* @method \WeMini\Delivery WeMiniDelivery($options = []) static 小程序即时配送
|
||||
* @method \WeMini\Image WeMiniImage($options = []) static 小程序图像处理
|
||||
* @method \WeMini\Logistics WeMiniLogistics($options = []) static 小程序物流助手
|
||||
* @method \WeMini\Message WeMiniMessage($options = []) static 小程序动态消息
|
||||
* @method \WeMini\Ocr WeMiniOcr($options = []) static 小程序ORC服务
|
||||
* @method \WeMini\Plugs WeMiniPlugs($options = []) static 小程序插件管理
|
||||
* @method \WeMini\Poi WeMiniPoi($options = []) static 小程序地址管理
|
||||
* @method \WeMini\Qrcode WeMiniQrcode($options = []) static 小程序二维码管理
|
||||
* @method \WeMini\Security WeMiniSecurity($options = []) static 小程序内容安全
|
||||
* @method \WeMini\Soter WeMiniSoter($options = []) static 小程序生物认证
|
||||
* @method \WeMini\Template WeMiniTemplate($options = []) static 小程序模板消息支持
|
||||
* @method \WeMini\Total WeMiniTotal($options = []) static 小程序数据接口
|
||||
*
|
||||
* ----- WePay -----
|
||||
* @method \WePay\Bill WePayBill($options = []) static 微信商户账单及评论
|
||||
* @method \WePay\Order WePayOrder($options = []) static 微信商户订单
|
||||
* @method \WePay\Refund WePayRefund($options = []) static 微信商户退款
|
||||
* @method \WePay\Coupon WePayCoupon($options = []) static 微信商户代金券
|
||||
* @method \WePay\Redpack WePayRedpack($options = []) static 微信红包支持
|
||||
* @method \WePay\Transfers WePayTransfers($options = []) static 微信商户打款到零钱
|
||||
* @method \WePay\TransfersBank WePayTransfersBank($options = []) static 微信商户打款到银行卡
|
||||
*
|
||||
* ----- AliPay ----
|
||||
* @method \AliPay\App AliPayApp($options) static 支付宝App支付网关
|
||||
* @method \AliPay\Bill AliPayBill($options) static 支付宝电子面单下载
|
||||
* @method \AliPay\Pos AliPayPos($options) static 支付宝刷卡支付
|
||||
* @method \AliPay\Scan AliPayScan($options) static 支付宝扫码支付
|
||||
* @method \AliPay\Transfer AliPayTransfer($options) static 支付宝转账到账户
|
||||
* @method \AliPay\Wap AliPayWap($options) static 支付宝手机网站支付
|
||||
* @method \AliPay\Web AliPayWeb($options) static 支付宝网站支付
|
||||
*/
|
||||
class We
|
||||
{
|
||||
/**
|
||||
* 定义当前版本
|
||||
* @var string
|
||||
*/
|
||||
const VERSION = '1.2.13';
|
||||
|
||||
/**
|
||||
* 静态配置
|
||||
* @var DataArray
|
||||
*/
|
||||
private static $config;
|
||||
|
||||
/**
|
||||
* 设置及获取参数
|
||||
* @param array $option
|
||||
* @return array
|
||||
*/
|
||||
public static function config($option = null)
|
||||
{
|
||||
if (is_array($option)) {
|
||||
self::$config = new DataArray($option);
|
||||
}
|
||||
if (self::$config instanceof DataArray) {
|
||||
return self::$config->get();
|
||||
}
|
||||
return [];
|
||||
}
|
||||
|
||||
/**
|
||||
* 静态魔术加载方法
|
||||
* @param string $name 静态类名
|
||||
* @param array $arguments 参数集合
|
||||
* @return mixed
|
||||
* @throws InvalidInstanceException
|
||||
*/
|
||||
public static function __callStatic($name, $arguments)
|
||||
{
|
||||
if (substr($name, 0, 6) === 'WeChat') {
|
||||
$class = 'WeChat\\' . substr($name, 6);
|
||||
} elseif (substr($name, 0, 6) === 'WeMini') {
|
||||
$class = 'WeMini\\' . substr($name, 6);
|
||||
} elseif (substr($name, 0, 6) === 'AliPay') {
|
||||
$class = 'AliPay\\' . substr($name, 6);
|
||||
} elseif (substr($name, 0, 5) === 'WePay') {
|
||||
$class = 'WePay\\' . substr($name, 5);
|
||||
}
|
||||
if (!empty($class) && class_exists($class)) {
|
||||
$option = array_shift($arguments);
|
||||
$config = is_array($option) ? $option : self::$config->get();
|
||||
return new $class($config);
|
||||
}
|
||||
throw new InvalidInstanceException("class {$name} not found");
|
||||
}
|
||||
|
||||
}
|
@ -14,7 +14,6 @@
|
||||
|
||||
namespace WeChat;
|
||||
|
||||
|
||||
use WeChat\Contracts\BasicWeChat;
|
||||
|
||||
/**
|
||||
@ -388,6 +387,65 @@ class Card extends BasicWeChat
|
||||
return $this->httpPostForJson($url, $data);
|
||||
}
|
||||
|
||||
|
||||
/**
|
||||
* 激活会员卡
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws Exceptions\InvalidResponseException
|
||||
* @throws Exceptions\LocalCacheException
|
||||
*/
|
||||
public function activateMemberCard(array $data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/card/membercard/activate?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->httpPostForJson($url, $data);
|
||||
}
|
||||
|
||||
/**
|
||||
* 设置开卡字段接口
|
||||
* 用户激活时需要填写的选项
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws Exceptions\InvalidResponseException
|
||||
* @throws Exceptions\LocalCacheException
|
||||
*/
|
||||
public function setActivateMemberCardUser(array $data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/card/membercard/activateuserform/set?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->httpPostForJson($url, $data);
|
||||
}
|
||||
|
||||
/**
|
||||
* 获取用户提交资料
|
||||
* 根据activate_ticket获取到用户填写的信息
|
||||
* @param string $activate_ticket
|
||||
* @return array
|
||||
* @throws Exceptions\InvalidResponseException
|
||||
* @throws Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getActivateMemberCardTempinfo($activate_ticket)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/card/membercard/activatetempinfo/get?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->httpPostForJson($url, ['activate_ticket' => $activate_ticket]);
|
||||
}
|
||||
|
||||
/**
|
||||
* 更新会员信息
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws Exceptions\InvalidResponseException
|
||||
* @throws Exceptions\LocalCacheException
|
||||
*/
|
||||
public function updateMemberCardUser(array $data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/card/membercard/updateuser?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->httpPostForJson($url, $data);
|
||||
}
|
||||
|
||||
/**
|
||||
* 拉取会员卡概况数据接口
|
||||
* @param string $begin_date 查询数据的起始时间
|
||||
@ -611,6 +669,4 @@ class Card extends BasicWeChat
|
||||
return $this->httpPostForJson($url, $data);
|
||||
}
|
||||
|
||||
|
||||
|
||||
}
|
286
WeChat/Contracts/BasicAliPay.php
Normal file
286
WeChat/Contracts/BasicAliPay.php
Normal file
@ -0,0 +1,286 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
namespace WeChat\Contracts;
|
||||
|
||||
use WeChat\Exceptions\InvalidArgumentException;
|
||||
use WeChat\Exceptions\InvalidResponseException;
|
||||
|
||||
/**
|
||||
* 支付宝支付基类
|
||||
* Class AliPay
|
||||
* @package AliPay\Contracts
|
||||
*/
|
||||
abstract class BasicAliPay
|
||||
{
|
||||
|
||||
/**
|
||||
* 支持配置
|
||||
* @var DataArray
|
||||
*/
|
||||
protected $config;
|
||||
|
||||
/**
|
||||
* 当前请求数据
|
||||
* @var DataArray
|
||||
*/
|
||||
protected $options;
|
||||
|
||||
/**
|
||||
* DzContent数据
|
||||
* @var DataArray
|
||||
*/
|
||||
protected $params;
|
||||
|
||||
/**
|
||||
* 静态缓存
|
||||
* @var static
|
||||
*/
|
||||
protected static $cache;
|
||||
|
||||
/**
|
||||
* 正常请求网关
|
||||
* @var string
|
||||
*/
|
||||
protected $gateway = 'https://openapi.alipay.com/gateway.do?charset=utf-8';
|
||||
|
||||
/**
|
||||
* AliPay constructor.
|
||||
* @param array $options
|
||||
*/
|
||||
public function __construct($options)
|
||||
{
|
||||
$this->params = new DataArray([]);
|
||||
$this->config = new DataArray($options);
|
||||
if (empty($options['appid'])) {
|
||||
throw new InvalidArgumentException("Missing Config -- [appid]");
|
||||
}
|
||||
if (empty($options['public_key'])) {
|
||||
throw new InvalidArgumentException("Missing Config -- [public_key]");
|
||||
}
|
||||
if (empty($options['private_key'])) {
|
||||
throw new InvalidArgumentException("Missing Config -- [private_key]");
|
||||
}
|
||||
if (!empty($options['debug'])) {
|
||||
$this->gateway = 'https://openapi.alipaydev.com/gateway.do?charset=utf-8';
|
||||
}
|
||||
$this->options = new DataArray([
|
||||
'app_id' => $this->config->get('appid'),
|
||||
'charset' => empty($options['charset']) ? 'utf-8' : $options['charset'],
|
||||
'format' => 'JSON',
|
||||
'version' => '1.0',
|
||||
'sign_type' => empty($options['sign_type']) ? 'RSA2' : $options['sign_type'],
|
||||
'timestamp' => date('Y-m-d H:i:s'),
|
||||
]);
|
||||
if (isset($options['notify_url']) && $options['notify_url'] !== '') {
|
||||
$this->options->set('notify_url', $options['notify_url']);
|
||||
}
|
||||
if (isset($options['return_url']) && $options['return_url'] !== '') {
|
||||
$this->options->set('return_url', $options['return_url']);
|
||||
}
|
||||
if (isset($options['app_auth_token']) && $options['app_auth_token'] !== '') {
|
||||
$this->options->set('app_auth_token', $options['app_auth_token']);
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* 静态创建对象
|
||||
* @param array $config
|
||||
* @return static
|
||||
*/
|
||||
public static function instance(array $config)
|
||||
{
|
||||
$key = md5(get_called_class() . serialize($config));
|
||||
if (isset(self::$cache[$key])) return self::$cache[$key];
|
||||
return self::$cache[$key] = new static($config);
|
||||
}
|
||||
|
||||
/**
|
||||
* 查询支付宝订单状态
|
||||
* @param string $out_trade_no
|
||||
* @return array|boolean
|
||||
* @throws InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function query($out_trade_no = '')
|
||||
{
|
||||
$this->options->set('method', 'alipay.trade.query');
|
||||
return $this->getResult(['out_trade_no' => $out_trade_no]);
|
||||
}
|
||||
|
||||
/**
|
||||
* 支付宝订单退款操作
|
||||
* @param array|string $options 退款参数或退款商户订单号
|
||||
* @param null $refund_amount 退款金额
|
||||
* @return array|boolean
|
||||
* @throws InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function refund($options, $refund_amount = null)
|
||||
{
|
||||
if (!is_array($options)) $options = ['out_trade_no' => $options, 'refund_amount' => $refund_amount];
|
||||
$this->options->set('method', 'alipay.trade.refund');
|
||||
return $this->getResult($options);
|
||||
}
|
||||
|
||||
/**
|
||||
* 关闭支付宝进行中的订单
|
||||
* @param array|string $options
|
||||
* @return array|boolean
|
||||
* @throws InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function close($options)
|
||||
{
|
||||
if (!is_array($options)) $options = ['out_trade_no' => $options];
|
||||
$this->options->set('method', 'alipay.trade.close');
|
||||
return $this->getResult($options);
|
||||
}
|
||||
|
||||
/**
|
||||
* 获取通知数据
|
||||
* @param boolean $needSignType 是否需要sign_type字段
|
||||
* @return boolean|array
|
||||
* @throws InvalidResponseException
|
||||
*/
|
||||
public function notify($needSignType = false)
|
||||
{
|
||||
$data = $_POST;
|
||||
if (empty($data) || empty($data['sign'])) {
|
||||
throw new InvalidResponseException('Illegal push request.', 0, $data);
|
||||
}
|
||||
$string = $this->getSignContent($data, $needSignType);
|
||||
$content = wordwrap($this->config->get('public_key'), 64, "\n", true);
|
||||
$res = "-----BEGIN PUBLIC KEY-----\n{$content}\n-----END PUBLIC KEY-----";
|
||||
if (openssl_verify($string, base64_decode($data['sign']), $res, OPENSSL_ALGO_SHA256) !== 1) {
|
||||
throw new InvalidResponseException('Data signature verification failed.', 0, $data);
|
||||
}
|
||||
return $data;
|
||||
}
|
||||
|
||||
/**
|
||||
* 验证接口返回的数据签名
|
||||
* @param array $data 通知数据
|
||||
* @param null|string $sign 数据签名
|
||||
* @return array|boolean
|
||||
* @throws InvalidResponseException
|
||||
*/
|
||||
protected function verify($data, $sign)
|
||||
{
|
||||
$content = wordwrap($this->config->get('public_key'), 64, "\n", true);
|
||||
$res = "-----BEGIN PUBLIC KEY-----\n{$content}\n-----END PUBLIC KEY-----";
|
||||
if ($this->options->get('sign_type') === 'RSA2') {
|
||||
if (openssl_verify(json_encode($data, 256), base64_decode($sign), $res, OPENSSL_ALGO_SHA256) !== 1) {
|
||||
throw new InvalidResponseException('Data signature verification failed.');
|
||||
}
|
||||
} else {
|
||||
if (openssl_verify(json_encode($data, 256), base64_decode($sign), $res, OPENSSL_ALGO_SHA1) !== 1) {
|
||||
throw new InvalidResponseException('Data signature verification failed.');
|
||||
}
|
||||
}
|
||||
return $data;
|
||||
}
|
||||
|
||||
/**
|
||||
* 获取数据签名
|
||||
* @return string
|
||||
*/
|
||||
protected function getSign()
|
||||
{
|
||||
$content = wordwrap($this->config->get('private_key'), 64, "\n", true);
|
||||
$string = "-----BEGIN RSA PRIVATE KEY-----\n{$content}\n-----END RSA PRIVATE KEY-----";
|
||||
if ($this->options->get('sign_type') === 'RSA2') {
|
||||
openssl_sign($this->getSignContent($this->options->get(), true), $sign, $string, OPENSSL_ALGO_SHA256);
|
||||
} else {
|
||||
openssl_sign($this->getSignContent($this->options->get(), true), $sign, $string, OPENSSL_ALGO_SHA1);
|
||||
}
|
||||
return base64_encode($sign);
|
||||
}
|
||||
|
||||
/**
|
||||
* 数据签名处理
|
||||
* @param array $data 需要进行签名数据
|
||||
* @param boolean $needSignType 是否需要sign_type字段
|
||||
* @return bool|string
|
||||
*/
|
||||
private function getSignContent(array $data, $needSignType = false)
|
||||
{
|
||||
list($attrs,) = [[], ksort($data)];
|
||||
if (isset($data['sign'])) unset($data['sign']);
|
||||
if (empty($needSignType)) unset($data['sign_type']);
|
||||
foreach ($data as $key => $value) {
|
||||
if ($value === '' || is_null($value)) continue;
|
||||
array_push($attrs, "{$key}={$value}");
|
||||
}
|
||||
return join('&', $attrs);
|
||||
}
|
||||
|
||||
/**
|
||||
* 数据包生成及数据签名
|
||||
* @param array $options
|
||||
*/
|
||||
protected function applyData($options)
|
||||
{
|
||||
$this->options->set('biz_content', json_encode($this->params->merge($options), 256));
|
||||
$this->options->set('sign', $this->getSign());
|
||||
}
|
||||
|
||||
/**
|
||||
* 请求接口并验证访问数据
|
||||
* @param array $options
|
||||
* @return array|boolean
|
||||
* @throws InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
protected function getResult($options)
|
||||
{
|
||||
$this->applyData($options);
|
||||
$method = str_replace('.', '_', $this->options['method']) . '_response';
|
||||
$data = json_decode(Tools::get($this->gateway, $this->options->get()), true);
|
||||
if (!isset($data[$method]['code']) || $data[$method]['code'] !== '10000') {
|
||||
throw new InvalidResponseException(
|
||||
"Error: " .
|
||||
(empty($data[$method]['code']) ? '' : "{$data[$method]['msg']} [{$data[$method]['code']}]\r\n") .
|
||||
(empty($data[$method]['sub_code']) ? '' : "{$data[$method]['sub_msg']} [{$data[$method]['sub_code']}]\r\n"),
|
||||
$data[$method]['code'], $data
|
||||
);
|
||||
}
|
||||
return $data[$method];
|
||||
// 去除返回结果签名检查
|
||||
// return $this->verify($data[$method], $data['sign']);
|
||||
}
|
||||
|
||||
/**
|
||||
* 生成支付HTML代码
|
||||
* @return string
|
||||
*/
|
||||
protected function buildPayHtml()
|
||||
{
|
||||
$html = "<form id='alipaysubmit' name='alipaysubmit' action='{$this->gateway}' method='post'>";
|
||||
foreach ($this->options->get() as $key => $value) {
|
||||
$value = str_replace("'", ''', $value);
|
||||
$html .= "<input type='hidden' name='{$key}' value='{$value}'/>";
|
||||
}
|
||||
$html .= "<input type='submit' value='ok' style='display:none;'></form>";
|
||||
return "{$html}<script>document.forms['alipaysubmit'].submit();</script>";
|
||||
}
|
||||
|
||||
/**
|
||||
* 应用数据操作
|
||||
* @param array $options
|
||||
* @return mixed
|
||||
*/
|
||||
abstract public function apply($options);
|
||||
|
||||
}
|
@ -43,18 +43,18 @@ class BasicPushEvent
|
||||
*/
|
||||
protected $encryptType;
|
||||
|
||||
/**
|
||||
* 公众号的推送请求参数
|
||||
* @var DataArray
|
||||
*/
|
||||
protected $input;
|
||||
|
||||
/**
|
||||
* 当前公众号配置对象
|
||||
* @var DataArray
|
||||
*/
|
||||
protected $config;
|
||||
|
||||
/**
|
||||
* 公众号的推送请求参数
|
||||
* @var DataArray
|
||||
*/
|
||||
protected $params;
|
||||
|
||||
/**
|
||||
* 公众号推送内容对象
|
||||
* @var DataArray
|
||||
@ -85,13 +85,13 @@ class BasicPushEvent
|
||||
}
|
||||
// 参数初始化
|
||||
$this->config = new DataArray($options);
|
||||
$this->params = new DataArray($_REQUEST);
|
||||
$this->input = new DataArray($_REQUEST);
|
||||
$this->appid = $this->config->get('appid');
|
||||
// 推送消息处理
|
||||
if ($_SERVER['REQUEST_METHOD'] == "POST") {
|
||||
$this->postxml = file_get_contents("php://input");
|
||||
$this->encryptType = $this->params->get('encrypt_type');
|
||||
if ($this->encryptType == 'aes') {
|
||||
$this->encryptType = $this->input->get('encrypt_type');
|
||||
if ($this->isEncrypt()) {
|
||||
if (empty($options['encodingaeskey'])) {
|
||||
throw new InvalidArgumentException("Missing Config -- [encodingaeskey]");
|
||||
}
|
||||
@ -109,23 +109,33 @@ class BasicPushEvent
|
||||
$this->receive = new DataArray(Tools::xml2arr($this->postxml));
|
||||
} elseif ($_SERVER['REQUEST_METHOD'] == "GET" && $this->checkSignature()) {
|
||||
@ob_clean();
|
||||
exit($this->params->get('echostr'));
|
||||
exit($this->input->get('echostr'));
|
||||
} else {
|
||||
throw new InvalidResponseException('Invalid interface request.', '0');
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* 消息是否需要加密
|
||||
* @return boolean
|
||||
*/
|
||||
public function isEncrypt()
|
||||
{
|
||||
return $this->encryptType === 'aes';
|
||||
}
|
||||
|
||||
/**
|
||||
* 回复消息
|
||||
* @param array $data 消息内容
|
||||
* @param bool $return 是否返回XML内容
|
||||
* @param boolean $return 是否返回XML内容
|
||||
* @param boolean $isEncrypt 是否加密内容
|
||||
* @return string
|
||||
* @throws InvalidDecryptException
|
||||
*/
|
||||
public function reply(array $data = [], $return = false)
|
||||
public function reply(array $data = [], $return = false, $isEncrypt = false)
|
||||
{
|
||||
$xml = Tools::arr2xml(empty($data) ? $this->message : $data);
|
||||
if ($this->encryptType == 'aes') {
|
||||
if ($this->isEncrypt() || $isEncrypt) {
|
||||
if (!class_exists('Prpcrypt', false)) {
|
||||
require __DIR__ . '/Prpcrypt.php';
|
||||
}
|
||||
@ -134,9 +144,7 @@ class BasicPushEvent
|
||||
$component_appid = $this->config->get('component_appid');
|
||||
$appid = empty($component_appid) ? $this->appid : $component_appid;
|
||||
$array = $prpcrypt->encrypt($xml, $appid);
|
||||
if ($array[0] > 0) {
|
||||
throw new InvalidDecryptException('Encrypt Error.', '0');
|
||||
}
|
||||
if ($array[0] > 0) throw new InvalidDecryptException('Encrypt Error.', '0');
|
||||
list($timestamp, $encrypt) = [time(), $array[1]];
|
||||
$nonce = rand(77, 999) * rand(605, 888) * rand(11, 99);
|
||||
$tmpArr = [$this->config->get('token'), $timestamp, $nonce, $encrypt];
|
||||
@ -145,9 +153,7 @@ class BasicPushEvent
|
||||
$format = "<xml><Encrypt><![CDATA[%s]]></Encrypt><MsgSignature><![CDATA[%s]]></MsgSignature><TimeStamp>%s</TimeStamp><Nonce><![CDATA[%s]]></Nonce></xml>";
|
||||
$xml = sprintf($format, $encrypt, $signature, $timestamp, $nonce);
|
||||
}
|
||||
if ($return) {
|
||||
return $xml;
|
||||
}
|
||||
if ($return) return $xml;
|
||||
@ob_clean();
|
||||
echo $xml;
|
||||
}
|
||||
@ -159,16 +165,13 @@ class BasicPushEvent
|
||||
*/
|
||||
private function checkSignature($str = '')
|
||||
{
|
||||
$nonce = $this->params->get('nonce');
|
||||
$timestamp = $this->params->get('timestamp');
|
||||
$msg_signature = $this->params->get('msg_signature');
|
||||
$signature = empty($msg_signature) ? $this->params->get('signature') : $msg_signature;
|
||||
$nonce = $this->input->get('nonce');
|
||||
$timestamp = $this->input->get('timestamp');
|
||||
$msg_signature = $this->input->get('msg_signature');
|
||||
$signature = empty($msg_signature) ? $this->input->get('signature') : $msg_signature;
|
||||
$tmpArr = [$this->config->get('token'), $timestamp, $nonce, $str];
|
||||
sort($tmpArr, SORT_STRING);
|
||||
if (sha1(implode($tmpArr)) == $signature) {
|
||||
return true;
|
||||
}
|
||||
return false;
|
||||
return sha1(implode($tmpArr)) === $signature;
|
||||
}
|
||||
|
||||
/**
|
||||
|
@ -40,19 +40,25 @@ class BasicWeChat
|
||||
* 当前请求方法参数
|
||||
* @var array
|
||||
*/
|
||||
private $currentMethod = [];
|
||||
protected $currentMethod = [];
|
||||
|
||||
/**
|
||||
* 当前模式
|
||||
* @var bool
|
||||
*/
|
||||
private $isTry = false;
|
||||
protected $isTry = false;
|
||||
|
||||
/**
|
||||
* 静态缓存
|
||||
* @var static
|
||||
*/
|
||||
protected static $cache;
|
||||
|
||||
/**
|
||||
* 注册代替函数
|
||||
* @var string
|
||||
*/
|
||||
private $GetAccessTokenCallback;
|
||||
protected $GetAccessTokenCallback;
|
||||
|
||||
/**
|
||||
* BasicWeChat constructor.
|
||||
@ -75,6 +81,18 @@ class BasicWeChat
|
||||
$this->config = new DataArray($options);
|
||||
}
|
||||
|
||||
/**
|
||||
* 静态创建对象
|
||||
* @param array $config
|
||||
* @return static
|
||||
*/
|
||||
public static function instance(array $config)
|
||||
{
|
||||
$key = md5(get_called_class() . serialize($config));
|
||||
if (isset(self::$cache[$key])) return self::$cache[$key];
|
||||
return self::$cache[$key] = new static($config);
|
||||
}
|
||||
|
||||
/**
|
||||
* 获取访问accessToken
|
||||
* @return string
|
||||
@ -105,11 +123,30 @@ class BasicWeChat
|
||||
if (!empty($result['access_token'])) {
|
||||
Tools::setCache($cache, $result['access_token'], 7000);
|
||||
}
|
||||
return $result['access_token'];
|
||||
return $this->access_token = $result['access_token'];
|
||||
}
|
||||
|
||||
/**
|
||||
* 清理删除accessToken
|
||||
* 设置外部接口 AccessToken
|
||||
* @param string $access_token
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
* @author 高一平 <iam@gaoyiping.com>
|
||||
*
|
||||
* 当用户使用自己的缓存驱动时,直接实例化对象后可直接设置 AccessToekn
|
||||
* - 多用于分布式项目时保持 AccessToken 统一
|
||||
* - 使用此方法后就由用户来保证传入的 AccessToekn 为有效 AccessToekn
|
||||
*/
|
||||
public function setAccessToken($access_token)
|
||||
{
|
||||
if (!is_string($access_token)) {
|
||||
throw new InvalidArgumentException("Invalid AccessToken type, need string.");
|
||||
}
|
||||
$cache = $this->config->get('appid') . '_access_token';
|
||||
Tools::setCache($cache, $this->access_token = $access_token);
|
||||
}
|
||||
|
||||
/**
|
||||
* 清理删除 AccessToken
|
||||
* @return bool
|
||||
*/
|
||||
public function delAccessToken()
|
||||
@ -122,18 +159,23 @@ class BasicWeChat
|
||||
* 以GET获取接口数据并转为数组
|
||||
* @param string $url 接口地址
|
||||
* @return array
|
||||
* @throws InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
protected function httpGetForJson($url)
|
||||
{
|
||||
try {
|
||||
return Tools::json2arr(Tools::get($url));
|
||||
} catch (InvalidResponseException $e) {
|
||||
if (!$this->isTry && in_array($e->getCode(), ['40014', '40001', '41001', '42001'])) {
|
||||
if (isset($this->currentMethod['method']) && empty($this->isTry)) {
|
||||
if (in_array($e->getCode(), ['40014', '40001', '41001', '42001'])) {
|
||||
$this->delAccessToken();
|
||||
$this->isTry = true;
|
||||
return call_user_func_array([$this, $this->currentMethod['method']], $this->currentMethod['arguments']);
|
||||
}
|
||||
}
|
||||
throw new InvalidResponseException($e->getMessage(), $e->getCode());
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
@ -142,6 +184,8 @@ class BasicWeChat
|
||||
* @param array $data 请求数据
|
||||
* @param bool $buildToJson
|
||||
* @return array
|
||||
* @throws InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
protected function httpPostForJson($url, array $data, $buildToJson = true)
|
||||
{
|
||||
@ -149,10 +193,10 @@ class BasicWeChat
|
||||
return Tools::json2arr(Tools::post($url, $buildToJson ? Tools::arr2json($data) : $data));
|
||||
} catch (InvalidResponseException $e) {
|
||||
if (!$this->isTry && in_array($e->getCode(), ['40014', '40001', '41001', '42001'])) {
|
||||
$this->delAccessToken();
|
||||
$this->isTry = true;
|
||||
[$this->delAccessToken(), $this->isTry = true];
|
||||
return call_user_func_array([$this, $this->currentMethod['method']], $this->currentMethod['arguments']);
|
||||
}
|
||||
throw new InvalidResponseException($e->getMessage(), $e->getCode());
|
||||
}
|
||||
}
|
||||
|
||||
|
203
WeChat/Contracts/BasicWePay.php
Normal file
203
WeChat/Contracts/BasicWePay.php
Normal file
@ -0,0 +1,203 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
namespace WeChat\Contracts;
|
||||
|
||||
use WeChat\Exceptions\InvalidArgumentException;
|
||||
use WeChat\Exceptions\InvalidResponseException;
|
||||
|
||||
/**
|
||||
* 微信支付基础类
|
||||
* Class BasicPay
|
||||
* @package WeChat\Contracts
|
||||
*/
|
||||
class BasicWePay
|
||||
{
|
||||
/**
|
||||
* 商户配置
|
||||
* @var DataArray
|
||||
*/
|
||||
protected $config;
|
||||
|
||||
/**
|
||||
* 当前请求数据
|
||||
* @var DataArray
|
||||
*/
|
||||
protected $params;
|
||||
|
||||
/**
|
||||
* 静态缓存
|
||||
* @var static
|
||||
*/
|
||||
protected static $cache;
|
||||
|
||||
/**
|
||||
* WeChat constructor.
|
||||
* @param array $options
|
||||
*/
|
||||
public function __construct(array $options)
|
||||
{
|
||||
if (empty($options['appid'])) {
|
||||
throw new InvalidArgumentException("Missing Config -- [appid]");
|
||||
}
|
||||
if (empty($options['mch_id'])) {
|
||||
throw new InvalidArgumentException("Missing Config -- [mch_id]");
|
||||
}
|
||||
if (empty($options['mch_key'])) {
|
||||
throw new InvalidArgumentException("Missing Config -- [mch_key]");
|
||||
}
|
||||
if (!empty($options['cache_path'])) {
|
||||
Tools::$cache_path = $options['cache_path'];
|
||||
}
|
||||
$this->config = new DataArray($options);
|
||||
// 商户基础参数
|
||||
$this->params = new DataArray([
|
||||
'appid' => $this->config->get('appid'),
|
||||
'mch_id' => $this->config->get('mch_id'),
|
||||
'nonce_str' => Tools::createNoncestr(),
|
||||
]);
|
||||
// 商户参数支持
|
||||
if ($this->config->get('sub_appid')) {
|
||||
$this->params->set('sub_appid', $this->config->get('sub_appid'));
|
||||
}
|
||||
if ($this->config->get('sub_mch_id')) {
|
||||
$this->params->set('sub_mch_id', $this->config->get('sub_mch_id'));
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* 静态创建对象
|
||||
* @param array $config
|
||||
* @return static
|
||||
*/
|
||||
public static function instance(array $config)
|
||||
{
|
||||
$key = md5(get_called_class() . serialize($config));
|
||||
if (isset(self::$cache[$key])) return self::$cache[$key];
|
||||
return self::$cache[$key] = new static($config);
|
||||
}
|
||||
|
||||
/**
|
||||
* 获取微信支付通知
|
||||
* @return array
|
||||
* @throws InvalidResponseException
|
||||
*/
|
||||
public function getNotify()
|
||||
{
|
||||
$data = Tools::xml2arr(file_get_contents('php://input'));
|
||||
if (isset($data['sign']) && $this->getPaySign($data) === $data['sign']) {
|
||||
return $data;
|
||||
}
|
||||
throw new InvalidResponseException('Invalid Notify.', '0');
|
||||
}
|
||||
|
||||
/**
|
||||
* 获取微信支付通知回复内容
|
||||
* @return string
|
||||
*/
|
||||
public function getNotifySuccessReply()
|
||||
{
|
||||
return Tools::arr2xml(['return_code' => 'SUCCESS', 'return_msg' => 'OK']);
|
||||
}
|
||||
|
||||
/**
|
||||
* 生成支付签名
|
||||
* @param array $data 参与签名的数据
|
||||
* @param string $signType 参与签名的类型
|
||||
* @param string $buff 参与签名字符串前缀
|
||||
* @return string
|
||||
*/
|
||||
public function getPaySign(array $data, $signType = 'MD5', $buff = '')
|
||||
{
|
||||
ksort($data);
|
||||
if (isset($data['sign'])) unset($data['sign']);
|
||||
foreach ($data as $k => $v) $buff .= "{$k}={$v}&";
|
||||
$buff .= ("key=" . $this->config->get('mch_key'));
|
||||
if (strtoupper($signType) === 'MD5') {
|
||||
return strtoupper(md5($buff));
|
||||
}
|
||||
return strtoupper(hash_hmac('SHA256', $buff, $this->config->get('mch_key')));
|
||||
}
|
||||
|
||||
/**
|
||||
* 转换短链接
|
||||
* @param string $longUrl 需要转换的URL,签名用原串,传输需URLencode
|
||||
* @return array
|
||||
* @throws InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function shortUrl($longUrl)
|
||||
{
|
||||
$url = 'https://api.mch.weixin.qq.com/tools/shorturl';
|
||||
return $this->callPostApi($url, ['long_url' => $longUrl]);
|
||||
}
|
||||
|
||||
|
||||
/**
|
||||
* 数组直接转xml数据输出
|
||||
* @param array $data
|
||||
* @param bool $isReturn
|
||||
* @return string
|
||||
*/
|
||||
public function toXml(array $data, $isReturn = false)
|
||||
{
|
||||
$xml = Tools::arr2xml($data);
|
||||
if ($isReturn) {
|
||||
return $xml;
|
||||
}
|
||||
echo $xml;
|
||||
}
|
||||
|
||||
/**
|
||||
* 以Post请求接口
|
||||
* @param string $url 请求
|
||||
* @param array $data 接口参数
|
||||
* @param bool $isCert 是否需要使用双向证书
|
||||
* @param string $signType 数据签名类型 MD5|SHA256
|
||||
* @param bool $needSignType 是否需要传签名类型参数
|
||||
* @return array
|
||||
* @throws InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
protected function callPostApi($url, array $data, $isCert = false, $signType = 'HMAC-SHA256', $needSignType = true)
|
||||
{
|
||||
$option = [];
|
||||
if ($isCert) {
|
||||
$option['ssl_p12'] = $this->config->get('ssl_p12');
|
||||
$option['ssl_cer'] = $this->config->get('ssl_cer');
|
||||
$option['ssl_key'] = $this->config->get('ssl_key');
|
||||
if (is_string($option['ssl_p12']) && file_exists($option['ssl_p12'])) {
|
||||
$content = file_get_contents($option['ssl_p12']);
|
||||
if (openssl_pkcs12_read($content, $certs, $this->config->get('mch_id'))) {
|
||||
$option['ssl_key'] = Tools::pushFile(md5($certs['pkey']) . '.pem', $certs['pkey']);
|
||||
$option['ssl_cer'] = Tools::pushFile(md5($certs['cert']) . '.pem', $certs['cert']);
|
||||
} else throw new InvalidArgumentException("P12 certificate does not match MCH_ID --- ssl_p12");
|
||||
}
|
||||
if (empty($option['ssl_cer']) || !file_exists($option['ssl_cer'])) {
|
||||
throw new InvalidArgumentException("Missing Config -- ssl_cer", '0');
|
||||
}
|
||||
if (empty($option['ssl_key']) || !file_exists($option['ssl_key'])) {
|
||||
throw new InvalidArgumentException("Missing Config -- ssl_key", '0');
|
||||
}
|
||||
}
|
||||
$params = $this->params->merge($data);
|
||||
$needSignType && ($params['sign_type'] = strtoupper($signType));
|
||||
$params['sign'] = $this->getPaySign($params, $signType);
|
||||
$result = Tools::xml2arr(Tools::post($url, Tools::arr2xml($params), $option));
|
||||
if ($result['return_code'] !== 'SUCCESS') {
|
||||
throw new InvalidResponseException($result['return_msg'], '0');
|
||||
}
|
||||
return $result;
|
||||
}
|
||||
}
|
63
WeChat/Contracts/MyCurlFile.php
Normal file
63
WeChat/Contracts/MyCurlFile.php
Normal file
@ -0,0 +1,63 @@
|
||||
<?php
|
||||
|
||||
namespace WeChat\Contracts;
|
||||
|
||||
/**
|
||||
* 自定义CURL文件类
|
||||
* Class MyCurlFile
|
||||
* @package WeChat\Contracts
|
||||
*/
|
||||
class MyCurlFile extends \stdClass
|
||||
{
|
||||
/**
|
||||
* 当前数据类型
|
||||
* @var string
|
||||
*/
|
||||
public $datatype = 'MY_CURL_FILE';
|
||||
|
||||
/**
|
||||
* MyCurlFile constructor.
|
||||
* @param string|array $filename
|
||||
* @param string $mimetype
|
||||
* @param string $postname
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function __construct($filename, $mimetype = '', $postname = '')
|
||||
{
|
||||
if (is_array($filename)) {
|
||||
foreach ($filename as $k => $v) $this->{$k} = $v;
|
||||
} else {
|
||||
$this->mimetype = $mimetype;
|
||||
$this->postname = $postname;
|
||||
$this->extension = pathinfo($filename, PATHINFO_EXTENSION);
|
||||
if (empty($this->extension)) $this->extension = 'tmp';
|
||||
if (empty($this->mimetype)) $this->mimetype = Tools::getExtMine($this->extension);
|
||||
if (empty($this->postname)) $this->postname = pathinfo($filename, PATHINFO_BASENAME);
|
||||
$this->content = base64_encode(file_get_contents($filename));
|
||||
$this->tempname = md5($this->content) . ".{$this->extension}";
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* 获取文件信息
|
||||
* @return \CURLFile|string
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function get()
|
||||
{
|
||||
$this->filename = Tools::pushFile($this->tempname, base64_decode($this->content));
|
||||
if (class_exists('CURLFile')) {
|
||||
return new \CURLFile($this->filename, $this->mimetype, $this->postname);
|
||||
}
|
||||
return "@{$this->tempname};filename={$this->postname};type={$this->mimetype}";
|
||||
}
|
||||
|
||||
/**
|
||||
* 类销毁处理
|
||||
*/
|
||||
public function __destruct()
|
||||
{
|
||||
// Tools::delCache($this->tempname);
|
||||
}
|
||||
|
||||
}
|
@ -31,6 +31,22 @@ class Tools
|
||||
*/
|
||||
public static $cache_path = null;
|
||||
|
||||
/**
|
||||
* 缓存写入操作
|
||||
* @var array
|
||||
*/
|
||||
public static $cache_callable = [
|
||||
'set' => null, // 写入缓存
|
||||
'get' => null, // 获取缓存
|
||||
'del' => null, // 删除缓存
|
||||
'put' => null, // 写入文件
|
||||
];
|
||||
|
||||
/**
|
||||
* 网络缓存
|
||||
* @var array
|
||||
*/
|
||||
private static $cache_curl = [];
|
||||
|
||||
/**
|
||||
* 产生随机字符串
|
||||
@ -49,8 +65,8 @@ class Tools
|
||||
|
||||
|
||||
/**
|
||||
* 根据文件后缀获取文件MINE
|
||||
* @param array $ext 文件后缀
|
||||
* 根据文件后缀获取文件类型
|
||||
* @param string|array $ext 文件后缀
|
||||
* @param array $mine 文件后缀MINE信息
|
||||
* @return string
|
||||
* @throws LocalCacheException
|
||||
@ -65,7 +81,7 @@ class Tools
|
||||
}
|
||||
|
||||
/**
|
||||
* 获取所有文件扩展的mine
|
||||
* 获取所有文件扩展的类型
|
||||
* @return array
|
||||
* @throws LocalCacheException
|
||||
*/
|
||||
@ -75,11 +91,7 @@ class Tools
|
||||
if (empty($mines)) {
|
||||
$content = file_get_contents('http://svn.apache.org/repos/asf/httpd/httpd/trunk/docs/conf/mime.types');
|
||||
preg_match_all('#^([^\s]{2,}?)\s+(.+?)$#ism', $content, $matches, PREG_SET_ORDER);
|
||||
foreach ($matches as $match) {
|
||||
foreach (explode(" ", $match[2]) as $ext) {
|
||||
$mines[$ext] = $match[1];
|
||||
}
|
||||
}
|
||||
foreach ($matches as $match) foreach (explode(" ", $match[2]) as $ext) $mines[$ext] = $match[1];
|
||||
self::setCache('all_ext_mine', $mines);
|
||||
}
|
||||
return $mines;
|
||||
@ -95,13 +107,16 @@ class Tools
|
||||
*/
|
||||
public static function createCurlFile($filename, $mimetype = null, $postname = null)
|
||||
{
|
||||
is_null($postname) && $postname = basename($filename);
|
||||
is_null($mimetype) && $mimetype = self::getExtMine(pathinfo($filename, 4));
|
||||
if (is_string($filename) && file_exists($filename)) {
|
||||
if (is_null($postname)) $postname = basename($filename);
|
||||
if (is_null($mimetype)) $mimetype = self::getExtMine(pathinfo($filename, 4));
|
||||
if (function_exists('curl_file_create')) {
|
||||
return curl_file_create($filename, $mimetype, $postname);
|
||||
}
|
||||
return "@{$filename};filename={$postname};type={$mimetype}";
|
||||
}
|
||||
return $filename;
|
||||
}
|
||||
|
||||
/**
|
||||
* 数组转XML内容
|
||||
@ -143,7 +158,10 @@ class Tools
|
||||
*/
|
||||
public static function xml2arr($xml)
|
||||
{
|
||||
return json_decode(self::arr2json(simplexml_load_string($xml, 'SimpleXMLElement', LIBXML_NOCDATA)), true);
|
||||
$entity = libxml_disable_entity_loader(true);
|
||||
$data = (array)simplexml_load_string($xml, 'SimpleXMLElement', LIBXML_NOCDATA);
|
||||
libxml_disable_entity_loader($entity);
|
||||
return json_decode(json_encode($data), true);
|
||||
}
|
||||
|
||||
/**
|
||||
@ -153,9 +171,70 @@ class Tools
|
||||
*/
|
||||
public static function arr2json($data)
|
||||
{
|
||||
return preg_replace_callback('/\\\\u([0-9a-f]{4})/i', function ($matches) {
|
||||
return mb_convert_encoding(pack("H*", $matches[1]), "UTF-8", "UCS-2BE");
|
||||
}, json_encode($data));
|
||||
$json = json_encode(self::buildEnEmojiData($data), JSON_UNESCAPED_UNICODE);
|
||||
return $json === '[]' ? '{}' : $json;
|
||||
}
|
||||
|
||||
/**
|
||||
* 数组对象Emoji编译处理
|
||||
* @param array $data
|
||||
* @return array
|
||||
*/
|
||||
public static function buildEnEmojiData(array $data)
|
||||
{
|
||||
foreach ($data as $key => $value) {
|
||||
if (is_array($value)) {
|
||||
$data[$key] = self::buildEnEmojiData($value);
|
||||
} elseif (is_string($value)) {
|
||||
$data[$key] = self::emojiEncode($value);
|
||||
} else {
|
||||
$data[$key] = $value;
|
||||
}
|
||||
}
|
||||
return $data;
|
||||
}
|
||||
|
||||
/**
|
||||
* 数组对象Emoji反解析处理
|
||||
* @param array $data
|
||||
* @return array
|
||||
*/
|
||||
public static function buildDeEmojiData(array $data)
|
||||
{
|
||||
foreach ($data as $key => $value) {
|
||||
if (is_array($value)) {
|
||||
$data[$key] = self::buildDeEmojiData($value);
|
||||
} elseif (is_string($value)) {
|
||||
$data[$key] = self::emojiDecode($value);
|
||||
} else {
|
||||
$data[$key] = $value;
|
||||
}
|
||||
}
|
||||
return $data;
|
||||
}
|
||||
|
||||
/**
|
||||
* Emoji原形转换为String
|
||||
* @param string $content
|
||||
* @return string
|
||||
*/
|
||||
public static function emojiEncode($content)
|
||||
{
|
||||
return json_decode(preg_replace_callback("/(\\\u[ed][0-9a-f]{3})/i", function ($string) {
|
||||
return addslashes($string[0]);
|
||||
}, json_encode($content)));
|
||||
}
|
||||
|
||||
/**
|
||||
* Emoji字符串转换为原形
|
||||
* @param string $content
|
||||
* @return string
|
||||
*/
|
||||
public static function emojiDecode($content)
|
||||
{
|
||||
return json_decode(preg_replace_callback('/\\\\\\\\/i', function () {
|
||||
return '\\';
|
||||
}, json_encode($content)));
|
||||
}
|
||||
|
||||
/**
|
||||
@ -181,7 +260,8 @@ class Tools
|
||||
* @param string $url 访问URL
|
||||
* @param array $query GET数
|
||||
* @param array $options
|
||||
* @return bool|string
|
||||
* @return boolean|string
|
||||
* @throws LocalCacheException
|
||||
*/
|
||||
public static function get($url, $query = [], $options = [])
|
||||
{
|
||||
@ -194,7 +274,8 @@ class Tools
|
||||
* @param string $url 访问URL
|
||||
* @param array $data POST数据
|
||||
* @param array $options
|
||||
* @return bool|string
|
||||
* @return boolean|string
|
||||
* @throws LocalCacheException
|
||||
*/
|
||||
public static function post($url, $data = [], $options = [])
|
||||
{
|
||||
@ -207,9 +288,10 @@ class Tools
|
||||
* @param string $method 请求方法
|
||||
* @param string $url 请求方法
|
||||
* @param array $options 请求参数[headers,data,ssl_cer,ssl_key]
|
||||
* @return bool|string
|
||||
* @return boolean|string
|
||||
* @throws LocalCacheException
|
||||
*/
|
||||
protected static function doRequest($method, $url, $options = [])
|
||||
public static function doRequest($method, $url, $options = [])
|
||||
{
|
||||
$curl = curl_init();
|
||||
// GET参数设置
|
||||
@ -223,34 +305,76 @@ class Tools
|
||||
// POST数据设置
|
||||
if (strtolower($method) === 'post') {
|
||||
curl_setopt($curl, CURLOPT_POST, true);
|
||||
curl_setopt($curl, CURLOPT_POSTFIELDS, $options['data']);
|
||||
curl_setopt($curl, CURLOPT_POSTFIELDS, self::_buildHttpData($options['data']));
|
||||
}
|
||||
// 证书文件设置
|
||||
if (!empty($options['ssl_cer'])) {
|
||||
if (file_exists($options['ssl_cer'])) {
|
||||
if (!empty($options['ssl_cer'])) if (file_exists($options['ssl_cer'])) {
|
||||
curl_setopt($curl, CURLOPT_SSLCERTTYPE, 'PEM');
|
||||
curl_setopt($curl, CURLOPT_SSLCERT, $options['ssl_cer']);
|
||||
} else {
|
||||
throw new InvalidArgumentException("Certificate files that do not exist. --- [ssl_cer]");
|
||||
}
|
||||
}
|
||||
} else throw new InvalidArgumentException("Certificate files that do not exist. --- [ssl_cer]");
|
||||
// 证书文件设置
|
||||
if (!empty($options['ssl_key'])) {
|
||||
if (file_exists($options['ssl_key'])) {
|
||||
if (!empty($options['ssl_key'])) if (file_exists($options['ssl_key'])) {
|
||||
curl_setopt($curl, CURLOPT_SSLKEYTYPE, 'PEM');
|
||||
curl_setopt($curl, CURLOPT_SSLKEY, $options['ssl_key']);
|
||||
} else {
|
||||
throw new InvalidArgumentException("Certificate files that do not exist. --- [ssl_key]");
|
||||
}
|
||||
}
|
||||
} else throw new InvalidArgumentException("Certificate files that do not exist. --- [ssl_key]");
|
||||
curl_setopt($curl, CURLOPT_URL, $url);
|
||||
curl_setopt($curl, CURLOPT_TIMEOUT, 60);
|
||||
curl_setopt($curl, CURLOPT_HEADER, false);
|
||||
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
|
||||
curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, false);
|
||||
curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, false);
|
||||
list($content, $status) = [curl_exec($curl), curl_getinfo($curl), curl_close($curl)];
|
||||
return (intval($status["http_code"]) === 200) ? $content : false;
|
||||
list($content) = [curl_exec($curl), curl_close($curl)];
|
||||
// 清理 CURL 缓存文件
|
||||
if (!empty(self::$cache_curl)) foreach (self::$cache_curl as $key => $file) {
|
||||
Tools::delCache($file);
|
||||
unset(self::$cache_curl[$key]);
|
||||
}
|
||||
return $content;
|
||||
}
|
||||
|
||||
/**
|
||||
* POST数据过滤处理
|
||||
* @param array $data 需要处理的数据
|
||||
* @param boolean $build 是否编译数据
|
||||
* @return array|string
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
private static function _buildHttpData($data, $build = true)
|
||||
{
|
||||
if (!is_array($data)) return $data;
|
||||
foreach ($data as $key => $value) if (is_object($value) && $value instanceof \CURLFile) {
|
||||
$build = false;
|
||||
} elseif (is_object($value) && isset($value->datatype) && $value->datatype === 'MY_CURL_FILE') {
|
||||
$build = false;
|
||||
$mycurl = new MyCurlFile((array)$value);
|
||||
$data[$key] = $mycurl->get();
|
||||
array_push(self::$cache_curl, $mycurl->tempname);
|
||||
} elseif (is_string($value) && class_exists('CURLFile', false) && stripos($value, '@') === 0) {
|
||||
if (($filename = realpath(trim($value, '@'))) && file_exists($filename)) {
|
||||
$build = false;
|
||||
$data[$key] = self::createCurlFile($filename);
|
||||
}
|
||||
}
|
||||
return $build ? http_build_query($data) : $data;
|
||||
}
|
||||
|
||||
/**
|
||||
* 写入文件
|
||||
* @param string $name 文件名称
|
||||
* @param string $content 文件内容
|
||||
* @return string
|
||||
* @throws LocalCacheException
|
||||
*/
|
||||
public static function pushFile($name, $content)
|
||||
{
|
||||
if (is_callable(self::$cache_callable['put'])) {
|
||||
return call_user_func_array(self::$cache_callable['put'], func_get_args());
|
||||
}
|
||||
$file = self::_getCacheName($name);
|
||||
if (!file_put_contents($file, $content)) {
|
||||
throw new LocalCacheException('local file write error.', '0');
|
||||
}
|
||||
return $file;
|
||||
}
|
||||
|
||||
/**
|
||||
@ -258,15 +382,20 @@ class Tools
|
||||
* @param string $name 缓存名称
|
||||
* @param string $value 缓存内容
|
||||
* @param int $expired 缓存时间(0表示永久缓存)
|
||||
* @return string
|
||||
* @throws LocalCacheException
|
||||
*/
|
||||
public static function setCache($name, $value = '', $expired = 3600)
|
||||
{
|
||||
$cache_file = self::getCacheName($name);
|
||||
$content = serialize(['name' => $name, 'value' => $value, 'expired' => time() + intval($expired)]);
|
||||
if (!file_put_contents($cache_file, $content)) {
|
||||
if (is_callable(self::$cache_callable['set'])) {
|
||||
return call_user_func_array(self::$cache_callable['set'], func_get_args());
|
||||
}
|
||||
$file = self::_getCacheName($name);
|
||||
$data = ['name' => $name, 'value' => $value, 'expired' => time() + intval($expired)];
|
||||
if (!file_put_contents($file, serialize($data))) {
|
||||
throw new LocalCacheException('local cache error.', '0');
|
||||
}
|
||||
return $file;
|
||||
}
|
||||
|
||||
/**
|
||||
@ -276,8 +405,11 @@ class Tools
|
||||
*/
|
||||
public static function getCache($name)
|
||||
{
|
||||
$cache_file = self::getCacheName($name);
|
||||
if (file_exists($cache_file) && ($content = file_get_contents($cache_file))) {
|
||||
if (is_callable(self::$cache_callable['get'])) {
|
||||
return call_user_func_array(self::$cache_callable['get'], func_get_args());
|
||||
}
|
||||
$file = self::_getCacheName($name);
|
||||
if (file_exists($file) && ($content = file_get_contents($file))) {
|
||||
$data = unserialize($content);
|
||||
if (isset($data['expired']) && (intval($data['expired']) === 0 || intval($data['expired']) >= time())) {
|
||||
return $data['value'];
|
||||
@ -290,12 +422,15 @@ class Tools
|
||||
/**
|
||||
* 移除缓存文件
|
||||
* @param string $name 缓存名称
|
||||
* @return bool
|
||||
* @return boolean
|
||||
*/
|
||||
public static function delCache($name)
|
||||
{
|
||||
$cache_file = self::getCacheName($name);
|
||||
return file_exists($cache_file) ? unlink($cache_file) : true;
|
||||
if (is_callable(self::$cache_callable['del'])) {
|
||||
return call_user_func_array(self::$cache_callable['del'], func_get_args());
|
||||
}
|
||||
$file = self::_getCacheName($name);
|
||||
return file_exists($file) ? unlink($file) : true;
|
||||
}
|
||||
|
||||
/**
|
||||
@ -303,10 +438,10 @@ class Tools
|
||||
* @param string $name
|
||||
* @return string
|
||||
*/
|
||||
private static function getCacheName($name)
|
||||
private static function _getCacheName($name)
|
||||
{
|
||||
if (empty(self::$cache_path)) {
|
||||
self::$cache_path = dirname(__DIR__) . DIRECTORY_SEPARATOR . 'Cache' . DIRECTORY_SEPARATOR;
|
||||
self::$cache_path = dirname(dirname(__DIR__)) . DIRECTORY_SEPARATOR . 'Cache' . DIRECTORY_SEPARATOR;
|
||||
}
|
||||
self::$cache_path = rtrim(self::$cache_path, '/\\') . DIRECTORY_SEPARATOR;
|
||||
file_exists(self::$cache_path) || mkdir(self::$cache_path, 0755, true);
|
||||
|
@ -28,14 +28,13 @@ class Custom extends BasicWeChat
|
||||
* 添加客服帐号
|
||||
* @param string $kf_account 客服账号
|
||||
* @param string $nickname 客服昵称
|
||||
* @param string $password 账号密码
|
||||
* @return array
|
||||
* @throws Exceptions\InvalidResponseException
|
||||
* @throws Exceptions\LocalCacheException
|
||||
*/
|
||||
public function addAccount($kf_account, $nickname, $password)
|
||||
public function addAccount($kf_account, $nickname)
|
||||
{
|
||||
$data = ['kf_account' => $kf_account, 'nickname' => $nickname, 'password' => $password];
|
||||
$data = ['kf_account' => $kf_account, 'nickname' => $nickname];
|
||||
$url = "https://api.weixin.qq.com/customservice/kfaccount/add?access_token=ACCESS_TOKEN";
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->httpPostForJson($url, $data);
|
||||
@ -45,14 +44,13 @@ class Custom extends BasicWeChat
|
||||
* 修改客服帐号
|
||||
* @param string $kf_account 客服账号
|
||||
* @param string $nickname 客服昵称
|
||||
* @param string $password 账号密码
|
||||
* @return array
|
||||
* @throws Exceptions\InvalidResponseException
|
||||
* @throws Exceptions\LocalCacheException
|
||||
*/
|
||||
public function updateAccount($kf_account, $nickname, $password)
|
||||
public function updateAccount($kf_account, $nickname)
|
||||
{
|
||||
$data = ['kf_account' => $kf_account, 'nickname' => $nickname, 'password' => $password];
|
||||
$data = ['kf_account' => $kf_account, 'nickname' => $nickname];
|
||||
$url = "https://api.weixin.qq.com/customservice/kfaccount/update?access_token=ACCESS_TOKEN";
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->httpPostForJson($url, $data);
|
||||
@ -61,15 +59,13 @@ class Custom extends BasicWeChat
|
||||
/**
|
||||
* 删除客服帐号
|
||||
* @param string $kf_account 客服账号
|
||||
* @param string $nickname 客服昵称
|
||||
* @param string $password 账号密码
|
||||
* @return array
|
||||
* @throws Exceptions\InvalidResponseException
|
||||
* @throws Exceptions\LocalCacheException
|
||||
*/
|
||||
public function deleteAccount($kf_account, $nickname, $password)
|
||||
public function deleteAccount($kf_account)
|
||||
{
|
||||
$data = ['kf_account' => $kf_account, 'nickname' => $nickname, 'password' => $password];
|
||||
$data = ['kf_account' => $kf_account];
|
||||
$url = "https://api.weixin.qq.com/customservice/kfaccount/del?access_token=ACCESS_TOKEN";
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->httpPostForJson($url, $data);
|
||||
@ -83,7 +79,7 @@ class Custom extends BasicWeChat
|
||||
* @throws Exceptions\InvalidResponseException
|
||||
* @throws Exceptions\LocalCacheException
|
||||
*/
|
||||
public function inviteworker($kf_account, $invite_wx)
|
||||
public function inviteWorker($kf_account, $invite_wx)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/customservice/kfaccount/inviteworker?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
|
@ -12,17 +12,29 @@
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
return [
|
||||
'token' => 'test',
|
||||
'appid' => 'wx60a43dd8161666d4',
|
||||
'appsecret' => '71308e96a204296c57d7cd4b21b883e8',
|
||||
'encodingaeskey' => 'BJIUzE0gqlWy0GxfPp4J1oPTBmOrNDIGPNav1YFH5Z5',
|
||||
// 配置商户支付参数
|
||||
'mch_id' => "1332187001",
|
||||
'mch_key' => '11bd3d66d85f322a1e803cb587d18c3f',
|
||||
// 配置商户支付双向证书目录
|
||||
'ssl_key' => '',
|
||||
'ssl_cer' => '',
|
||||
// 配置缓存目录,需要拥有写权限
|
||||
'cache_path' => '',
|
||||
];
|
||||
namespace WeChat\Exceptions;
|
||||
|
||||
/**
|
||||
* 加载类异常
|
||||
* Class InvalidInstanceException
|
||||
* @package WeChat\Exceptions
|
||||
*/
|
||||
class InvalidInstanceException extends \Exception
|
||||
{
|
||||
/**
|
||||
* @var array
|
||||
*/
|
||||
public $raw = [];
|
||||
|
||||
/**
|
||||
* InvalidResponseException constructor.
|
||||
* @param string $message
|
||||
* @param integer $code
|
||||
* @param array $raw
|
||||
*/
|
||||
public function __construct($message, $code = 0, $raw = [])
|
||||
{
|
||||
parent::__construct($message, intval($code));
|
||||
$this->raw = $raw;
|
||||
}
|
||||
}
|
@ -14,7 +14,6 @@
|
||||
|
||||
namespace WeChat;
|
||||
|
||||
|
||||
use WeChat\Contracts\BasicWeChat;
|
||||
|
||||
/**
|
||||
@ -38,5 +37,32 @@ class Limit extends BasicWeChat
|
||||
return $this->callPostApi($url, ['appid' => $this->config->get('appid')]);
|
||||
}
|
||||
|
||||
/**
|
||||
* 网络检测
|
||||
* @param string $action 执行的检测动作
|
||||
* @param string $operator 指定平台从某个运营商进行检测
|
||||
* @return array
|
||||
* @throws Exceptions\InvalidResponseException
|
||||
* @throws Exceptions\LocalCacheException
|
||||
*/
|
||||
public function ping($action = 'all', $operator = 'DEFAULT')
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/callback/check?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, ['action' => $action, 'check_operator' => $operator]);
|
||||
}
|
||||
|
||||
/**
|
||||
* 获取微信服务器IP地址
|
||||
* @return array
|
||||
* @throws Exceptions\InvalidResponseException
|
||||
* @throws Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getCallbackIp()
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/getcallbackip?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->httpGetForJson($url);
|
||||
}
|
||||
|
||||
}
|
@ -46,15 +46,24 @@ class Media extends BasicWeChat
|
||||
/**
|
||||
* 获取临时素材
|
||||
* @param string $media_id
|
||||
* @return bool|string
|
||||
* @param string $outType 返回处理函数
|
||||
* @return array|string
|
||||
* @throws Exceptions\LocalCacheException
|
||||
* @throws InvalidResponseException
|
||||
*/
|
||||
public function get($media_id)
|
||||
public function get($media_id, $outType = null)
|
||||
{
|
||||
$url = "https://api.weixin.qq.com/cgi-bin/media/get?access_token=ACCESS_TOKEN&media_id={$media_id}";
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return Tools::get($url);
|
||||
$result = Tools::get($url);
|
||||
if (is_array($json = json_decode($result, true))) {
|
||||
if (!$this->isTry && isset($json['errcode']) && in_array($json['errcode'], ['40014', '40001', '41001', '42001'])) {
|
||||
[$this->delAccessToken(), $this->isTry = true];
|
||||
return call_user_func_array([$this, $this->currentMethod['method']], $this->currentMethod['arguments']);
|
||||
}
|
||||
return Tools::json2arr($result);
|
||||
}
|
||||
return is_null($outType) ? $result : $outType($result);
|
||||
}
|
||||
|
||||
/**
|
||||
@ -124,15 +133,24 @@ class Media extends BasicWeChat
|
||||
/**
|
||||
* 获取永久素材
|
||||
* @param string $media_id
|
||||
* @return array
|
||||
* @param null|string $outType 输出类型
|
||||
* @return array|string
|
||||
* @throws Exceptions\LocalCacheException
|
||||
* @throws InvalidResponseException
|
||||
*/
|
||||
public function getMaterial($media_id)
|
||||
public function getMaterial($media_id, $outType = null)
|
||||
{
|
||||
$url = "https://api.weixin.qq.com/cgi-bin/material/get_material?access_token=ACCESS_TOKEN";
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->httpPostForJson($url, ['media_id' => $media_id]);
|
||||
$result = Tools::post($url, ['media_id' => $media_id]);
|
||||
if (is_array($json = json_decode($result, true))) {
|
||||
if (!$this->isTry && isset($json['errcode']) && in_array($json['errcode'], ['40014', '40001', '41001', '42001'])) {
|
||||
[$this->delAccessToken(), $this->isTry = true];
|
||||
return call_user_func_array([$this, $this->currentMethod['method']], $this->currentMethod['arguments']);
|
||||
}
|
||||
return Tools::json2arr($result);
|
||||
}
|
||||
return is_null($outType) ? $result : $outType($result);
|
||||
}
|
||||
|
||||
/**
|
||||
|
@ -59,9 +59,6 @@ class Menu extends BasicWeChat
|
||||
*/
|
||||
public function create(array $data)
|
||||
{
|
||||
try {
|
||||
} catch (\Exception $e) {
|
||||
}
|
||||
$url = "https://api.weixin.qq.com/cgi-bin/menu/create?access_token=ACCESS_TOKEN";
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->httpPostForJson($url, $data);
|
||||
|
@ -41,6 +41,8 @@ class Oauth extends BasicWeChat
|
||||
/**
|
||||
* 通过 code 获取 AccessToken 和 openid
|
||||
* @return bool|array
|
||||
* @throws Exceptions\InvalidResponseException
|
||||
* @throws Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getOauthAccessToken()
|
||||
{
|
||||
@ -55,6 +57,8 @@ class Oauth extends BasicWeChat
|
||||
* 刷新AccessToken并续期
|
||||
* @param string $refresh_token
|
||||
* @return bool|array
|
||||
* @throws Exceptions\InvalidResponseException
|
||||
* @throws Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getOauthRefreshToken($refresh_token)
|
||||
{
|
||||
@ -68,6 +72,8 @@ class Oauth extends BasicWeChat
|
||||
* @param string $access_token 网页授权接口调用凭证,注意:此access_token与基础支持的access_token不同
|
||||
* @param string $openid 用户的唯一标识
|
||||
* @return array
|
||||
* @throws Exceptions\InvalidResponseException
|
||||
* @throws Exceptions\LocalCacheException
|
||||
*/
|
||||
public function checkOauthAccessToken($access_token, $openid)
|
||||
{
|
||||
@ -81,6 +87,8 @@ class Oauth extends BasicWeChat
|
||||
* @param string $openid 用户的唯一标识
|
||||
* @param string $lang 返回国家地区语言版本,zh_CN 简体,zh_TW 繁体,en 英语
|
||||
* @return array
|
||||
* @throws Exceptions\InvalidResponseException
|
||||
* @throws Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getUserInfo($access_token, $openid, $lang = 'zh_CN')
|
||||
{
|
||||
|
229
WeChat/Pay.php
229
WeChat/Pay.php
@ -14,262 +14,219 @@
|
||||
|
||||
namespace WeChat;
|
||||
|
||||
use WeChat\Contracts\DataArray;
|
||||
use WeChat\Contracts\Tools;
|
||||
use WeChat\Exceptions\InvalidArgumentException;
|
||||
use WeChat\Contracts\BasicWePay;
|
||||
use WeChat\Exceptions\InvalidResponseException;
|
||||
use WePay\Bill;
|
||||
use WePay\Order;
|
||||
use WePay\Refund;
|
||||
use WePay\Transfers;
|
||||
use WePay\TransfersBank;
|
||||
|
||||
/**
|
||||
* 微信支付商户
|
||||
* Class Pay
|
||||
* @package WeChat\Contracts
|
||||
*/
|
||||
class Pay
|
||||
class Pay extends BasicWePay
|
||||
{
|
||||
|
||||
/**
|
||||
* 商户配置
|
||||
* @var DataArray
|
||||
*/
|
||||
protected $config;
|
||||
|
||||
/**
|
||||
* 当前请求数据
|
||||
* @var DataArray
|
||||
*/
|
||||
protected $params;
|
||||
|
||||
|
||||
/**
|
||||
* WeChat constructor.
|
||||
* @param array $options
|
||||
*/
|
||||
public function __construct(array $options)
|
||||
{
|
||||
if (empty($options['appid'])) {
|
||||
throw new InvalidArgumentException("Missing Config -- [appid]");
|
||||
}
|
||||
if (empty($options['mch_id'])) {
|
||||
throw new InvalidArgumentException("Missing Config -- [mch_id]");
|
||||
}
|
||||
if (empty($options['mch_key'])) {
|
||||
throw new InvalidArgumentException("Missing Config -- [mch_key]");
|
||||
}
|
||||
if (!empty($options['cache_path'])) {
|
||||
Tools::$cache_path = $options['cache_path'];
|
||||
}
|
||||
$this->config = new DataArray($options);
|
||||
$this->params = new DataArray([
|
||||
'appid' => $this->config->get('appid'),
|
||||
'mch_id' => $this->config->get('mch_id'),
|
||||
'nonce_str' => Tools::createNoncestr(),
|
||||
]);
|
||||
}
|
||||
|
||||
/**
|
||||
* 统一下单
|
||||
* @param array $options
|
||||
* @return array
|
||||
* @throws Exceptions\LocalCacheException
|
||||
* @throws InvalidResponseException
|
||||
*/
|
||||
public function createOrder(array $options)
|
||||
{
|
||||
$url = 'https://api.mch.weixin.qq.com/pay/unifiedorder';
|
||||
return $this->callPostApi($url, $options);
|
||||
return Order::instance($this->config->get())->create($options);
|
||||
}
|
||||
|
||||
/**
|
||||
* 刷卡支付
|
||||
* @param array $options
|
||||
* @return array
|
||||
* @throws Exceptions\LocalCacheException
|
||||
* @throws InvalidResponseException
|
||||
*/
|
||||
public function createMicropay($options)
|
||||
{
|
||||
return Order::instance($this->config->get())->micropay($options);
|
||||
}
|
||||
|
||||
/**
|
||||
* 创建JsApi及H5支付参数
|
||||
* @param string $prepay_id 统一下单预支付码
|
||||
* @return array
|
||||
*/
|
||||
public function createParamsForJsApi($prepay_id)
|
||||
{
|
||||
return Order::instance($this->config->get())->jsapiParams($prepay_id);
|
||||
}
|
||||
|
||||
/**
|
||||
* 获取APP支付参数
|
||||
* @param string $prepay_id 统一下单预支付码
|
||||
* @return array
|
||||
*/
|
||||
public function createParamsForApp($prepay_id)
|
||||
{
|
||||
return Order::instance($this->config->get())->appParams($prepay_id);
|
||||
}
|
||||
|
||||
/**
|
||||
* 获取支付规则二维码
|
||||
* @param string $product_id 商户定义的商品id 或者订单号
|
||||
* @return string
|
||||
*/
|
||||
public function createParamsForRuleQrc($product_id)
|
||||
{
|
||||
return Order::instance($this->config->get())->qrcParams($product_id);
|
||||
}
|
||||
|
||||
/**
|
||||
* 查询订单
|
||||
* @param array $options
|
||||
* @return array
|
||||
* @throws Exceptions\LocalCacheException
|
||||
* @throws InvalidResponseException
|
||||
*/
|
||||
public function queryOrder(array $options)
|
||||
{
|
||||
$url = 'https://api.mch.weixin.qq.com/pay/orderquery';
|
||||
return $this->callPostApi($url, $options);
|
||||
return Order::instance($this->config->get())->query($options);
|
||||
}
|
||||
|
||||
/**
|
||||
* 关闭订单
|
||||
* @param string $out_trade_no 商户订单号
|
||||
* @return array
|
||||
* @throws Exceptions\LocalCacheException
|
||||
* @throws InvalidResponseException
|
||||
*/
|
||||
public function closeOrder($out_trade_no)
|
||||
{
|
||||
$url = 'https://api.mch.weixin.qq.com/pay/closeorder';
|
||||
return $this->callPostApi($url, ['out_trade_no' => $out_trade_no]);
|
||||
return Order::instance($this->config->get())->close($out_trade_no);
|
||||
}
|
||||
|
||||
/**
|
||||
* 申请退款
|
||||
* @param array $options
|
||||
* @return array
|
||||
* @throws Exceptions\LocalCacheException
|
||||
* @throws InvalidResponseException
|
||||
*/
|
||||
public function createRefund(array $options)
|
||||
{
|
||||
$url = 'https://api.mch.weixin.qq.com/secapi/pay/refund';
|
||||
return $this->callPostApi($url, $options, true);
|
||||
return Refund::instance($this->config->get())->create($options);
|
||||
}
|
||||
|
||||
/**
|
||||
* 查询退款
|
||||
* @param array $options
|
||||
* @return array
|
||||
* @throws Exceptions\LocalCacheException
|
||||
* @throws InvalidResponseException
|
||||
*/
|
||||
public function queryRefund(array $options)
|
||||
{
|
||||
$url = 'https://api.mch.weixin.qq.com/pay/refundquery';
|
||||
return $this->callPostApi($url, $options);
|
||||
return Refund::instance($this->config->get())->query($options);
|
||||
}
|
||||
|
||||
/**
|
||||
* 交易保障
|
||||
* @param array $options
|
||||
* @return array
|
||||
* @throws Exceptions\LocalCacheException
|
||||
* @throws InvalidResponseException
|
||||
*/
|
||||
public function report(array $options)
|
||||
{
|
||||
$url = 'https://api.mch.weixin.qq.com/payitil/report';
|
||||
return $this->callPostApi($url, $options);
|
||||
return Order::instance($this->config->get())->report($options);
|
||||
}
|
||||
|
||||
/**
|
||||
* 授权码查询openid
|
||||
* @param string $authCode 扫码支付授权码,设备读取用户微信中的条码或者二维码信息
|
||||
* @return array
|
||||
* @throws Exceptions\LocalCacheException
|
||||
* @throws InvalidResponseException
|
||||
*/
|
||||
public function queryAuthCode($authCode)
|
||||
{
|
||||
$url = 'https://api.mch.weixin.qq.com/tools/authcodetoopenid';
|
||||
return $this->callPostApi($url, ['auth_code' => $authCode]);
|
||||
}
|
||||
|
||||
/**
|
||||
* 转换短链接
|
||||
* @param string $longUrl 需要转换的URL,签名用原串,传输需URLencode
|
||||
* @return array
|
||||
* @throws InvalidResponseException
|
||||
*/
|
||||
public function shortUrl($longUrl)
|
||||
{
|
||||
$url = 'https://api.mch.weixin.qq.com/tools/shorturl';
|
||||
return $this->callPostApi($url, ['long_url' => $longUrl]);
|
||||
return Order::instance($this->config->get())->queryAuthCode($authCode);
|
||||
}
|
||||
|
||||
/**
|
||||
* 下载对账单
|
||||
* @param array $options
|
||||
* @return array
|
||||
* @param array $options 静音参数
|
||||
* @param null|string $outType 输出类型
|
||||
* @return bool|string
|
||||
* @throws Exceptions\LocalCacheException
|
||||
* @throws InvalidResponseException
|
||||
*/
|
||||
public function billDownload(array $options)
|
||||
public function billDownload(array $options, $outType = null)
|
||||
{
|
||||
$url = 'https://api.mch.weixin.qq.com/pay/downloadbill';
|
||||
return $this->callPostApi($url, $options);
|
||||
return Bill::instance($this->config->get())->download($options, $outType);
|
||||
}
|
||||
|
||||
|
||||
/**
|
||||
* 拉取订单评价数据
|
||||
* @param array $options
|
||||
* @return array
|
||||
* @throws Exceptions\LocalCacheException
|
||||
* @throws InvalidResponseException
|
||||
*/
|
||||
public function billCommtent(array $options)
|
||||
{
|
||||
$url = 'https://api.mch.weixin.qq.com/billcommentsp/batchquerycomment';
|
||||
return $this->callPostApi($url, $options, true);
|
||||
return Bill::instance($this->config->get())->comment($options);
|
||||
}
|
||||
|
||||
/**
|
||||
* 企业付款到零钱
|
||||
* @param array $options
|
||||
* @return array
|
||||
* @throws Exceptions\LocalCacheException
|
||||
* @throws InvalidResponseException
|
||||
*/
|
||||
public function createTransfers(array $options)
|
||||
{
|
||||
$url = 'https://api.mch.weixin.qq.com/mmpaymkttransfers/promotion/transfers';
|
||||
return $this->callPostApi($url, $options, true);
|
||||
return Transfers::instance($this->config->get())->create($options);
|
||||
}
|
||||
|
||||
/**
|
||||
* 查询企业付款到零钱
|
||||
* @param string $partner_trade_no 商户调用企业付款API时使用的商户订单号
|
||||
* @return array
|
||||
* @throws Exceptions\LocalCacheException
|
||||
* @throws InvalidResponseException
|
||||
*/
|
||||
public function queryTransfers($partner_trade_no)
|
||||
{
|
||||
$url = 'https://api.mch.weixin.qq.com/mmpaymkttransfers/gettransferinfo';
|
||||
return $this->callPostApi($url, ['partner_trade_no' => $partner_trade_no], true);
|
||||
return Transfers::instance($this->config->get())->query($partner_trade_no);
|
||||
}
|
||||
|
||||
/**
|
||||
* 获取微信支付通知
|
||||
* 企业付款到银行卡
|
||||
* @param array $options
|
||||
* @return array
|
||||
* @throws Exceptions\LocalCacheException
|
||||
* @throws Exceptions\InvalidDecryptException
|
||||
* @throws Exceptions\InvalidResponseException
|
||||
*/
|
||||
public function createTransfersBank(array $options)
|
||||
{
|
||||
return TransfersBank::instance($this->config->get())->create($options);
|
||||
}
|
||||
|
||||
/**
|
||||
* 商户企业付款到银行卡操作进行结果查询
|
||||
* @param string $partner_trade_no 商户订单号,需保持唯一
|
||||
* @return array
|
||||
* @throws Exceptions\LocalCacheException
|
||||
* @throws InvalidResponseException
|
||||
*/
|
||||
public function getNotify()
|
||||
public function queryTransFresBank($partner_trade_no)
|
||||
{
|
||||
$data = Tools::xml2arr(file_get_contents('php://input'));
|
||||
if (!empty($data['sign'])) {
|
||||
if ($this->getPaySign($data) === $data['sign']) {
|
||||
return $data;
|
||||
}
|
||||
}
|
||||
throw new InvalidResponseException('Invalid Notify.', '0');
|
||||
}
|
||||
|
||||
/**
|
||||
* 生成支付签名
|
||||
* @param array $data
|
||||
* @return string
|
||||
*/
|
||||
public function getPaySign(array $data)
|
||||
{
|
||||
unset($data['sign']);
|
||||
ksort($data);
|
||||
list($key, $str) = [$this->config->get('mch_key'), ''];
|
||||
foreach ($data as $k => $v) {
|
||||
$str .= "{$k}={$v}&";
|
||||
}
|
||||
return strtoupper(hash_hmac('SHA256', "{$str}key={$key}", $key));
|
||||
}
|
||||
|
||||
/**
|
||||
* 以Post请求接口
|
||||
* @param string $url 请求
|
||||
* @param array $data 接口参数
|
||||
* @param bool $isCert 是否需要使用双向证书
|
||||
* @return array
|
||||
* @throws InvalidResponseException
|
||||
*/
|
||||
public function callPostApi($url, array $data, $isCert = false)
|
||||
{
|
||||
$option = [];
|
||||
if ($isCert) {
|
||||
foreach (['ssl_cer', 'ssl_key'] as $key) {
|
||||
if (empty($options[$key])) {
|
||||
throw new InvalidArgumentException("Missing Config -- [{$key}]", '0');
|
||||
}
|
||||
}
|
||||
$option['ssl_cer'] = $this->config->get('ssl_cer');
|
||||
$option['ssl_key'] = $this->config->get('ssl_key');
|
||||
}
|
||||
$params = $this->params->merge($data);
|
||||
$params['sign_type'] = 'HMAC-SHA256';
|
||||
$params['sign'] = $this->getPaySign($params);
|
||||
$result = Tools::xml2arr(Tools::post($url, Tools::arr2xml($params), $option));
|
||||
if ($result['return_code'] !== 'SUCCESS') {
|
||||
throw new InvalidResponseException($result['return_msg'], '0');
|
||||
}
|
||||
return $result;
|
||||
return TransfersBank::instance($this->config->get())->query($partner_trade_no);
|
||||
}
|
||||
}
|
@ -14,7 +14,6 @@
|
||||
|
||||
namespace WeChat;
|
||||
|
||||
|
||||
use WeChat\Contracts\BasicWeChat;
|
||||
|
||||
/**
|
||||
|
@ -14,8 +14,8 @@
|
||||
|
||||
namespace WeChat;
|
||||
|
||||
use WeChat\Contracts\Tools;
|
||||
use WeChat\Contracts\BasicWeChat;
|
||||
use WeChat\Contracts\Tools;
|
||||
use WeChat\Exceptions\InvalidResponseException;
|
||||
|
||||
/**
|
||||
@ -87,15 +87,11 @@ class Script extends BasicWeChat
|
||||
"timestamp" => $data['timestamp'],
|
||||
"signature" => $this->getSignature($data, 'sha1'),
|
||||
'jsApiList' => [
|
||||
'onWXDeviceBluetoothStateChange', 'onWXDeviceStateChange',
|
||||
'openProductSpecificView', 'addCard', 'chooseCard', 'openCard',
|
||||
'translateVoice', 'getNetworkType', 'openLocation', 'getLocation',
|
||||
'onMenuShareTimeline', 'onMenuShareAppMessage', 'onMenuShareQQ', 'onMenuShareWeibo', 'onMenuShareQZone',
|
||||
'chooseImage', 'previewImage', 'uploadImage', 'downloadImage', 'closeWindow', 'scanQRCode', 'chooseWXPay',
|
||||
'hideOptionMenu', 'showOptionMenu', 'hideMenuItems', 'showMenuItems', 'hideAllNonBaseMenuItem', 'showAllNonBaseMenuItem',
|
||||
'startScanWXDevice', 'stopScanWXDevice', 'onWXDeviceBindStateChange', 'onScanWXDeviceResult', 'onReceiveDataFromWXDevice',
|
||||
'updateAppMessageShareData', 'updateTimelineShareData', 'onMenuShareTimeline', 'onMenuShareAppMessage', 'onMenuShareQQ', 'onMenuShareWeibo', 'onMenuShareQZone',
|
||||
'startRecord', 'stopRecord', 'onVoiceRecordEnd', 'playVoice', 'pauseVoice', 'stopVoice', 'onVoicePlayEnd', 'uploadVoice', 'downloadVoice',
|
||||
'openWXDeviceLib', 'closeWXDeviceLib', 'getWXDeviceInfos', 'sendDataToWXDevice', 'disconnectWXDevice', 'getWXDeviceTicket', 'connectWXDevice',
|
||||
'chooseImage', 'previewImage', 'uploadImage', 'downloadImage', 'translateVoice', 'getNetworkType', 'openLocation', 'getLocation',
|
||||
'hideOptionMenu', 'showOptionMenu', 'hideMenuItems', 'showMenuItems', 'hideAllNonBaseMenuItem', 'showAllNonBaseMenuItem',
|
||||
'closeWindow', 'scanQRCode', 'chooseWXPay', 'openProductSpecificView', 'addCard', 'chooseCard', 'openCard',
|
||||
],
|
||||
];
|
||||
}
|
||||
@ -104,18 +100,14 @@ class Script extends BasicWeChat
|
||||
* 数据生成签名
|
||||
* @param array $data 签名数组
|
||||
* @param string $method 签名方法
|
||||
* @param array $params 签名参数
|
||||
* @return bool|string 签名值
|
||||
*/
|
||||
protected function getSignature($data, $method = "sha1")
|
||||
protected function getSignature($data, $method = "sha1", $params = [])
|
||||
{
|
||||
if (!function_exists($method)) {
|
||||
return false;
|
||||
}
|
||||
ksort($data);
|
||||
$params = [];
|
||||
foreach ($data as $key => $value) {
|
||||
$params[] = "{$key}={$value}";
|
||||
}
|
||||
if (!function_exists($method)) return false;
|
||||
foreach ($data as $k => $v) array_push($params, "{$k}={$v}");
|
||||
return $method(join('&', $params));
|
||||
}
|
||||
}
|
@ -90,7 +90,7 @@ class Tags extends BasicWeChat
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/tags/members/batchtagging?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->httpPostForJson($url, ['openid_list' => $openids, $tagId]);
|
||||
return $this->httpPostForJson($url, ['openid_list' => $openids, 'tagid' => $tagId]);
|
||||
}
|
||||
|
||||
/**
|
||||
@ -105,7 +105,7 @@ class Tags extends BasicWeChat
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/tags/members/batchuntagging?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->httpPostForJson($url, ['openid_list' => $openids, $tagId]);
|
||||
return $this->httpPostForJson($url, ['openid_list' => $openids, 'tagid' => $tagId]);
|
||||
}
|
||||
|
||||
/**
|
||||
|
@ -49,7 +49,7 @@ class User extends BasicWeChat
|
||||
*/
|
||||
public function getUserInfo($openid, $lang = 'zh_CN')
|
||||
{
|
||||
$url = "https://api.weixin.qq.com/cgi-bin/user/info?access_token=ACCESS_TOKEN&openid={$openid}&lang=zh_CN";
|
||||
$url = "https://api.weixin.qq.com/cgi-bin/user/info?access_token=ACCESS_TOKEN&openid={$openid}&lang={$lang}";
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->httpGetForJson($url);
|
||||
}
|
||||
@ -87,6 +87,21 @@ class User extends BasicWeChat
|
||||
return $this->httpGetForJson($url);
|
||||
}
|
||||
|
||||
/**
|
||||
* 获取标签下粉丝列表
|
||||
* @param integer $tagid 标签ID
|
||||
* @param string $next_openid 第一个拉取的OPENID
|
||||
* @return array
|
||||
* @throws Exceptions\InvalidResponseException
|
||||
* @throws Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getUserListByTag($tagid, $next_openid = '')
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/user/tag/get?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->httpPostForJson($url, ['tagid' => $tagid, 'next_openid' => $next_openid]);
|
||||
}
|
||||
|
||||
/**
|
||||
* 获取公众号的黑名单列表
|
||||
* @param string $begin_openid
|
||||
|
@ -14,7 +14,6 @@
|
||||
|
||||
namespace WeChat;
|
||||
|
||||
|
||||
use WeChat\Contracts\BasicWeChat;
|
||||
|
||||
/**
|
||||
|
105
WeMini/Crypt.php
Normal file
105
WeMini/Crypt.php
Normal file
@ -0,0 +1,105 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
namespace WeMini;
|
||||
|
||||
use WeChat\Contracts\BasicWeChat;
|
||||
use WeChat\Contracts\Tools;
|
||||
use WeChat\Exceptions\InvalidDecryptException;
|
||||
use WeChat\Exceptions\InvalidResponseException;
|
||||
|
||||
|
||||
/**
|
||||
* 数据加密处理
|
||||
* Class Crypt
|
||||
* @package WeMini
|
||||
*/
|
||||
class Crypt extends BasicWeChat
|
||||
{
|
||||
|
||||
/**
|
||||
* 数据签名校验
|
||||
* @param string $iv
|
||||
* @param string $sessionKey
|
||||
* @param string $encryptedData
|
||||
* @return bool
|
||||
*/
|
||||
public function decode($iv, $sessionKey, $encryptedData)
|
||||
{
|
||||
require_once __DIR__ . DIRECTORY_SEPARATOR . 'crypt' . DIRECTORY_SEPARATOR . 'wxBizDataCrypt.php';
|
||||
$pc = new \WXBizDataCrypt($this->config->get('appid'), $sessionKey);
|
||||
$errCode = $pc->decryptData($encryptedData, $iv, $data);
|
||||
if ($errCode == 0) {
|
||||
return json_decode($data, true);
|
||||
}
|
||||
return false;
|
||||
}
|
||||
|
||||
/**
|
||||
* 登录凭证校验
|
||||
* @param string $code 登录时获取的 code
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function session($code)
|
||||
{
|
||||
$appid = $this->config->get('appid');
|
||||
$secret = $this->config->get('appsecret');
|
||||
$url = "https://api.weixin.qq.com/sns/jscode2session?appid={$appid}&secret={$secret}&js_code={$code}&grant_type=authorization_code";
|
||||
return json_decode(Tools::get($url), true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 换取用户信息
|
||||
* @param string $code 用户登录凭证(有效期五分钟)
|
||||
* @param string $iv 加密算法的初始向量
|
||||
* @param string $encryptedData 加密数据( encryptedData )
|
||||
* @return array
|
||||
* @throws InvalidDecryptException
|
||||
* @throws InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function userInfo($code, $iv, $encryptedData)
|
||||
{
|
||||
$result = $this->session($code);
|
||||
if (empty($result['session_key'])) {
|
||||
throw new InvalidResponseException('Code 换取 SessionKey 失败', 403);
|
||||
}
|
||||
$userinfo = $this->decode($iv, $result['session_key'], $encryptedData);
|
||||
if (empty($userinfo)) {
|
||||
throw new InvalidDecryptException('用户信息解析失败', 403);
|
||||
}
|
||||
return array_merge($result, $userinfo);
|
||||
}
|
||||
|
||||
/**
|
||||
* 用户支付完成后,获取该用户的 UnionId
|
||||
* @param string $openid 支付用户唯一标识
|
||||
* @param null|string $transaction_id 微信支付订单号
|
||||
* @param null|string $mch_id 微信支付分配的商户号,和商户订单号配合使用
|
||||
* @param null|string $out_trade_no 微信支付商户订单号,和商户号配合使用
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getPaidUnionId($openid, $transaction_id = null, $mch_id = null, $out_trade_no = null)
|
||||
{
|
||||
$url = "https://api.weixin.qq.com/wxa/getpaidunionid?access_token=ACCESS_TOKEN&openid={$openid}";
|
||||
if (is_null($mch_id)) $url .= "&mch_id={$mch_id}";
|
||||
if (is_null($out_trade_no)) $url .= "&out_trade_no={$out_trade_no}";
|
||||
if (is_null($transaction_id)) $url .= "&transaction_id={$transaction_id}";
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callGetApi($url);
|
||||
}
|
||||
}
|
181
WeMini/Delivery.php
Normal file
181
WeMini/Delivery.php
Normal file
@ -0,0 +1,181 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
namespace WeMini;
|
||||
|
||||
use WeChat\Contracts\BasicWeChat;
|
||||
|
||||
/**
|
||||
* 小程序即时配送
|
||||
* Class Delivery
|
||||
* @package WeMini
|
||||
*/
|
||||
class Delivery extends BasicWeChat
|
||||
{
|
||||
|
||||
/**
|
||||
* 异常件退回商家商家确认收货接口
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function abnormalConfirm($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/express/local/business/order/confirm_return?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 下配送单接口
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function addOrder($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/express/local/business/order/add?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 可以对待接单状态的订单增加小费
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function addTip($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/express/local/business/order/addtips?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 取消配送单接口
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function cancelOrder($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/express/local/business/order/cancel?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 获取已支持的配送公司列表接口
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getAllImmeDelivery($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/express/local/business/delivery/getall?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 拉取已绑定账号
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getBindAccount($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/express/local/business/shop/get?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 拉取配送单信息
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getOrder($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/express/local/business/order/get?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 模拟配送公司更新配送单状态
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function mockUpdateOrder($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/express/local/business/test_update_order?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 预下配送单接口
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function preAddOrder($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/express/local/business/order/pre_add?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 预取消配送单接口
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function preCancelOrder($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/express/local/business/order/precancel?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 重新下单
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function reOrder($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/express/local/business/order/readd?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
}
|
72
WeMini/Image.php
Normal file
72
WeMini/Image.php
Normal file
@ -0,0 +1,72 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
namespace WeMini;
|
||||
|
||||
use WeChat\Contracts\BasicWeChat;
|
||||
use WeChat\Exceptions\InvalidResponseException;
|
||||
|
||||
/**
|
||||
* 小程序图像处理
|
||||
* Class Image
|
||||
* @package WeMini
|
||||
*/
|
||||
class Image extends BasicWeChat
|
||||
{
|
||||
|
||||
/**
|
||||
* 本接口提供基于小程序的图片智能裁剪能力
|
||||
* @param string $img_url 要检测的图片 url,传这个则不用传 img 参数。
|
||||
* @param string $img form-data 中媒体文件标识,有filename、filelength、content-type等信息,传这个则不用穿 img_url
|
||||
* @return array
|
||||
* @throws InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function aiCrop($img_url, $img)
|
||||
{
|
||||
$url = "https://api.weixin.qq.com/cv/img/aicrop?access_token=ACCESS_TOCKEN";
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, ['img_url' => $img_url, 'img' => $img], true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 本接口提供基于小程序的条码/二维码识别的API
|
||||
* @param string $img_url 要检测的图片 url,传这个则不用传 img 参数。
|
||||
* @param string $img form-data 中媒体文件标识,有filename、filelength、content-type等信息,传这个则不用穿 img_url
|
||||
* @return array
|
||||
* @throws InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function scanQRCode($img_url, $img)
|
||||
{
|
||||
$url = "https://api.weixin.qq.com/cv/img/qrcode?img_url=ENCODE_URL&access_token=ACCESS_TOCKEN";
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, ['img_url' => $img_url, 'img' => $img], true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 本接口提供基于小程序的图片高清化能力
|
||||
* @param string $img_url 要检测的图片 url,传这个则不用传 img 参数
|
||||
* @param string $img form-data 中媒体文件标识,有filename、filelength、content-type等信息,传这个则不用穿 img_url
|
||||
* @return array
|
||||
* @throws InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function superresolution($img_url, $img)
|
||||
{
|
||||
$url = "https://api.weixin.qq.com/cv/img/qrcode?img_url=ENCODE_URL&access_token=ACCESS_TOCKEN";
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, ['img_url' => $img_url, 'img' => $img], true);
|
||||
}
|
||||
}
|
206
WeMini/Logistics.php
Normal file
206
WeMini/Logistics.php
Normal file
@ -0,0 +1,206 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
namespace WeMini;
|
||||
|
||||
use WeChat\Contracts\BasicWeChat;
|
||||
|
||||
/**
|
||||
* 小程序物流助手
|
||||
* Class Logistics
|
||||
* @package WeMini
|
||||
*/
|
||||
class Logistics extends BasicWeChat
|
||||
{
|
||||
/**
|
||||
* 生成运单
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function addOrder($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/express/business/order/add?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 取消运单
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function cancelOrder($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/express/business/order/cancel?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 获取支持的快递公司列表
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getAllDelivery()
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/express/business/delivery/getall?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callGetApi($url);
|
||||
}
|
||||
|
||||
/**
|
||||
* 获取运单数据
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getOrder($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/express/business/order/get?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 查询运单轨迹
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getPath($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/express/business/path/get?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 获取打印员。若需要使用微信打单 PC 软件,才需要调用
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getPrinter()
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/express/business/printer/getall?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callGetApi($url);
|
||||
}
|
||||
|
||||
/**
|
||||
* 获取电子面单余额。仅在使用加盟类快递公司时,才可以调用
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getQuota($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/express/business/path/get?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 模拟快递公司更新订单状态, 该接口只能用户测试
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function testUpdateOrder($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/express/business/test_update_order?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 配置面单打印员,若需要使用微信打单 PC 软件,才需要调用
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function updatePrinter($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/express/business/printer/update?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 获取面单联系人信息
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getContact($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/express/delivery/contact/get?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 预览面单模板。用于调试面单模板使用
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function previewTemplate($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/express/delivery/template/preview?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 更新商户审核结果
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function updateBusiness($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/express/delivery/service/business/update?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 更新运单轨迹
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function updatePath($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/express/delivery/path/update?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
}
|
67
WeMini/Message.php
Normal file
67
WeMini/Message.php
Normal file
@ -0,0 +1,67 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
namespace WeMini;
|
||||
|
||||
use WeChat\Contracts\BasicWeChat;
|
||||
|
||||
/**
|
||||
* 小程序动态消息
|
||||
* Class Message
|
||||
* @package WeMini
|
||||
*/
|
||||
class Message extends BasicWeChat
|
||||
{
|
||||
/**
|
||||
* 动态消息,创建被分享动态消息的 activity_id
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function createActivityId($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/message/wxopen/activityid/create?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 动态消息,修改被分享的动态消息
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function setUpdatableMsg($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/message/wxopen/updatablemsg/send?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 下发小程序和公众号统一的服务消息
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function uniformSend($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/message/wxopen/template/uniform_send?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
}
|
110
WeMini/Ocr.php
Normal file
110
WeMini/Ocr.php
Normal file
@ -0,0 +1,110 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
namespace WeMini;
|
||||
|
||||
use WeChat\Contracts\BasicWeChat;
|
||||
|
||||
/**
|
||||
* 小程序ORC服务
|
||||
* Class Ocr
|
||||
* @package WeMini
|
||||
*/
|
||||
class Ocr extends BasicWeChat
|
||||
{
|
||||
/**
|
||||
* 本接口提供基于小程序的银行卡 OCR 识别
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function bankcard($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cv/ocr/bankcard?access_token=ACCESS_TOCKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 本接口提供基于小程序的营业执照 OCR 识别
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function businessLicense($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cv/ocr/bizlicense?access_token=ACCESS_TOCKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 本接口提供基于小程序的驾驶证 OCR 识别
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function driverLicense($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cv/ocr/drivinglicense?access_token=ACCESS_TOCKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 本接口提供基于小程序的身份证 OCR 识别
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function idcard($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cv/ocr/idcard?access_token=ACCESS_TOCKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 本接口提供基于小程序的通用印刷体 OCR 识别
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function printedText($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cv/ocr/comm?access_token=ACCESS_TOCKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 本接口提供基于小程序的行驶证 OCR 识别
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function vehicleLicense($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cv/ocr/driving?access_token=ACCESS_TOCKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
}
|
112
WeMini/Plugs.php
Normal file
112
WeMini/Plugs.php
Normal file
@ -0,0 +1,112 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
namespace WeMini;
|
||||
|
||||
use WeChat\Contracts\BasicWeChat;
|
||||
|
||||
/**
|
||||
* 微信小程序插件管理
|
||||
* Class Plugs
|
||||
* @package WeMini
|
||||
*/
|
||||
class Plugs extends BasicWeChat
|
||||
{
|
||||
/**
|
||||
* 1.申请使用插件
|
||||
* @param string $plugin_appid 插件appid
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function apply($plugin_appid)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/wxa/plugin?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, ['action' => 'apply', 'plugin_appid' => $plugin_appid], true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 2.查询已添加的插件
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getList()
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/wxa/plugin?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, ['action' => 'list'], true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 3.删除已添加的插件
|
||||
* @param string $plugin_appid 插件appid
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function unbind($plugin_appid)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/wxa/plugin?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, ['action' => 'unbind', 'plugin_appid' => $plugin_appid], true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 获取当前所有插件使用方
|
||||
* 修改插件使用申请的状态
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function devplugin($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/wxa/devplugin?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 4.获取当前所有插件使用方(供插件开发者调用)
|
||||
* @param integer $page 拉取第page页的数据
|
||||
* @param integer $num 表示每页num条记录
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function devApplyList($page = 1, $num = 10)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/wxa/plugin?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
$data = ['action' => 'dev_apply_list', 'page' => $page, 'num' => $num];
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 5.修改插件使用申请的状态(供插件开发者调用)
|
||||
* @param string $action dev_agree:同意申请;dev_refuse:拒绝申请;dev_delete:删除已拒绝的申请者
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function devAgree($action = 'dev_agree')
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/wxa/plugin?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, ['action' => $action], true);
|
||||
}
|
||||
|
||||
}
|
91
WeMini/Poi.php
Normal file
91
WeMini/Poi.php
Normal file
@ -0,0 +1,91 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
namespace WeMini;
|
||||
|
||||
use WeChat\Contracts\BasicWeChat;
|
||||
|
||||
/**
|
||||
* 微信小程序地址管理
|
||||
* Class Poi
|
||||
* @package WeMini
|
||||
*/
|
||||
class Poi extends BasicWeChat
|
||||
{
|
||||
/**
|
||||
* 添加地点
|
||||
* @param string $related_name 经营资质主体
|
||||
* @param string $related_credential 经营资质证件号
|
||||
* @param string $related_address 经营资质地址
|
||||
* @param string $related_proof_material 相关证明材料照片临时素材mediaid
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function addBearByPoi($related_name, $related_credential, $related_address, $related_proof_material)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/wxa/addnearbypoi?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
$data = [
|
||||
'related_name' => $related_name, 'related_credential' => $related_credential,
|
||||
'related_address' => $related_address, 'related_proof_material' => $related_proof_material,
|
||||
];
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 查看地点列表
|
||||
* @param integer $page 起始页id(从1开始计数)
|
||||
* @param integer $page_rows 每页展示个数(最多1000个)
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getNearByPoiList($page = 1, $page_rows = 1000)
|
||||
{
|
||||
$url = "https://api.weixin.qq.com/wxa/getnearbypoilist?page={$page}&page_rows={$page_rows}&access_token=ACCESS_TOKEN";
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callGetApi($url);
|
||||
}
|
||||
|
||||
/**
|
||||
* 删除地点
|
||||
* @param string $poi_id 附近地点ID
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function delNearByPoiList($poi_id)
|
||||
{
|
||||
$url = "https://api.weixin.qq.com/wxa/delnearbypoi?access_token=ACCESS_TOKEN";
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, ['poi_id' => $poi_id], true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 展示/取消展示附近小程序
|
||||
* @param string $poi_id 附近地点ID
|
||||
* @param string $status 0:取消展示;1:展示
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function setNearByPoiShowStatus($poi_id, $status)
|
||||
{
|
||||
$url = "https://api.weixin.qq.com/wxa/setnearbypoishowstatus?access_token=ACCESS_TOKEN";
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, ['poi_id' => $poi_id, 'status' => $status], true);
|
||||
}
|
||||
|
||||
}
|
112
WeMini/Qrcode.php
Normal file
112
WeMini/Qrcode.php
Normal file
@ -0,0 +1,112 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
namespace WeMini;
|
||||
|
||||
use WeChat\Contracts\BasicWeChat;
|
||||
use WeChat\Contracts\Tools;
|
||||
|
||||
/**
|
||||
* 微信小程序二维码管理
|
||||
* Class Qrcode
|
||||
* @package WeMini
|
||||
*/
|
||||
class Qrcode extends BasicWeChat
|
||||
{
|
||||
|
||||
/**
|
||||
* 获取小程序码(永久有效)
|
||||
* 接口A: 适用于需要的码数量较少的业务场景
|
||||
* @param string $path 不能为空,最大长度 128 字节
|
||||
* @param integer $width 二维码的宽度
|
||||
* @param bool $auto_color 自动配置线条颜色,如果颜色依然是黑色,则说明不建议配置主色调
|
||||
* @param array $line_color auto_color 为 false 时生效
|
||||
* @param boolean $is_hyaline 是否需要透明底色
|
||||
* @param null|string $outType 输出类型
|
||||
* @return array|string
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function createMiniPath($path, $width = 430, $auto_color = false, $line_color = ["r" => "0", "g" => "0", "b" => "0"], $is_hyaline = true, $outType = null)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/wxa/getwxacode?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
$data = ['path' => $path, 'width' => $width, 'auto_color' => $auto_color, 'line_color' => $line_color, 'is_hyaline' => $is_hyaline];
|
||||
$result = Tools::post($url, Tools::arr2json($data));
|
||||
if (is_array($json = json_decode($result, true))) {
|
||||
if (!$this->isTry && isset($json['errcode']) && in_array($json['errcode'], ['40014', '40001', '41001', '42001'])) {
|
||||
[$this->delAccessToken(), $this->isTry = true];
|
||||
return call_user_func_array([$this, $this->currentMethod['method']], $this->currentMethod['arguments']);
|
||||
}
|
||||
return Tools::json2arr($result);
|
||||
}
|
||||
return is_null($outType) ? $result : $outType($result);
|
||||
}
|
||||
|
||||
/**
|
||||
* 获取小程序码(永久有效)
|
||||
* 接口B:适用于需要的码数量极多的业务场景
|
||||
* @param string $scene 最大32个可见字符,只支持数字
|
||||
* @param string $page 必须是已经发布的小程序存在的页面
|
||||
* @param integer $width 二维码的宽度
|
||||
* @param bool $auto_color 自动配置线条颜色,如果颜色依然是黑色,则说明不建议配置主色调
|
||||
* @param array $line_color auto_color 为 false 时生效
|
||||
* @param boolean $is_hyaline 是否需要透明底色
|
||||
* @param null|string $outType 输出类型
|
||||
* @return array|string
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function createMiniScene($scene, $page, $width = 430, $auto_color = false, $line_color = ["r" => "0", "g" => "0", "b" => "0"], $is_hyaline = true, $outType = null)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/wxa/getwxacodeunlimit?access_token=ACCESS_TOKEN';
|
||||
$data = ['scene' => $scene, 'width' => $width, 'auto_color' => $auto_color, 'page' => $page, 'line_color' => $line_color, 'is_hyaline' => $is_hyaline];
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
$result = Tools::post($url, Tools::arr2json($data));
|
||||
if (is_array($json = json_decode($result, true))) {
|
||||
if (!$this->isTry && isset($json['errcode']) && in_array($json['errcode'], ['40014', '40001', '41001', '42001'])) {
|
||||
[$this->delAccessToken(), $this->isTry = true];
|
||||
return call_user_func_array([$this, $this->currentMethod['method']], $this->currentMethod['arguments']);
|
||||
}
|
||||
return Tools::json2arr($result);
|
||||
}
|
||||
return is_null($outType) ? $result : $outType($result);
|
||||
}
|
||||
|
||||
/**
|
||||
* 获取小程序二维码(永久有效)
|
||||
* 接口C:适用于需要的码数量较少的业务场景
|
||||
* @param string $path 不能为空,最大长度 128 字节
|
||||
* @param integer $width 二维码的宽度
|
||||
* @param null|string $outType 输出类型
|
||||
* @return array|string
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function createDefault($path, $width = 430, $outType = null)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/wxaapp/createwxaqrcode?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
$result = Tools::post($url, Tools::arr2json(['path' => $path, 'width' => $width]));
|
||||
if (is_array($json = json_decode($result, true))) {
|
||||
if (!$this->isTry && isset($json['errcode']) && in_array($json['errcode'], ['40014', '40001', '41001', '42001'])) {
|
||||
[$this->delAccessToken(), $this->isTry = true];
|
||||
return call_user_func_array([$this, $this->currentMethod['method']], $this->currentMethod['arguments']);
|
||||
}
|
||||
return Tools::json2arr($result);
|
||||
}
|
||||
return is_null($outType) ? $result : $outType($result);
|
||||
}
|
||||
|
||||
}
|
70
WeMini/Security.php
Normal file
70
WeMini/Security.php
Normal file
@ -0,0 +1,70 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
namespace WeMini;
|
||||
|
||||
use WeChat\Contracts\BasicWeChat;
|
||||
use WeChat\Exceptions\InvalidResponseException;
|
||||
|
||||
/**
|
||||
* 小程序内容安全
|
||||
* Class Security
|
||||
* @package WeMini
|
||||
*/
|
||||
class Security extends BasicWeChat
|
||||
{
|
||||
|
||||
/**
|
||||
* 校验一张图片是否含有违法违规内容
|
||||
* @param string $media 要检测的图片文件,格式支持PNG、JPEG、JPG、GIF,图片尺寸不超过 750px x 1334px
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function imgSecCheck($media)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/wxa/img_sec_check?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, ['media' => $media], true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 异步校验图片/音频是否含有违法违规内容
|
||||
* @param string $media_url
|
||||
* @param string $media_type
|
||||
* @return array
|
||||
* @throws InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function mediaCheckAsync($media_url, $media_type)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/wxa/media_check_async?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, ['media_url' => $media_url, 'media_type' => $media_type], true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 检查一段文本是否含有违法违规内容
|
||||
* @param string $content
|
||||
* @return array
|
||||
* @throws InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function msgSecCheck($content)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/wxa/msg_sec_check?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, ['content' => $content], true);
|
||||
}
|
||||
}
|
40
WeMini/Soter.php
Normal file
40
WeMini/Soter.php
Normal file
@ -0,0 +1,40 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
namespace WeMini;
|
||||
|
||||
use WeChat\Contracts\BasicWeChat;
|
||||
|
||||
/**
|
||||
* 小程序生物认证
|
||||
* Class Soter
|
||||
* @package WeMini
|
||||
*/
|
||||
class Soter extends BasicWeChat
|
||||
{
|
||||
/**
|
||||
* SOTER 生物认证秘钥签名验证
|
||||
* @param array $data
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function verifySignature($data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/soter/verify_signature?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
}
|
110
WeMini/Template.php
Normal file
110
WeMini/Template.php
Normal file
@ -0,0 +1,110 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
namespace WeMini;
|
||||
|
||||
use WeChat\Contracts\BasicWeChat;
|
||||
|
||||
/**
|
||||
* 公众号小程序模板消息支持
|
||||
* Class Mini
|
||||
* @package WeChat
|
||||
*/
|
||||
class Template extends BasicWeChat
|
||||
{
|
||||
|
||||
/**
|
||||
* 获取小程序模板库标题列表
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getTemplateLibraryList()
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/wxopen/template/library/list?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, ['offset' => '0', 'count' => '20'], true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 获取模板库某个模板标题下关键词库
|
||||
* @param string $template_id 模板标题id,可通过接口获取,也可登录小程序后台查看获取
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getTemplateLibrary($template_id)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/wxopen/template/library/get?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, ['id' => $template_id], true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 组合模板并添加至帐号下的个人模板库
|
||||
* @param string $template_id 模板标题id,可通过接口获取,也可登录小程序后台查看获取
|
||||
* @param array $keyword_id_list 开发者自行组合好的模板关键词列表,关键词顺序可以自由搭配(例如[3,5,4]或[4,5,3]),最多支持10个关键词组合
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function addTemplate($template_id, array $keyword_id_list)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/wxopen/template/add?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, ['id' => $template_id, 'keyword_id_list' => $keyword_id_list], true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 获取帐号下已存在的模板列表
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getTemplateList()
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/wxopen/template/list?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, ['offset' => '0', 'count' => '20'], true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 删除模板消息
|
||||
* @param string $template_id 要删除的模板id
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function delTemplate($template_id)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/wxopen/template/del?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, ['template_id' => $template_id], true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 发送模板消息
|
||||
* @param array $data 发送的消息对象数组
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function send(array $data)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/cgi-bin/message/wxopen/template/send?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, $data, true);
|
||||
}
|
||||
|
||||
}
|
176
WeMini/Total.php
Normal file
176
WeMini/Total.php
Normal file
@ -0,0 +1,176 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
namespace WeMini;
|
||||
|
||||
use WeChat\Contracts\BasicWeChat;
|
||||
|
||||
/**
|
||||
* 微信小程序数据接口
|
||||
* Class Total
|
||||
* @package WeMini
|
||||
*/
|
||||
class Total extends BasicWeChat
|
||||
{
|
||||
/**
|
||||
* 数据分析接口
|
||||
* @param string $begin_date 开始日期
|
||||
* @param string $end_date 结束日期,限定查询1天数据,end_date允许设置的最大值为昨日
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getWeanalysisAppidDailySummarytrend($begin_date, $end_date)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/datacube/getweanalysisappiddailysummarytrend?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, ['begin_date' => $begin_date, 'end_date' => $end_date], true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 访问分析
|
||||
* @param string $begin_date 开始日期
|
||||
* @param string $end_date 结束日期,限定查询1天数据,end_date允许设置的最大值为昨日
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getWeanalysisAppidDailyVisittrend($begin_date, $end_date)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/datacube/getweanalysisappiddailyvisittrend?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, ['begin_date' => $begin_date, 'end_date' => $end_date], true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 周趋势
|
||||
* @param string $begin_date 开始日期,为周一日期
|
||||
* @param string $end_date 结束日期,为周日日期,限定查询一周数据
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getWeanalysisAppidWeeklyVisittrend($begin_date, $end_date)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/datacube/getweanalysisappidweeklyvisittrend?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, ['begin_date' => $begin_date, 'end_date' => $end_date], true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 月趋势
|
||||
* @param string $begin_date 开始日期,为自然月第一天
|
||||
* @param string $end_date 结束日期,为自然月最后一天,限定查询一个月数据
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getWeanalysisAppidMonthlyVisittrend($begin_date, $end_date)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/datacube/getweanalysisappidmonthlyvisittrend?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, ['begin_date' => $begin_date, 'end_date' => $end_date], true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 访问分布
|
||||
* @param string $begin_date 开始日期
|
||||
* @param string $end_date 结束日期,限定查询1天数据,end_date允许设置的最大值为昨日
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getWeanalysisAppidVisitdistribution($begin_date, $end_date)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/datacube/getweanalysisappidvisitdistribution?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, ['begin_date' => $begin_date, 'end_date' => $end_date], true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 日留存
|
||||
* @param string $begin_date 开始日期
|
||||
* @param string $end_date 结束日期,限定查询1天数据,end_date允许设置的最大值为昨日
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getWeanalysisAppidDailyRetaininfo($begin_date, $end_date)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/datacube/getweanalysisappiddailyretaininfo?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, ['begin_date' => $begin_date, 'end_date' => $end_date], true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 周留存
|
||||
* @param string $begin_date 开始日期,为周一日期
|
||||
* @param string $end_date 结束日期,为周日日期,限定查询一周数据
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getWeanalysisAppidWeeklyRetaininfo($begin_date, $end_date)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/datacube/getweanalysisappidweeklyretaininfo?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, ['begin_date' => $begin_date, 'end_date' => $end_date], true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 月留存
|
||||
* @param string $begin_date 开始日期,为自然月第一天
|
||||
* @param string $end_date 结束日期,为自然月最后一天,限定查询一个月数据
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getWeanalysisAppidMonthlyRetaininfo($begin_date, $end_date)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/datacube/getweanalysisappidmonthlyretaininfo?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, ['begin_date' => $begin_date, 'end_date' => $end_date], true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 访问页面
|
||||
* @param string $begin_date 开始日期
|
||||
* @param string $end_date 结束日期,限定查询1天数据,end_date允许设置的最大值为昨日
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getWeanalysisAppidVisitPage($begin_date, $end_date)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/datacube/getweanalysisappidvisitpage?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, ['begin_date' => $begin_date, 'end_date' => $end_date], true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 用户画像
|
||||
* @param string $begin_date 开始日期
|
||||
* @param string $end_date 结束日期,开始日期与结束日期相差的天数限定为0/6/29,分别表示查询最近1/7/30天数据,end_date允许设置的最大值为昨日
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function getWeanalysisAppidUserportrait($begin_date, $end_date)
|
||||
{
|
||||
$url = 'https://api.weixin.qq.com/datacube/getweanalysisappiduserportrait?access_token=ACCESS_TOKEN';
|
||||
$this->registerApi($url, __FUNCTION__, func_get_args());
|
||||
return $this->callPostApi($url, ['begin_date' => $begin_date, 'end_date' => $end_date], true);
|
||||
}
|
||||
|
||||
}
|
20
WeMini/crypt/errorCode.php
Normal file
20
WeMini/crypt/errorCode.php
Normal file
@ -0,0 +1,20 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
* error code 说明.
|
||||
* <ul>
|
||||
* <li>-41001: encodingAesKey 非法</li>
|
||||
* <li>-41003: aes 解密失败</li>
|
||||
* <li>-41004: 解密后得到的buffer非法</li>
|
||||
* <li>-41005: base64加密失败</li>
|
||||
* <li>-41016: base64解密失败</li>
|
||||
* </ul>
|
||||
*/
|
||||
class ErrorCode
|
||||
{
|
||||
public static $OK = 0;
|
||||
public static $IllegalAesKey = -41001;
|
||||
public static $IllegalIv = -41002;
|
||||
public static $IllegalBuffer = -41003;
|
||||
public static $DecodeBase64Error = -41004;
|
||||
}
|
57
WeMini/crypt/wxBizDataCrypt.php
Normal file
57
WeMini/crypt/wxBizDataCrypt.php
Normal file
@ -0,0 +1,57 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
* 对微信小程序用户加密数据的解密示例代码
|
||||
* Class WXBizDataCrypt
|
||||
* @copyright Copyright (c) 1998-2014 Tencent Inc.
|
||||
*/
|
||||
class WXBizDataCrypt
|
||||
{
|
||||
private $appid;
|
||||
private $sessionKey;
|
||||
|
||||
/**
|
||||
* 构造函数
|
||||
* @param $sessionKey string 用户在小程序登录后获取的会话密钥
|
||||
* @param $appid string 小程序的appid
|
||||
*/
|
||||
public function __construct($appid, $sessionKey)
|
||||
{
|
||||
$this->appid = $appid;
|
||||
$this->sessionKey = $sessionKey;
|
||||
include_once __DIR__ . DIRECTORY_SEPARATOR . "errorCode.php";
|
||||
}
|
||||
|
||||
/**
|
||||
* 检验数据的真实性,并且获取解密后的明文.
|
||||
* @param $encryptedData string 加密的用户数据
|
||||
* @param $iv string 与用户数据一同返回的初始向量
|
||||
* @param $data string 解密后的原文
|
||||
*
|
||||
* @return int 成功0,失败返回对应的错误码
|
||||
*/
|
||||
public function decryptData($encryptedData, $iv, &$data)
|
||||
{
|
||||
if (strlen($this->sessionKey) != 24) {
|
||||
return \ErrorCode::$IllegalAesKey;
|
||||
}
|
||||
$aesKey = base64_decode($this->sessionKey);
|
||||
if (strlen($iv) != 24) {
|
||||
return \ErrorCode::$IllegalIv;
|
||||
}
|
||||
$aesIV = base64_decode($iv);
|
||||
$aesCipher = base64_decode($encryptedData);
|
||||
$result = openssl_decrypt($aesCipher, "AES-128-CBC", $aesKey, 1, $aesIV);
|
||||
$dataObj = json_decode($result);
|
||||
if ($dataObj == null) {
|
||||
return \ErrorCode::$IllegalBuffer;
|
||||
}
|
||||
if ($dataObj->watermark->appid != $this->appid) {
|
||||
return \ErrorCode::$IllegalBuffer;
|
||||
}
|
||||
$data = $result;
|
||||
return \ErrorCode::$OK;
|
||||
}
|
||||
|
||||
}
|
||||
|
63
WePay/Bill.php
Normal file
63
WePay/Bill.php
Normal file
@ -0,0 +1,63 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
namespace WePay;
|
||||
|
||||
use WeChat\Contracts\BasicWePay;
|
||||
use WeChat\Contracts\Tools;
|
||||
use WeChat\Exceptions\InvalidResponseException;
|
||||
|
||||
/**
|
||||
* 微信商户账单及评论
|
||||
* Class Bill
|
||||
* @package WePay
|
||||
*/
|
||||
class Bill extends BasicWePay
|
||||
{
|
||||
/**
|
||||
* 下载对账单
|
||||
* @param array $options 静音参数
|
||||
* @param null|string $outType 输出类型
|
||||
* @return bool|string
|
||||
* @throws InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function download(array $options, $outType = null)
|
||||
{
|
||||
$this->params->set('sign_type', 'MD5');
|
||||
$params = $this->params->merge($options);
|
||||
$params['sign'] = $this->getPaySign($params, 'MD5');
|
||||
$result = Tools::post('https://api.mch.weixin.qq.com/pay/downloadbill', Tools::arr2xml($params));
|
||||
if (($jsonData = Tools::xml2arr($result))) {
|
||||
if ($jsonData['return_code'] !== 'SUCCESS') {
|
||||
throw new InvalidResponseException($jsonData['return_msg'], '0');
|
||||
}
|
||||
}
|
||||
return is_null($outType) ? $result : $outType($result);
|
||||
}
|
||||
|
||||
|
||||
/**
|
||||
* 拉取订单评价数据
|
||||
* @param array $options
|
||||
* @return array
|
||||
* @throws InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function comment(array $options)
|
||||
{
|
||||
$url = 'https://api.mch.weixin.qq.com/billcommentsp/batchquerycomment';
|
||||
return $this->callPostApi($url, $options, true);
|
||||
}
|
||||
}
|
65
WePay/Coupon.php
Normal file
65
WePay/Coupon.php
Normal file
@ -0,0 +1,65 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
namespace WePay;
|
||||
|
||||
use WeChat\Contracts\BasicWePay;
|
||||
|
||||
/**
|
||||
* 微信商户代金券
|
||||
* Class Coupon
|
||||
* @package WePay
|
||||
*/
|
||||
class Coupon extends BasicWePay
|
||||
{
|
||||
/**
|
||||
* 发放代金券
|
||||
* @param array $options
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function create(array $options)
|
||||
{
|
||||
$url = "https://api.mch.weixin.qq.com/mmpaymkttransfers/send_coupon";
|
||||
return $this->callPostApi($url, $options, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 查询代金券批次
|
||||
* @param array $options
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function queryStock(array $options)
|
||||
{
|
||||
$url = "https://api.mch.weixin.qq.com/mmpaymkttransfers/query_coupon_stock";
|
||||
return $this->callPostApi($url, $options, false);
|
||||
}
|
||||
|
||||
/**
|
||||
* 查询代金券信息
|
||||
* @param array $options
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function queryInfo(array $options)
|
||||
{
|
||||
$url = "https://api.mch.weixin.qq.com/mmpaymkttransfers/query_coupon_stock";
|
||||
return $this->callPostApi($url, $options, false);
|
||||
}
|
||||
|
||||
}
|
173
WePay/Order.php
Normal file
173
WePay/Order.php
Normal file
@ -0,0 +1,173 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
namespace WePay;
|
||||
|
||||
use WeChat\Contracts\BasicWePay;
|
||||
use WeChat\Contracts\Tools;
|
||||
|
||||
/**
|
||||
* 微信商户订单
|
||||
* Class Order
|
||||
* @package WePay
|
||||
*/
|
||||
class Order extends BasicWePay
|
||||
{
|
||||
|
||||
/**
|
||||
* 统一下单
|
||||
* @param array $options
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function create(array $options)
|
||||
{
|
||||
$url = 'https://api.mch.weixin.qq.com/pay/unifiedorder';
|
||||
return $this->callPostApi($url, $options, false, 'MD5');
|
||||
}
|
||||
|
||||
/**
|
||||
* 刷卡支付
|
||||
* @param array $options
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function micropay(array $options)
|
||||
{
|
||||
$url = 'https://api.mch.weixin.qq.com/pay/micropay';
|
||||
return $this->callPostApi($url, $options, false, 'MD5');
|
||||
}
|
||||
|
||||
/**
|
||||
* 查询订单
|
||||
* @param array $options
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function query(array $options)
|
||||
{
|
||||
$url = 'https://api.mch.weixin.qq.com/pay/orderquery';
|
||||
return $this->callPostApi($url, $options);
|
||||
}
|
||||
|
||||
/**
|
||||
* 关闭订单
|
||||
* @param string $outTradeNo 商户订单号
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function close($outTradeNo)
|
||||
{
|
||||
$url = 'https://api.mch.weixin.qq.com/pay/closeorder';
|
||||
return $this->callPostApi($url, ['out_trade_no' => $outTradeNo]);
|
||||
}
|
||||
|
||||
/**
|
||||
* 创建JsApi及H5支付参数
|
||||
* @param string $prepayId 统一下单预支付码
|
||||
* @return array
|
||||
*/
|
||||
public function jsapiParams($prepayId)
|
||||
{
|
||||
$option = [];
|
||||
$option["appId"] = $this->config->get('appid');
|
||||
$option["timeStamp"] = (string)time();
|
||||
$option["nonceStr"] = Tools::createNoncestr();
|
||||
$option["package"] = "prepay_id={$prepayId}";
|
||||
$option["signType"] = "MD5";
|
||||
$option["paySign"] = $this->getPaySign($option, 'MD5');
|
||||
$option['timestamp'] = $option['timeStamp'];
|
||||
return $option;
|
||||
}
|
||||
|
||||
/**
|
||||
* 获取支付规则二维码
|
||||
* @param string $productId 商户定义的商品id或者订单号
|
||||
* @return string
|
||||
*/
|
||||
public function qrcParams($productId)
|
||||
{
|
||||
$data = [
|
||||
'appid' => $this->config->get('appid'),
|
||||
'mch_id' => $this->config->get('mch_id'),
|
||||
'time_stamp' => (string)time(),
|
||||
'nonce_str' => Tools::createNoncestr(),
|
||||
'product_id' => (string)$productId,
|
||||
];
|
||||
$data['sign'] = $this->getPaySign($data, 'MD5');
|
||||
return "weixin://wxpay/bizpayurl?" . http_build_query($data);
|
||||
}
|
||||
|
||||
/**
|
||||
* 获取微信App支付秘需参数
|
||||
* @param string $prepayId 统一下单预支付码
|
||||
* @return array
|
||||
*/
|
||||
public function appParams($prepayId)
|
||||
{
|
||||
$data = [
|
||||
'appid' => $this->config->get('appid'),
|
||||
'partnerid' => $this->config->get('mch_id'),
|
||||
'prepayid' => (string)$prepayId,
|
||||
'package' => 'Sign=WXPay',
|
||||
'timestamp' => (string)time(),
|
||||
'noncestr' => Tools::createNoncestr(),
|
||||
];
|
||||
$data['sign'] = $this->getPaySign($data, 'MD5');
|
||||
return $data;
|
||||
}
|
||||
|
||||
/**
|
||||
* 刷卡支付 撤销订单
|
||||
* @param array $options
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function reverse(array $options)
|
||||
{
|
||||
$url = 'https://api.mch.weixin.qq.com/secapi/pay/reverse';
|
||||
return $this->callPostApi($url, $options, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 刷卡支付 授权码查询openid
|
||||
* @param string $authCode 扫码支付授权码,设备读取用户微信中的条码或者二维码信息
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function queryAuthCode($authCode)
|
||||
{
|
||||
$url = 'https://api.mch.weixin.qq.com/tools/authcodetoopenid';
|
||||
return $this->callPostApi($url, ['auth_code' => $authCode]);
|
||||
}
|
||||
|
||||
/**
|
||||
* 刷卡支付 交易保障
|
||||
* @param array $options
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function report(array $options)
|
||||
{
|
||||
$url = 'https://api.mch.weixin.qq.com/payitil/report';
|
||||
return $this->callPostApi($url, $options);
|
||||
}
|
||||
}
|
72
WePay/Redpack.php
Normal file
72
WePay/Redpack.php
Normal file
@ -0,0 +1,72 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
namespace WePay;
|
||||
|
||||
use WeChat\Contracts\BasicWePay;
|
||||
|
||||
/**
|
||||
* 微信红包支持
|
||||
* Class Redpack
|
||||
* @package WePay
|
||||
*/
|
||||
class Redpack extends BasicWePay
|
||||
{
|
||||
|
||||
/**
|
||||
* 发放普通红包
|
||||
* @param array $options
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function create(array $options)
|
||||
{
|
||||
$this->params->offsetUnset('appid');
|
||||
$this->params->set('wxappid', $this->config->get('appid'));
|
||||
$url = "https://api.mch.weixin.qq.com/mmpaymkttransfers/sendredpack";
|
||||
return $this->callPostApi($url, $options, true, 'MD5', false);
|
||||
}
|
||||
|
||||
/**
|
||||
* 发放裂变红包
|
||||
* @param array $options
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function groups(array $options)
|
||||
{
|
||||
$this->params->offsetUnset('appid');
|
||||
$this->params->set('wxappid', $this->config->get('appid'));
|
||||
$url = "https://api.mch.weixin.qq.com/mmpaymkttransfers/sendgroupredpack";
|
||||
return $this->callPostApi($url, $options, true, 'MD5', false);
|
||||
}
|
||||
|
||||
/**
|
||||
* 查询红包记录
|
||||
* @param string $mchBillno 商户发放红包的商户订单号
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function query($mchBillno)
|
||||
{
|
||||
$this->params->offsetUnset('wxappid');
|
||||
$this->params->set('appid', $this->config->get('appid'));
|
||||
$url = "https://api.mch.weixin.qq.com/mmpaymkttransfers/gethbinfo";
|
||||
return $this->callPostApi($url, ['mch_billno' => $mchBillno, 'bill_type' => 'MCHT'], true, 'MD5', false);
|
||||
}
|
||||
|
||||
}
|
78
WePay/Refund.php
Normal file
78
WePay/Refund.php
Normal file
@ -0,0 +1,78 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
namespace WePay;
|
||||
|
||||
use WeChat\Contracts\BasicWePay;
|
||||
use WeChat\Contracts\Tools;
|
||||
use WeChat\Exceptions\InvalidResponseException;
|
||||
|
||||
/**
|
||||
* 微信商户退款
|
||||
* Class Refund
|
||||
* @package WePay
|
||||
*/
|
||||
class Refund extends BasicWePay
|
||||
{
|
||||
|
||||
/**
|
||||
* 创建退款订单
|
||||
* @param array $options
|
||||
* @return array
|
||||
* @throws InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function create(array $options)
|
||||
{
|
||||
$url = 'https://api.mch.weixin.qq.com/secapi/pay/refund';
|
||||
return $this->callPostApi($url, $options, true);
|
||||
}
|
||||
|
||||
/**
|
||||
* 查询退款
|
||||
* @param array $options
|
||||
* @return array
|
||||
* @throws InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function query(array $options)
|
||||
{
|
||||
$url = 'https://api.mch.weixin.qq.com/pay/refundquery';
|
||||
return $this->callPostApi($url, $options);
|
||||
}
|
||||
|
||||
/**
|
||||
* 获取退款通知
|
||||
* @return array
|
||||
* @throws InvalidResponseException
|
||||
*/
|
||||
public function getNotify()
|
||||
{
|
||||
$data = Tools::xml2arr(file_get_contents("php://input"));
|
||||
if (!isset($data['return_code']) || $data['return_code'] !== 'SUCCESS') {
|
||||
throw new InvalidResponseException('获取退款通知XML失败!');
|
||||
}
|
||||
if (!class_exists('Prpcrypt', false)) {
|
||||
include dirname(__DIR__) . '/WeChat/Contracts/Prpcrypt.php';
|
||||
}
|
||||
$pc = new \Prpcrypt(md5($this->config->get('mch_key')));
|
||||
$array = $pc->decrypt(base64_decode($data['req_info']));
|
||||
if (intval($array[0]) > 0) {
|
||||
throw new InvalidResponseException($array[1], $array[0]);
|
||||
}
|
||||
$data['decode'] = $array[1];
|
||||
return $data;
|
||||
}
|
||||
|
||||
}
|
61
WePay/Transfers.php
Normal file
61
WePay/Transfers.php
Normal file
@ -0,0 +1,61 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
namespace WePay;
|
||||
|
||||
use WeChat\Contracts\BasicWePay;
|
||||
|
||||
/**
|
||||
* 微信商户打款到零钱
|
||||
* Class Transfers
|
||||
* @package WePay
|
||||
*/
|
||||
class Transfers extends BasicWePay
|
||||
{
|
||||
|
||||
/**
|
||||
* 企业付款到零钱
|
||||
* @param array $options
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function create(array $options)
|
||||
{
|
||||
$this->params->offsetUnset('appid');
|
||||
$this->params->offsetUnset('mch_id');
|
||||
$this->params->set('mchid', $this->config->get('mch_id'));
|
||||
$this->params->set('mch_appid', $this->config->get('appid'));
|
||||
$url = 'https://api.mch.weixin.qq.com/mmpaymkttransfers/promotion/transfers';
|
||||
return $this->callPostApi($url, $options, true, 'MD5', false);
|
||||
}
|
||||
|
||||
/**
|
||||
* 查询企业付款到零钱
|
||||
* @param string $partnerTradeNo 商户调用企业付款API时使用的商户订单号
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function query($partnerTradeNo)
|
||||
{
|
||||
$this->params->offsetUnset('mchid');
|
||||
$this->params->offsetUnset('mch_appid');
|
||||
$this->params->set('appid', $this->config->get('appid'));
|
||||
$this->params->set('mch_id', $this->config->get('mch_id'));
|
||||
$url = 'https://api.mch.weixin.qq.com/mmpaymkttransfers/gettransferinfo';
|
||||
return $this->callPostApi($url, ['partner_trade_no' => $partnerTradeNo], true, 'MD5', false);
|
||||
}
|
||||
|
||||
}
|
125
WePay/TransfersBank.php
Normal file
125
WePay/TransfersBank.php
Normal file
@ -0,0 +1,125 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
namespace WePay;
|
||||
|
||||
use WeChat\Contracts\BasicWePay;
|
||||
use WeChat\Contracts\Tools;
|
||||
use WeChat\Exceptions\InvalidArgumentException;
|
||||
use WeChat\Exceptions\InvalidDecryptException;
|
||||
use WeChat\Exceptions\InvalidResponseException;
|
||||
|
||||
/**
|
||||
* 微信商户打款到银行卡
|
||||
* Class TransfersBank
|
||||
* @package WePay
|
||||
*/
|
||||
class TransfersBank extends BasicWePay
|
||||
{
|
||||
|
||||
/**
|
||||
* 企业付款到银行卡
|
||||
* @param array $options
|
||||
* @return array
|
||||
* @throws \WeChat\Exceptions\InvalidDecryptException
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function create(array $options)
|
||||
{
|
||||
if (!isset($options['partner_trade_no'])) {
|
||||
throw new InvalidArgumentException('Missing Options -- [partner_trade_no]');
|
||||
}
|
||||
if (!isset($options['enc_bank_no'])) {
|
||||
throw new InvalidArgumentException('Missing Options -- [enc_bank_no]');
|
||||
}
|
||||
if (!isset($options['enc_true_name'])) {
|
||||
throw new InvalidArgumentException('Missing Options -- [enc_true_name]');
|
||||
}
|
||||
if (!isset($options['bank_code'])) {
|
||||
throw new InvalidArgumentException('Missing Options -- [bank_code]');
|
||||
}
|
||||
if (!isset($options['amount'])) {
|
||||
throw new InvalidArgumentException('Missing Options -- [amount]');
|
||||
}
|
||||
$this->params->offsetUnset('appid');
|
||||
return $this->callPostApi('https://api.mch.weixin.qq.com/mmpaysptrans/pay_bank', [
|
||||
'amount' => $options['amount'],
|
||||
'bank_code' => $options['bank_code'],
|
||||
'partner_trade_no' => $options['partner_trade_no'],
|
||||
'enc_bank_no' => $this->rsaEncode($options['enc_bank_no']),
|
||||
'enc_true_name' => $this->rsaEncode($options['enc_true_name']),
|
||||
'desc' => isset($options['desc']) ? $options['desc'] : '',
|
||||
], true, 'MD5', false);
|
||||
}
|
||||
|
||||
/**
|
||||
* 商户企业付款到银行卡操作进行结果查询
|
||||
* @param string $partnerTradeNo 商户订单号,需保持唯一
|
||||
* @return array
|
||||
* @throws InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
public function query($partnerTradeNo)
|
||||
{
|
||||
$this->params->offsetUnset('appid');
|
||||
$url = 'https://api.mch.weixin.qq.com/mmpaysptrans/query_bank';
|
||||
return $this->callPostApi($url, ['partner_trade_no' => $partnerTradeNo], true, 'MD5', false);
|
||||
}
|
||||
|
||||
/**
|
||||
* RSA加密处理
|
||||
* @param string $string
|
||||
* @param string $encrypted
|
||||
* @return string
|
||||
* @throws \WeChat\Exceptions\InvalidDecryptException
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
private function rsaEncode($string, $encrypted = '')
|
||||
{
|
||||
$search = ['-----BEGIN RSA PUBLIC KEY-----', '-----END RSA PUBLIC KEY-----', "\n", "\r"];
|
||||
$pkc1 = str_replace($search, '', $this->getRsaContent());
|
||||
$publicKey = '-----BEGIN PUBLIC KEY-----' . PHP_EOL .
|
||||
wordwrap('MIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8A' . $pkc1, 64, PHP_EOL, true) . PHP_EOL .
|
||||
'-----END PUBLIC KEY-----';
|
||||
if (!openssl_public_encrypt("{$string}", $encrypted, $publicKey, OPENSSL_PKCS1_OAEP_PADDING)) {
|
||||
throw new InvalidDecryptException('Rsa Encrypt Error.');
|
||||
}
|
||||
return base64_encode($encrypted);
|
||||
}
|
||||
|
||||
/**
|
||||
* 获取签名文件内容
|
||||
* @return string
|
||||
* @throws \WeChat\Exceptions\InvalidResponseException
|
||||
* @throws \WeChat\Exceptions\LocalCacheException
|
||||
*/
|
||||
private function getRsaContent()
|
||||
{
|
||||
$cacheKey = "pub_ras_key_" . $this->config->get('mch_id');
|
||||
if (($pub_key = Tools::getCache($cacheKey))) {
|
||||
return $pub_key;
|
||||
}
|
||||
$data = $this->callPostApi('https://fraud.mch.weixin.qq.com/risk/getpublickey', [], true, 'MD5');
|
||||
if (!isset($data['return_code']) || $data['return_code'] !== 'SUCCESS' || $data['result_code'] !== 'SUCCESS') {
|
||||
$error = 'ResultError:' . $data['return_msg'];
|
||||
$error .= isset($data['err_code_des']) ? ' - ' . $data['err_code_des'] : '';
|
||||
throw new InvalidResponseException($error, 20000, $data);
|
||||
}
|
||||
Tools::setCache($cacheKey, $data['pub_key'], 600);
|
||||
return $data['pub_key'];
|
||||
}
|
||||
|
||||
}
|
39
_test/alipay-app.php
Normal file
39
_test/alipay-app.php
Normal file
@ -0,0 +1,39 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
// 1. 手动加载入口文件
|
||||
include "../include.php";
|
||||
|
||||
// 2. 准备公众号配置参数
|
||||
$config = include "./alipay.php";
|
||||
|
||||
try {
|
||||
// 实例支付对象
|
||||
// $pay = \We::AliPayApp($config);
|
||||
// $pay = new \AliPay\App($config);
|
||||
$pay = \AliPay\App::instance($config);
|
||||
|
||||
// 请参考(请求参数):https://docs.open.alipay.com/api_1/alipay.trade.app.pay
|
||||
$result = $pay->apply([
|
||||
'out_trade_no' => time(), // 商户订单号
|
||||
'total_amount' => '1', // 支付金额
|
||||
'subject' => 'test subject', // 支付订单描述
|
||||
]);
|
||||
echo '<pre>';
|
||||
var_export($result);
|
||||
} catch (\Exception $e) {
|
||||
echo $e->getMessage();
|
||||
}
|
||||
|
||||
|
36
_test/alipay-bill.php
Normal file
36
_test/alipay-bill.php
Normal file
@ -0,0 +1,36 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
// 1. 手动加载入口文件
|
||||
include "../include.php";
|
||||
|
||||
// 2. 准备公众号配置参数
|
||||
$config = include "./alipay.php";
|
||||
|
||||
try {
|
||||
// 实例支付对象
|
||||
// $pay = new \AliPay\Bill($config);
|
||||
// $pay = \We::AliPayBill($config);
|
||||
$pay = \AliPay\Bill::instance($config);
|
||||
|
||||
// 请参考(请求参数):https://docs.open.alipay.com/api_15/alipay.data.dataservice.bill.downloadurl.query
|
||||
$result = $pay->apply([
|
||||
'bill_date' => '2018-10-03', // 账单时间(日账单yyyy-MM-dd,月账单 yyyy-MM)
|
||||
'bill_type' => 'signcustomer', // 账单类型(trade指商户基于支付宝交易收单的业务账单,signcustomer是指基于商户支付宝余额收入及支出等资金变动的帐务账单)
|
||||
]);
|
||||
echo '<pre>';
|
||||
var_export($result);
|
||||
} catch (Exception $e) {
|
||||
echo $e->getMessage();
|
||||
}
|
39
_test/alipay-notify.php
Normal file
39
_test/alipay-notify.php
Normal file
@ -0,0 +1,39 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
// 1. 手动加载入口文件
|
||||
include "../include.php";
|
||||
|
||||
// 2. 准备公众号配置参数
|
||||
$config = include "./alipay.php";
|
||||
|
||||
try {
|
||||
// 实例支付对象
|
||||
// $pay = \We::AliPayApp($config);
|
||||
// $pay = new \AliPay\App($config);
|
||||
$pay = \AliPay\App::instance($config);
|
||||
|
||||
$data = $pay->notify();
|
||||
if (in_array($data['trade_status'], ['TRADE_SUCCESS', 'TRADE_FINISHED'])) {
|
||||
// @todo 更新订单状态,支付完成
|
||||
file_put_contents('notify.txt', "收到来自支付宝的异步通知\r\n", FILE_APPEND);
|
||||
file_put_contents('notify.txt', '订单号:' . $data['out_trade_no'] . "\r\n", FILE_APPEND);
|
||||
file_put_contents('notify.txt', '订单金额:' . $data['total_amount'] . "\r\n\r\n", FILE_APPEND);
|
||||
} else {
|
||||
file_put_contents('notify.txt', "收到异步通知\r\n", FILE_APPEND);
|
||||
}
|
||||
} catch (\Exception $e) {
|
||||
// 异常处理
|
||||
echo $e->getMessage();
|
||||
}
|
41
_test/alipay-pos.php
Normal file
41
_test/alipay-pos.php
Normal file
@ -0,0 +1,41 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
// 1. 手动加载入口文件
|
||||
include "../include.php";
|
||||
|
||||
// 2. 准备公众号配置参数
|
||||
$config = include "./alipay.php";
|
||||
|
||||
try {
|
||||
// 实例支付对象
|
||||
// $pay = We::AliPayPos($config);
|
||||
// $pay = new \AliPay\Pos($config);
|
||||
$pay = \AliPay\Pos::instance($config);
|
||||
|
||||
// 参数链接:https://docs.open.alipay.com/api_1/alipay.trade.pay
|
||||
$result = $pay->apply([
|
||||
'out_trade_no' => '4312412343', // 订单号
|
||||
'total_amount' => '13', // 订单金额,单位:元
|
||||
'subject' => '订单商品标题', // 订单商品标题
|
||||
'auth_code' => '123456', // 授权码
|
||||
]);
|
||||
|
||||
echo '<pre>';
|
||||
var_export($result);
|
||||
} catch (Exception $e) {
|
||||
echo $e->getMessage();
|
||||
}
|
||||
|
||||
|
39
_test/alipay-refund.php
Normal file
39
_test/alipay-refund.php
Normal file
@ -0,0 +1,39 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
// 1. 手动加载入口文件
|
||||
include "../include.php";
|
||||
|
||||
// 2. 准备公众号配置参数
|
||||
$config = include "./alipay.php";
|
||||
|
||||
// 原商户订单号
|
||||
$out_trade_no = '56737188841424';
|
||||
// 申请退款金额
|
||||
$refund_fee = '1.00';
|
||||
|
||||
try {
|
||||
// 实例支付对象
|
||||
// $pay = We::AliPayApp($config);
|
||||
// $pay = new \AliPay\App($config);
|
||||
$pay = \AliPay\App::instance($config);
|
||||
|
||||
// 参考链接:https://docs.open.alipay.com/api_1/alipay.trade.refund
|
||||
$result = $pay->refund($out_trade_no, $refund_fee);
|
||||
|
||||
echo '<pre>';
|
||||
var_export($result);
|
||||
} catch (Exception $e) {
|
||||
echo $e->getMessage();
|
||||
}
|
40
_test/alipay-scan.php
Normal file
40
_test/alipay-scan.php
Normal file
@ -0,0 +1,40 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
// 1. 手动加载入口文件
|
||||
include "../include.php";
|
||||
|
||||
// 2. 准备公众号配置参数
|
||||
$config = include "./alipay.php";
|
||||
|
||||
try {
|
||||
// 实例支付对象
|
||||
// $pay = We::AliPayScan($config);
|
||||
// $pay = new \AliPay\Scan($config);
|
||||
$pay = \AliPay\Scan::instance($config);
|
||||
|
||||
// 参考链接:https://docs.open.alipay.com/api_1/alipay.trade.precreate
|
||||
$result = $pay->apply([
|
||||
'out_trade_no' => '14321412', // 订单号
|
||||
'total_amount' => '13', // 订单金额,单位:元
|
||||
'subject' => '订单商品标题', // 订单商品标题
|
||||
]);
|
||||
|
||||
echo '<pre>';
|
||||
var_export($result);
|
||||
} catch (Exception $e) {
|
||||
echo $e->getMessage();
|
||||
}
|
||||
|
||||
|
43
_test/alipay-transfer.php
Normal file
43
_test/alipay-transfer.php
Normal file
@ -0,0 +1,43 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
// 1. 手动加载入口文件
|
||||
include "../include.php";
|
||||
|
||||
// 2. 准备公众号配置参数
|
||||
$config = include "./alipay.php";
|
||||
|
||||
try {
|
||||
// 实例支付对象
|
||||
// $pay = We::AliPayTransfer($config);
|
||||
// $pay = new \AliPay\Transfer($config);
|
||||
$pay = \AliPay\Transfer::instance($config);
|
||||
|
||||
// 参考链接:https://docs.open.alipay.com/api_28/alipay.fund.trans.toaccount.transfer
|
||||
$result = $pay->apply([
|
||||
'out_biz_no' => time(), // 订单号
|
||||
'payee_type' => 'ALIPAY_LOGONID', // 收款方账户类型(ALIPAY_LOGONID | ALIPAY_USERID)
|
||||
'payee_account' => 'demo@sandbox.com', // 收款方账户
|
||||
'amount' => '10', // 转账金额
|
||||
'payer_show_name' => '未寒', // 付款方姓名
|
||||
'payee_real_name' => '张三', // 收款方真实姓名
|
||||
'remark' => '张三', // 转账备注
|
||||
]);
|
||||
|
||||
echo '<pre>';
|
||||
var_export($result);
|
||||
} catch (Exception $e) {
|
||||
echo $e->getMessage();
|
||||
}
|
||||
|
42
_test/alipay-wap.php
Normal file
42
_test/alipay-wap.php
Normal file
@ -0,0 +1,42 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
// 1. 手动加载入口文件
|
||||
include "../include.php";
|
||||
|
||||
// 2. 准备公众号配置参数
|
||||
$config = include "./alipay.php";
|
||||
// 参考公共参数 https://docs.open.alipay.com/203/107090/
|
||||
$config['notify_url'] = 'http://pay.thinkadmin.top/test/alipay-notify.php';
|
||||
$config['return_url'] = 'http://pay.thinkadmin.top/test/alipay-success.php';
|
||||
|
||||
try {
|
||||
// 实例支付对象
|
||||
// $pay = We::AliPayWap($config);
|
||||
// $pay = new \AliPay\Wap($config);
|
||||
$pay = \AliPay\Wap::instance($config);
|
||||
|
||||
// 参考链接:https://docs.open.alipay.com/api_1/alipay.trade.wap.pay
|
||||
$result = $pay->apply([
|
||||
'out_trade_no' => time(), // 商户订单号
|
||||
'total_amount' => '1', // 支付金额
|
||||
'subject' => '支付订单描述', // 支付订单描述
|
||||
]);
|
||||
|
||||
echo $result;
|
||||
} catch (Exception $e) {
|
||||
echo $e->getMessage();
|
||||
}
|
||||
|
||||
|
43
_test/alipay-web.php
Normal file
43
_test/alipay-web.php
Normal file
@ -0,0 +1,43 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
// 1. 手动加载入口文件
|
||||
include "../include.php";
|
||||
|
||||
// 2. 准备公众号配置参数
|
||||
$config = include "./alipay.php";
|
||||
|
||||
// 参考公共参数 https://docs.open.alipay.com/203/107090/
|
||||
$config['notify_url'] = 'http://pay.thinkadmin.top/test/alipay-notify.php';
|
||||
$config['return_url'] = 'http://pay.thinkadmin.top/test/alipay-success.php';
|
||||
|
||||
try {
|
||||
// 实例支付对象
|
||||
// $pay = We::AliPayWeb($config);
|
||||
// $pay = new \AliPay\Web($config);
|
||||
$pay = \AliPay\Web::instance($config);
|
||||
|
||||
// 参考链接:https://docs.open.alipay.com/api_1/alipay.trade.page.pay
|
||||
$result = $pay->apply([
|
||||
'out_trade_no' => time(), // 商户订单号
|
||||
'total_amount' => '1', // 支付金额
|
||||
'subject' => '支付订单描述', // 支付订单描述
|
||||
]);
|
||||
|
||||
echo $result;
|
||||
} catch (Exception $e) {
|
||||
echo $e->getMessage();
|
||||
}
|
||||
|
||||
|
30
_test/alipay.php
Normal file
30
_test/alipay.php
Normal file
@ -0,0 +1,30 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
return [
|
||||
// 沙箱模式
|
||||
'debug' => true,
|
||||
// 签名类型(RSA|RSA2)
|
||||
'sign_type' => "RSA2",
|
||||
// 应用ID
|
||||
'appid' => '2016090900468879',
|
||||
// 支付宝公钥(1行填写,特别注意,这里是支付宝公钥,不是应用公钥,最好从开发者中心的网页上去复制)
|
||||
'public_key' => 'MIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAtU71NY53UDGY7JNvLYAhsNa+taTF6KthIHJmGgdio9bkqeJGhHk6ttkTKkLqFgwIfgAkHpdKiOv1uZw6gVGZ7TCu5LfHTqKrCd6Uz+N7hxhY+4IwicLgprcV1flXQLmbkJYzFMZqkXGkSgOsR2yXh4LyQZczgk9N456uuzGtRy7MoB4zQy34PLUkkxR6W1B2ftNbLRGXv6tc7p/cmDcrY6K1bSxnGmfRxFSb8lRfhe0V0UM6pKq2SGGSeovrKHN0OLp+Nn5wcULVnFgATXGCENshRlp96piPEBFwneXs19n+sX1jx60FTR7/rME3sW3AHug0fhZ9mSqW4x401WjdnwIDAQAB',
|
||||
// 支付宝私钥(1行填写)
|
||||
'private_key' => 'MIIEvQIBADANBgkqhkiG9w0BAQEFAASCBKcwggSjAgEAAoIBAQC3pbN7esinxgjE8uxXAsccgGNKIq+PR1LteNTFOy0fsete43ObQCrzd9DO0zaUeBUzpIOnxrKxez7QoZROZMYrinttFZ/V5rbObEM9E5AR5Tv/Fr4IBywoS8ZtN16Xb+fZmibfU91yq9O2RYSvscncU2qEYmmaTenM0QlUO80ZKqPsM5JkgCNdcYZTUeHclWeyER3dSImNtlSKiSBSSTHthb11fkudjzdiUXua0NKVWyYuAOoDMcpXbD6NJmYqEA/iZ/AxtQt08pv0Mow581GPB0Uop5+qA2hCV85DpagE94a067sKcRui0rtkJzHem9k7xVL+2RoFm1fv3RnUkMwhAgMBAAECggEAAetkddzxrfc+7jgPylUIGb8pyoOUTC4Vqs/BgZI9xYAJksNT2QKRsFvHPfItNt4Ocqy8h4tnIL3GCU43C564B4p6AcjhE85GiN/O0BudPOKlfuQQ9mqExqMMHuYeQfz0cmzPDTSGMwWiv9v4KBH2pyvkCCAzNF6uG+rvawb4/NNVuiI7C8Ku/wYsamtbgjMZVOFFdScYgIw1BgA99RUU/fWBLMnTQkoyowSRb9eSmEUHjt/WQt+/QgKAT2WmuX4RhaGy0qcQLbNaJNKXdJ+PVhQrSiasINNtqYMa8GsQuuKsk3X8TCg9K6/lowivt5ruhyWcP2sx93zY/LGzIHgHcQKBgQDoZlcs9RWxTdGDdtH8kk0J/r+QtMijNzWI0a+t+ZsWOyd3rw+uM/8O4JTNP4Y98TvvxhJXewITbfiuOIbW1mxh8bnO/fcz7+RXZKgPDeoTeNo717tZFZGBEyUdH9M9Inqvht7+hjVDIMCYBDomYebdk3Xqo4mDBjLRdVNGrhGmVQKBgQDKS/MgTMK8Ktfnu1KzwCbn/FfHTOrp1a1t1wWPv9AW0rJPYeaP6lOkgIoO/1odG9qDDhdB6njqM+mKY5Yr3N94PHamHbwJUCmbkqEunCWpGzgcQZ1Q254xk9D7UKq/XUqW2WDqDq80GQeNial+fBc46yelQzokwdA+JdIFKoyinQKBgQCBems9V/rTAtkk1nFdt6EGXZEbLS3PiXXhGXo4gqV+OEzf6H/i/YMwJb2hsK+5GQrcps0XQihA7PctEb9GOMa/tu5fva0ZmaDtc94SLR1p5d4okyQFGPgtIp594HpPSEN0Qb9BrUJFeRz0VP6U3dzDPGHo7V4yyqRLgIN6EIcy1QKBgAqdh6mHPaTAHspDMyjJiYEc5cJIj/8rPkmIQft0FkhMUB0IRyAALNlyAUyeK61hW8sKvz+vPR8VEEk5xpSQp41YpuU6pDZc5YILZLfca8F+8yfQbZ/jll6Foi694efezl4yE/rUQG9cbOAJfEJt4o4TEOaEK5XoMbRBKc8pl22lAoGARTq0qOr9SStihRAy9a+8wi2WEwL4QHcmOjH7iAuJxy5b5TRDSjlk6h+0dnTItiFlTXdfpO8KhWA8EoSJVBZ1kcACQDFgMIA+VM+yXydtzMotOn21W4stfZ4I6dHFiujMsnKpNYVpQh3oCrJf4SeXiQDdiSCodqb1HlKkEc6naHQ=',
|
||||
// 支付成功通知地址
|
||||
'notify_url' => '',
|
||||
// 网页支付回跳地址
|
||||
'return_url' => '',
|
||||
];
|
45
_test/config.php
Normal file
45
_test/config.php
Normal file
@ -0,0 +1,45 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
// 配置缓存处理函数
|
||||
//\WeChat\Contracts\Tools::$cache_callable = [
|
||||
// 'set' => function ($name, $value, $expired = 360) {
|
||||
// var_dump(func_get_args());
|
||||
// },
|
||||
// 'get' => function ($name) {
|
||||
// var_dump(func_get_args());
|
||||
// },
|
||||
// 'del' => function ($name) {
|
||||
// var_dump(func_get_args());
|
||||
// },
|
||||
// 'put' => function ($name) {
|
||||
// var_dump(func_get_args());
|
||||
// },
|
||||
//];
|
||||
|
||||
return [
|
||||
'token' => 'test',
|
||||
'appid' => 'wx60a43dd8161666d4',
|
||||
'appsecret' => 'b4e28746f1bd73b5c6684f5e01883c36',
|
||||
'encodingaeskey' => 'BJIUzE0gqlWy0GxfPp4J1oPTBmOrNDIGPNav1YFH5Z5',
|
||||
// 配置商户支付参数
|
||||
'mch_id' => "1332187001",
|
||||
'mch_key' => 'A82DC5BD1F3359081049C568D8502BC5',
|
||||
// 配置商户支付双向证书目录 (p12 | key,cert 二选一,两者都配置时p12优先)
|
||||
'ssl_p12' => __DIR__ . DIRECTORY_SEPARATOR . 'cert' . DIRECTORY_SEPARATOR . '1332187001_20181030_cert.p12',
|
||||
// 'ssl_key' => __DIR__ . DIRECTORY_SEPARATOR . 'cert' . DIRECTORY_SEPARATOR . '1332187001_20181030_key.pem',
|
||||
// 'ssl_cer' => __DIR__ . DIRECTORY_SEPARATOR . 'cert' . DIRECTORY_SEPARATOR . '1332187001_20181030_cert.pem',
|
||||
// 配置缓存目录,需要拥有写权限
|
||||
'cache_path' => '',
|
||||
];
|
24
_test/mini-login.php
Normal file
24
_test/mini-login.php
Normal file
@ -0,0 +1,24 @@
|
||||
<?php
|
||||
|
||||
include '../include.php';
|
||||
|
||||
// 小程序配置
|
||||
$config = [
|
||||
'appid' => 'wx6bb7b70258da09c6',
|
||||
'appsecret' => '78b7b8d65bd67b078babf951d4342b42',
|
||||
];
|
||||
|
||||
// 解码数据
|
||||
$iv = 'ltM/wT7hsAl0TijEBI4v/g==';
|
||||
$code = '013LyiTR0TwjC92QjJRR0mEsTR0LyiT3';
|
||||
$decode = 'eIoVtIC2YzLCnrwiIs1IBbXMvC0vyL8bo1IhD38fUQIRbk3lgTWa0Hdw/Ty7NTs3iu7YlqqZBti+cxd6dCfeXBUQwTO2QpbHg0WTeDAdrihsHRHm4dCWdfTx8rzDloGbNOIsKdRElIhUH5YFdiTr5AYiufUDb34cwJ4GNWLAUq4bR0dmFeVEi+3nfwe2MAjGYDl4aq719VLsHodOggK6lXZvM5wjoDyuZsK2dPqJr3/Ji30Z0mdyFq32R4uR3rtJH/h+Rj0+/QmE9QYG7Y6Z48hgPE8cpnhRQNwH49jnC/zKZ9wtDkQ/J8J3Ed2i58zcuY01v8IV+pZ8oBUKXfO5ha+APOxtBSTzyHraU/2RGo8UWtOF6h64OQZhd/UQQy362eyc/qoq8sF9JnEFRP0mRmTDJ+u9oyDhxswCu6x8V73ERWaJeEGSCyjiGpep7/DxZ6eSSBq36OB0BWBkJqsq9Q==';
|
||||
$sessionKey = 'OetNxl86B/yMpbwG6wtMEw==';
|
||||
|
||||
// $mini = \We::WeMiniCrypt($config);
|
||||
// $mini = new WeMini\Crypt($config);
|
||||
$mini = \WeMini\Crypt::instance($config);
|
||||
|
||||
echo '<pre>';
|
||||
//print_r($mini->session($code));
|
||||
print_r($mini->decode($iv, $sessionKey, $decode));
|
||||
//print_r($mini->userInfo($code, $iv, $decode));
|
25
_test/mini-qrc.php
Normal file
25
_test/mini-qrc.php
Normal file
@ -0,0 +1,25 @@
|
||||
<?php
|
||||
|
||||
include '../include.php';
|
||||
|
||||
// 小程序配置
|
||||
$config = [
|
||||
'appid' => 'wx6bb7b70258da09c6',
|
||||
'appsecret' => '78b7b8d65bd67b078babf951d4342b42',
|
||||
];
|
||||
|
||||
//We::config($config);
|
||||
|
||||
// $mini = We::WeMiniQrcode($config);
|
||||
// $mini = new WeMini\Qrcode($config);
|
||||
$mini = \WeMini\Qrcode::instance($config);
|
||||
|
||||
//echo '<pre>';
|
||||
try {
|
||||
header('Content-type:image/jpeg'); //输出的类型
|
||||
// echo $mini->createDefault('pages/index?query=1');
|
||||
// echo $mini->createMiniScene('432432', 'pages/index/index');
|
||||
echo $mini->createMiniPath('pages/index?query=1');
|
||||
} catch (Exception $e) {
|
||||
var_dump($e->getMessage());
|
||||
}
|
@ -21,7 +21,9 @@ try {
|
||||
$config = include "./config.php";
|
||||
|
||||
// 3. 创建接口实例
|
||||
$wechat = new \WeChat\Pay($config);
|
||||
// $wechat = new \WeChat\Pay($config);
|
||||
// $wechat = \We::WeChatPay($config);
|
||||
$wechat = \WeChat\Pay::instance($config);
|
||||
|
||||
// 4. 组装参数,可以参考官方商户文档
|
||||
$options = [
|
@ -21,7 +21,9 @@ try {
|
||||
$config = include "./config.php";
|
||||
|
||||
// 3. 创建接口实例
|
||||
$wechat = new \WeChat\Pay($config);
|
||||
// $wechat = new \WeChat\Pay($config);
|
||||
// $wechat = \We::WeChatPay($config);
|
||||
$wechat = \WeChat\Pay::instance($config);
|
||||
|
||||
// 4. 组装参数,可以参考官方商户文档
|
||||
$options = '1217752501201407033233368018';
|
@ -21,7 +21,9 @@ try {
|
||||
$config = include "./config.php";
|
||||
|
||||
// 3. 创建接口实例
|
||||
$wechat = new \WeChat\Pay($config);
|
||||
// $wechat = new \WeChat\Pay($config);
|
||||
// $wechat = \We::WeChatPay($config);
|
||||
$wechat = \WeChat\Pay::instance($config);
|
||||
|
||||
// 4. 组装参数,可以参考官方商户文档
|
||||
$options = [
|
||||
@ -33,10 +35,18 @@ try {
|
||||
'notify_url' => 'http://a.com/text.html',
|
||||
'spbill_create_ip' => '127.0.0.1',
|
||||
];
|
||||
// 生成预支付码
|
||||
$result = $wechat->createOrder($options);
|
||||
// 创建JSAPI参数签名
|
||||
$options = $wechat->createParamsForJsApi($result['prepay_id']);
|
||||
|
||||
echo '<pre>';
|
||||
echo "\n--- 创建预支付码 ---\n";
|
||||
var_export($result);
|
||||
|
||||
echo "\n\n--- JSAPI 及 H5 参数 ---\n";
|
||||
var_export($options);
|
||||
|
||||
} catch (Exception $e) {
|
||||
|
||||
// 出错啦,处理下吧
|
44
_test/pay-order-notify.php
Normal file
44
_test/pay-order-notify.php
Normal file
@ -0,0 +1,44 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
try {
|
||||
|
||||
// 1. 手动加载入口文件
|
||||
include "../include.php";
|
||||
|
||||
// 2. 准备公众号配置参数
|
||||
$config = include "./config.php";
|
||||
|
||||
// 3. 创建接口实例
|
||||
// $wechat = new \WeChat\Pay($config);
|
||||
// $wechat = \We::WeChatPay($config);
|
||||
$wechat = \WeChat\Pay::instance($config);
|
||||
|
||||
// 4. 获取通知参数
|
||||
$data = $wechat->getNotify();
|
||||
if ($data['return_code'] === 'SUCCESS' && $data['result_code'] === 'SUCCESS') {
|
||||
// @todo 去更新下原订单的支付状态
|
||||
$order_no = $data['out_trade_no'];
|
||||
|
||||
// 返回接收成功的回复
|
||||
ob_clean();
|
||||
echo $wechat->getNotifySuccessReply();
|
||||
}
|
||||
|
||||
} catch (Exception $e) {
|
||||
|
||||
// 出错啦,处理下吧
|
||||
echo $e->getMessage() . PHP_EOL;
|
||||
|
||||
}
|
@ -21,7 +21,9 @@ try {
|
||||
$config = include "./config.php";
|
||||
|
||||
// 3. 创建接口实例
|
||||
$wechat = new \WeChat\Pay($config);
|
||||
// $wechat = new \WeChat\Pay($config);
|
||||
// $wechat = \We::WeChatPay($config);
|
||||
$wechat = \WeChat\Pay::instance($config);
|
||||
|
||||
// 4. 组装参数,可以参考官方商户文档
|
||||
$options = [
|
53
_test/pay-redpack-create.php
Normal file
53
_test/pay-redpack-create.php
Normal file
@ -0,0 +1,53 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
try {
|
||||
|
||||
// 1. 手动加载入口文件
|
||||
include "../include.php";
|
||||
|
||||
// 2. 准备公众号配置参数
|
||||
$config = include "./config.php";
|
||||
|
||||
// 3. 创建接口实例
|
||||
// $wechat = new \WePay\Redpack($config);
|
||||
// $wechat = \We::WePayRedpack($config);
|
||||
$wechat = \WePay\Redpack::instance($config);
|
||||
|
||||
// 4. 组装参数,可以参考官方商户文档
|
||||
$options = [
|
||||
'mch_billno' => time(),
|
||||
're_openid' => 'o38gps3vNdCqaggFfrBRCRikwlWY',
|
||||
'send_name' => '商户名称😍',
|
||||
'act_name' => '活动名称',
|
||||
'total_amount' => '100',
|
||||
'total_num' => '1',
|
||||
'wishing' => '感谢您参加猜灯谜活动,祝您元宵节快乐!',
|
||||
'remark' => '猜越多得越多,快来抢!',
|
||||
'client_ip' => '127.0.0.1',
|
||||
];
|
||||
// 发送红包记录
|
||||
$result = $wechat->create($options);
|
||||
echo '<pre>';
|
||||
var_export($result);
|
||||
// 查询红包记录
|
||||
$result = $wechat->query($options['mch_billno']);
|
||||
var_export($result);
|
||||
|
||||
} catch (Exception $e) {
|
||||
|
||||
// 出错啦,处理下吧
|
||||
echo $e->getMessage() . PHP_EOL;
|
||||
|
||||
}
|
@ -21,7 +21,9 @@ try {
|
||||
$config = include "./config.php";
|
||||
|
||||
// 3. 创建接口实例
|
||||
$wechat = new \WeChat\Pay($config);
|
||||
// $wechat = new \WeChat\Pay($config);
|
||||
// $wechat = \We::WeChatPay($config);
|
||||
$wechat = \WeChat\Pay::instance($config);
|
||||
|
||||
// 4. 组装参数,可以参考官方商户文档
|
||||
$options = [
|
@ -21,7 +21,9 @@ try {
|
||||
$config = include "./config.php";
|
||||
|
||||
// 3. 创建接口实例
|
||||
$wechat = new \WeChat\Pay($config);
|
||||
// $wechat = new \WeChat\Pay($config);
|
||||
// $wechat = \We::WeChatPay($config);
|
||||
$wechat = \WeChat\Pay::instance($config);
|
||||
|
||||
// 4. 组装参数,可以参考官方商户文档
|
||||
$options = [
|
48
_test/pay-transfers-create.php
Normal file
48
_test/pay-transfers-create.php
Normal file
@ -0,0 +1,48 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
try {
|
||||
|
||||
// 1. 手动加载入口文件
|
||||
include "../include.php";
|
||||
|
||||
// 2. 准备公众号配置参数
|
||||
$config = include "./config.php";
|
||||
|
||||
// 3. 创建接口实例
|
||||
// $wechat = new \WeChat\Pay($config);
|
||||
// $wechat = \We::WeChatPay($config);
|
||||
$wechat = \WeChat\Pay::instance($config);
|
||||
|
||||
// 4. 组装参数,可以参考官方商户文档
|
||||
$options = [
|
||||
'partner_trade_no' => time(),
|
||||
'openid' => 'o38gps3vNdCqaggFfrBRCRikwlWY',
|
||||
'check_name' => 'NO_CHECK',
|
||||
'amount' => '100',
|
||||
'desc' => '企业付款操作说明信息',
|
||||
'spbill_create_ip' => '127.0.0.1',
|
||||
];
|
||||
$result = $wechat->createTransfers($options);
|
||||
echo '<pre>';
|
||||
var_export($result);
|
||||
$result = $wechat->queryTransfers($options['partner_trade_no']);
|
||||
var_export($result);
|
||||
|
||||
} catch (Exception $e) {
|
||||
|
||||
// 出错啦,处理下吧
|
||||
echo $e->getMessage() . PHP_EOL;
|
||||
|
||||
}
|
46
_test/pay-transfersbank-create.php
Normal file
46
_test/pay-transfersbank-create.php
Normal file
@ -0,0 +1,46 @@
|
||||
<?php
|
||||
|
||||
// +----------------------------------------------------------------------
|
||||
// | WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
// | 版权所有 2014~2018 广州楚才信息科技有限公司 [ http://www.cuci.cc ]
|
||||
// +----------------------------------------------------------------------
|
||||
// | 官方网站: http://think.ctolog.com
|
||||
// +----------------------------------------------------------------------
|
||||
// | 开源协议 ( https://mit-license.org )
|
||||
// +----------------------------------------------------------------------
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
try {
|
||||
|
||||
// 1. 手动加载入口文件
|
||||
include "../include.php";
|
||||
|
||||
// 2. 准备公众号配置参数
|
||||
$config = include "./config.php";
|
||||
|
||||
// 3. 创建接口实例
|
||||
// $wechat = new \WeChat\Pay($config);
|
||||
// $wechat = \We::WeChatPay($config);
|
||||
$wechat = \WeChat\Pay::instance($config);
|
||||
|
||||
// 4. 组装参数,可以参考官方商户文档
|
||||
$options = [
|
||||
'partner_trade_no' => time(),
|
||||
'enc_bank_no' => '6212263602037318102',
|
||||
'enc_true_name' => '邹景立',
|
||||
'bank_code' => '1002',
|
||||
'amount' => '100',
|
||||
'desc' => '打款测试',
|
||||
];
|
||||
echo '<pre>';
|
||||
$result = $wechat->createTransfersBank($options);
|
||||
var_export($result);
|
||||
|
||||
} catch (Exception $e) {
|
||||
|
||||
// 出错啦,处理下吧
|
||||
echo $e->getMessage() . PHP_EOL;
|
||||
|
||||
}
|
@ -21,7 +21,9 @@ try {
|
||||
$config = include "./config.php";
|
||||
|
||||
// 3. 创建接口实例
|
||||
$wechat = new \WeChat\Script($config);
|
||||
// $wechat = \We::WeChatScript($config);
|
||||
// $wechat = new \WeChat\Script($config);
|
||||
$wechat = \WeChat\Script::instance($config);
|
||||
|
||||
// 4. 获取JSSDK网址签名配置
|
||||
$result = $wechat->getJsSign('http://a.com/test.php');
|
@ -21,7 +21,9 @@ try {
|
||||
$config = include "./config.php";
|
||||
|
||||
// 3. 创建接口实例
|
||||
$menu = new \WeChat\Menu($config);
|
||||
// $menu = \We::WeChatMenu($config);
|
||||
// $menu = new \WeChat\Menu($config);
|
||||
$menu = \WeChat\Menu::instance($config);
|
||||
|
||||
// 4. 获取菜单数据
|
||||
$result = $menu->get();
|
@ -21,7 +21,9 @@ try {
|
||||
$config = include "./config.php";
|
||||
|
||||
// 3. 创建接口实例
|
||||
$wechat = new \WeChat\Qrcode($config);
|
||||
// $wechat = \We::WeChatQrcode($config);
|
||||
// $wechat = new \WeChat\Qrcode($config);
|
||||
$wechat = \WeChat\Qrcode::instance($config);
|
||||
|
||||
// 4. 获取用户列表
|
||||
$result = $wechat->create('场景内容');
|
@ -21,11 +21,14 @@ try {
|
||||
$config = include "./config.php";
|
||||
|
||||
// 3. 创建接口实例
|
||||
$wechat = new \WeChat\User($config);
|
||||
// $wechat = \We::WeChatUser($config);
|
||||
// $wechat = new \WeChat\User($config);
|
||||
$wechat = \WeChat\User::instance($config);
|
||||
|
||||
// 4. 获取用户列表
|
||||
$result = $wechat->getUserList();
|
||||
|
||||
echo '<pre>';
|
||||
var_export($result);
|
||||
|
||||
// 5. 批量获取用户资料
|
@ -12,18 +12,31 @@
|
||||
}
|
||||
],
|
||||
"keywords": [
|
||||
"WePay",
|
||||
"AliPay",
|
||||
"WeMini",
|
||||
"WeChat",
|
||||
"WeChatPay",
|
||||
"WeChatDeveloper"
|
||||
],
|
||||
"require": {
|
||||
"php": ">=5.4",
|
||||
"ext-json": "*",
|
||||
"ext-curl": "*",
|
||||
"ext-openssl": "*"
|
||||
"ext-libxml": "*",
|
||||
"ext-openssl": "*",
|
||||
"ext-mbstring": "*",
|
||||
"ext-simplexml": "*"
|
||||
},
|
||||
"autoload": {
|
||||
"classmap": [
|
||||
"We.php"
|
||||
],
|
||||
"psr-4": {
|
||||
"WeChat\\": "WeChat"
|
||||
"WePay\\": "WePay",
|
||||
"WeMini\\": "WeMini",
|
||||
"WeChat\\": "WeChat",
|
||||
"AliPay\\": "AliPay"
|
||||
}
|
||||
}
|
||||
}
|
14
include.php
14
include.php
@ -12,10 +12,16 @@
|
||||
// | github开源项目:https://github.com/zoujingli/WeChatDeveloper
|
||||
// +----------------------------------------------------------------------
|
||||
|
||||
// 动态注册SDK自动加载
|
||||
spl_autoload_register(function ($classname) {
|
||||
$filename = __DIR__ . DIRECTORY_SEPARATOR . str_replace('\\', DIRECTORY_SEPARATOR, $classname) . '.php';
|
||||
if (stripos($classname, 'WeChat') === 0 && file_exists($filename)) {
|
||||
include $filename;
|
||||
$pathname = __DIR__ . DIRECTORY_SEPARATOR;
|
||||
$filename = str_replace('\\', DIRECTORY_SEPARATOR, $classname) . '.php';
|
||||
if (file_exists($pathname . $filename)) {
|
||||
foreach (['WeChat', 'WeMini', 'AliPay', 'WePay', 'We'] as $prefix) {
|
||||
if (stripos($classname, $prefix) === 0) {
|
||||
include $pathname . $filename;
|
||||
return true;
|
||||
}
|
||||
}
|
||||
}
|
||||
return false;
|
||||
});
|
267
readme.md
Normal file
267
readme.md
Normal file
@ -0,0 +1,267 @@
|
||||
[](https://packagist.org/packages/zoujingli/wechat-developer)
|
||||
[](https://packagist.org/packages/zoujingli/wechat-developer)
|
||||
[](https://packagist.org/packages/zoujingli/wechat-developer)
|
||||
[](https://packagist.org/packages/zoujingli/wechat-developer)
|
||||
|
||||
WeChatDeveloper for PHP
|
||||
----
|
||||
* WeChatDeveloper 是基于 [wechat-php-sdk](https://github.com/zoujingli/wechat-php-sdk) 重构,优化并完善;
|
||||
* 运行最底要求 PHP 版本 5.4 , 建议在 PHP7 上运行以获取最佳性能;
|
||||
* WeChatDeveloper 针对 access_token 失效增加了自动刷新机制;
|
||||
* 微信的部分接口需要缓存数据在本地,因此对目录需要有写权限;
|
||||
* 我们鼓励大家使用 composer 来管理您的第三方库,方便后期更新操作;
|
||||
* WeChatDeveloper 已历经数个线上项目考验,欢迎 fork 或 star 此项目。
|
||||
|
||||
功能描述
|
||||
----
|
||||
* 微信小程序,服务端接口支持
|
||||
* 微信认证服务号,服务端接口支持
|
||||
* 微信支付(账单、卡券、红包、退款、转账、App支付、JSAPI支付、Web支付、扫码支付等)
|
||||
* 支付宝支付(账单、转账、App支付、刷卡支付、扫码支付、Web支付、Wap支付等)
|
||||
|
||||
技术帮助
|
||||
----
|
||||
PHP开发技术交流(QQ群 513350915)
|
||||
|
||||
[](http://shang.qq.com/wpa/qunwpa?idkey=ae25cf789dafbef62e50a980ffc31242f150bc61a61164458216dd98c411832a)
|
||||
|
||||
WeChatDeveloper 是基于官方接口封装,在做微信开发前,必需先阅读微信官方文档。
|
||||
* 微信官方文档:https://mp.weixin.qq.com/wiki
|
||||
* 商户支付文档:https://pay.weixin.qq.com/wiki/doc/api/index.html
|
||||
|
||||
针对 WeChatDeveloper 也有一准备了帮助资料可供参考。
|
||||
* ThinkAdmin:https://github.com/zoujingli/ThinkAdmin
|
||||
* WeChatDeveloper:https://www.kancloud.cn/zoujingli/wechat-developer
|
||||
|
||||
|
||||
代码仓库
|
||||
----
|
||||
WeChatDeveloper 为开源项目,允许把它用于任何地方,不受任何约束,欢迎 fork 项目。
|
||||
* Gitee 托管地址:https://gitee.com/zoujingli/WeChatDeveloper
|
||||
* GitHub 托管地址:https://github.com/zoujingli/WeChatDeveloper
|
||||
|
||||
文件说明
|
||||
----
|
||||
|
||||
|文件名|类名|描述|类型|加载 ①|
|
||||
|---|---|---|---|---|
|
||||
| App.php | AliPay\App | 支付宝App支付 | 支付宝支付 | \We::AliPayApp() |
|
||||
| Bill.php | AliPay\Bill | 支付宝账单下载 | 支付宝支付 | \We::AliPayBill() |
|
||||
| Pos.php | AliPay\Pos | 支付宝刷卡支付 | 支付宝支付 | \We::AliPayPos() |
|
||||
| Scan.php | AliPay\Scan | 支付宝扫码支付 | 支付宝支付 | \We::AliPayScan() |
|
||||
| Transfer.php | AliPay\Transfer | 支付宝转账 | 支付宝支付 | \We::AliPayTransfer() |
|
||||
| Wap.php | AliPay\Wap | 支付宝Wap支付 | 支付宝支付 | \We::AliPayWap() |
|
||||
| Web.php | AliPay\Web | 支付宝Web支付 | 支付宝支付 | \We::AliPayWeb() |
|
||||
| Card.php | WeChat\Card | 微信卡券接口支持 | 认证服务号 | \We::WeChatCard() |
|
||||
| Custom.php | WeChat\Custom | 微信客服消息接口支持 | 认证服务号 | \We::WeChatCustom() |
|
||||
| Media.php | WeChat\Media | 微信媒体素材接口支持 | 认证服务号 | \We::WeChatMedia() |
|
||||
| Oauth.php | WeChat\Oauth | 微信网页授权消息类接口 | 认证服务号 | \We::WeChatOauth() |
|
||||
| Pay.php | WeChat\Pay | 微信支付类接口 | 认证服务号 | \We::WeChatPay() |
|
||||
| Product.php | WeChat\Product | 微信商店类接口 | 认证服务号 | \We::WeChatProduct() |
|
||||
| Qrcode.php | WeChat\Qrcode | 微信二维码接口支持 | 认证服务号 | \We::WeChatQrcode() |
|
||||
| Receive.php | WeChat\Receive | 微信推送事件消息处理支持 | 认证服务号 | \We::WeChatReceive() |
|
||||
| Scan.php | WeChat\Scan | 微信扫一扫接口支持 | 认证服务号 | \We::WeChatScan() |
|
||||
| Script.php | WeChat\Script | 微信前端JSSDK支持 | 认证服务号 | \We::WeChatScript() |
|
||||
| Shake.php | WeChat\Shake | 微信蓝牙设备揺一揺接口 | 认证服务号 | \We::WeChatShake() |
|
||||
| Tags.php | WeChat\Tags | 微信粉丝标签接口支持 | 认证服务号 | \We::WeChatTags() |
|
||||
| Template.php | WeChat\Template | 微信模板消息接口支持 | 认证服务号 | \We::WeChatTemplate() |
|
||||
| User.php | WeChat\User | 微信粉丝管理接口支持 | 认证服务号 | \We::WeChatCard() |
|
||||
| Wifi.php | WeChat\Wifi | 微信门店WIFI管理支持 | 认证服务号 | \We::WeChatWifi() |
|
||||
| Bill.php | WePay\Bill | 微信商户账单及评论 | 微信支付 | \We::WePayBill() |
|
||||
| Coupon.php | WePay\Coupon | 微信商户代金券 | 微信支付 | \We::WePayCoupon() |
|
||||
| Order.php | WePay\Order | 微信商户订单 | 微信支付 | \We::WePayOrder() |
|
||||
| Redpack.php | WePay\Redpack | 微信红包支持 | 微信支付 | \We::WePayRedpack() |
|
||||
| Refund.php | WePay\Refund | 微信商户退款 | 微信支付 | \We::WePayRefund() |
|
||||
| Transfers.php | WePay\Transfers | 微信商户打款到零钱 | 微信支付 | \We::WePayTransfers() |
|
||||
| TransfersBank.php | WePay\TransfersBank | 微信商户打款到银行卡 | 微信支付 | \We::WePayTransfersBank() |
|
||||
| Crypt.php | WeMini\Crypt | 微信小程序数据加密处理 | 微信小程序 | \We::WeMiniCrypt() |
|
||||
| Plugs.php | WeMini\Plugs | 微信小程序插件管理 | 微信小程序 | \We::WeMiniPlugs() |
|
||||
| Poi.php | WeMini\Poi | 微信小程序地址管理 | 微信小程序 | \We::WeMiniPoi() |
|
||||
| Qrcode.php | WeMini\Qrcode | 微信小程序二维码管理 | 微信小程序 | \We::WeMiniCrypt() |
|
||||
| Template.php | WeMini\Template | 微信小程序模板消息支持 | 微信小程序 | \We::WeMiniTemplate() |
|
||||
| Total.php | WeMini\Total | 微信小程序数据接口 | 微信小程序 | \We::WeMiniTotal() |
|
||||
|
||||
|
||||
安装使用
|
||||
----
|
||||
1.1 通过 Composer 来管理安装
|
||||
```shell
|
||||
# 首次安装 线上版本(稳定)
|
||||
composer require zoujingli/wechat-developer
|
||||
|
||||
# 首次安装 开发版本(开发)
|
||||
composer require zoujingli/wechat-developer dev-master
|
||||
|
||||
# 更新 WeChatDeveloper
|
||||
composer update zoujingli/wechat-developer
|
||||
```
|
||||
|
||||
1.2 如果不使用 Composer, 可以下载 WeChatDeveloper 并解压到项目中
|
||||
```php
|
||||
# 在项目中加载初始化文件
|
||||
include "您的目录/WeChatDeveloper/include.php";
|
||||
```
|
||||
|
||||
2.1 接口实例所需参数
|
||||
```php
|
||||
$config = [
|
||||
'token' => 'test',
|
||||
'appid' => 'wx60a43dd8161666d4',
|
||||
'appsecret' => '71308e96a204296c57d7cd4b21b883e8',
|
||||
'encodingaeskey' => 'BJIUzE0gqlWy0GxfPp4J1oPTBmOrNDIGPNav1YFH5Z5',
|
||||
// 配置商户支付参数(可选,在使用支付功能时需要)
|
||||
'mch_id' => "1235704602",
|
||||
'mch_key' => 'IKI4kpHjU94ji3oqre5zYaQMwLHuZPmj',
|
||||
// 配置商户支付双向证书目录(可选,在使用退款|打款|红包时需要)
|
||||
'ssl_key' => '',
|
||||
'ssl_cer' => '',
|
||||
// 缓存目录配置(可选,需拥有读写权限)
|
||||
'cache_path' => '',
|
||||
];
|
||||
```
|
||||
|
||||
3.1 实例指定接口
|
||||
```php
|
||||
try {
|
||||
|
||||
// 实例对应的接口对象
|
||||
$user = new \WeChat\User($config);
|
||||
|
||||
// 调用接口对象方法
|
||||
$list = $user->getUserList();
|
||||
|
||||
// 处理返回的结果
|
||||
echo '<pre>';
|
||||
var_export($list);
|
||||
|
||||
} catch (Exception $e) {
|
||||
|
||||
// 出错啦,处理下吧
|
||||
echo $e->getMessage() . PHP_EOL;
|
||||
|
||||
}
|
||||
```
|
||||
|
||||
微信支付
|
||||
---
|
||||
```php
|
||||
// 创建接口实例
|
||||
$wechat = new \WeChat\Pay($config);
|
||||
|
||||
// 组装参数,可以参考官方商户文档
|
||||
$options = [
|
||||
'body' => '测试商品',
|
||||
'out_trade_no' => time(),
|
||||
'total_fee' => '1',
|
||||
'openid' => 'o38gpszoJoC9oJYz3UHHf6bEp0Lo',
|
||||
'trade_type' => 'JSAPI',
|
||||
'notify_url' => 'http://a.com/text.html',
|
||||
'spbill_create_ip' => '127.0.0.1',
|
||||
];
|
||||
|
||||
try {
|
||||
|
||||
// 生成预支付码
|
||||
$result = $wechat->createOrder($options);
|
||||
|
||||
// 创建JSAPI参数签名
|
||||
$options = $wechat->createParamsForJsApi($result['prepay_id']);
|
||||
|
||||
// @todo 把 $options 传到前端用js发起支付就可以了
|
||||
|
||||
} catch (Exception $e) {
|
||||
|
||||
// 出错啦,处理下吧
|
||||
echo $e->getMessage() . PHP_EOL;
|
||||
|
||||
}
|
||||
```
|
||||
* 更多功能请阅读测试代码或SDK封装源码
|
||||
|
||||
支付宝支付
|
||||
----
|
||||
* 支付参数配置(可用沙箱模式)
|
||||
```php
|
||||
$config = [
|
||||
// 沙箱模式
|
||||
'debug' => true,
|
||||
// 应用ID
|
||||
'appid' => '2016090900468879',
|
||||
// 支付宝公钥(1行填写)
|
||||
'public_key' => 'MIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAtU71NY53UDGY7JNvLYAhsNa+taTF6KthIHJmGgdio9bkqeJGhHk6ttkTKkLqFgwIfgAkHpdKiOv1uZw6gVGZ7TCu5LfHTqKrCd6Uz+N7hxhY+4IwicLgprcV1flXQLmbkJYzFMZqkXGkSgOsR2yXh4LyQZczgk9N456uuzGtRy7MoB4zQy34PLUkkxR6W1B2ftNbLRGXv6tc7p/cmDcrY6K1bSxnGmfRxFSb8lRfhe0V0UM6pKq2SGGSeovrKHN0OLp+Nn5wcULVnFgATXGCENshRlp96piPEBFwneXs19n+sX1jx60FTR7/rME3sW3AHug0fhZ9mSqW4x401WjdnwIDAQAB',
|
||||
// 支付宝私钥(1行填写)
|
||||
'private_key' => 'MIIEvQIBADANBgkqhkiG9w0BAQEFAASCBKcwggSjAgEAAoIBAQC3pbN7esinxgjE8uxXAsccgGNKIq+PR1LteNTFOy0fsete43ObQCrzd9DO0zaUeBUzpIOnxrKxez7QoZROZMYrinttFZ/V5rbObEM9E5AR5Tv/Fr4IBywoS8ZtN16Xb+fZmibfU91yq9O2RYSvscncU2qEYmmaTenM0QlUO80ZKqPsM5JkgCNdcYZTUeHclWeyER3dSImNtlSKiSBSSTHthb11fkudjzdiUXua0NKVWyYuAOoDMcpXbD6NJmYqEA/iZ/AxtQt08pv0Mow581GPB0Uop5+qA2hCV85DpagE94a067sKcRui0rtkJzHem9k7xVL+2RoFm1fv3RnUkMwhAgMBAAECggEAAetkddzxrfc+7jgPylUIGb8pyoOUTC4Vqs/BgZI9xYAJksNT2QKRsFvHPfItNt4Ocqy8h4tnIL3GCU43C564B4p6AcjhE85GiN/O0BudPOKlfuQQ9mqExqMMHuYeQfz0cmzPDTSGMwWiv9v4KBH2pyvkCCAzNF6uG+rvawb4/NNVuiI7C8Ku/wYsamtbgjMZVOFFdScYgIw1BgA99RUU/fWBLMnTQkoyowSRb9eSmEUHjt/WQt+/QgKAT2WmuX4RhaGy0qcQLbNaJNKXdJ+PVhQrSiasINNtqYMa8GsQuuKsk3X8TCg9K6/lowivt5ruhyWcP2sx93zY/LGzIHgHcQKBgQDoZlcs9RWxTdGDdtH8kk0J/r+QtMijNzWI0a+t+ZsWOyd3rw+uM/8O4JTNP4Y98TvvxhJXewITbfiuOIbW1mxh8bnO/fcz7+RXZKgPDeoTeNo717tZFZGBEyUdH9M9Inqvht7+hjVDIMCYBDomYebdk3Xqo4mDBjLRdVNGrhGmVQKBgQDKS/MgTMK8Ktfnu1KzwCbn/FfHTOrp1a1t1wWPv9AW0rJPYeaP6lOkgIoO/1odG9qDDhdB6njqM+mKY5Yr3N94PHamHbwJUCmbkqEunCWpGzgcQZ1Q254xk9D7UKq/XUqW2WDqDq80GQeNial+fBc46yelQzokwdA+JdIFKoyinQKBgQCBems9V/rTAtkk1nFdt6EGXZEbLS3PiXXhGXo4gqV+OEzf6H/i/YMwJb2hsK+5GQrcps0XQihA7PctEb9GOMa/tu5fva0ZmaDtc94SLR1p5d4okyQFGPgtIp594HpPSEN0Qb9BrUJFeRz0VP6U3dzDPGHo7V4yyqRLgIN6EIcy1QKBgAqdh6mHPaTAHspDMyjJiYEc5cJIj/8rPkmIQft0FkhMUB0IRyAALNlyAUyeK61hW8sKvz+vPR8VEEk5xpSQp41YpuU6pDZc5YILZLfca8F+8yfQbZ/jll6Foi694efezl4yE/rUQG9cbOAJfEJt4o4TEOaEK5XoMbRBKc8pl22lAoGARTq0qOr9SStihRAy9a+8wi2WEwL4QHcmOjH7iAuJxy5b5TRDSjlk6h+0dnTItiFlTXdfpO8KhWA8EoSJVBZ1kcACQDFgMIA+VM+yXydtzMotOn21W4stfZ4I6dHFiujMsnKpNYVpQh3oCrJf4SeXiQDdiSCodqb1HlKkEc6naHQ=',
|
||||
// 支付成功通知地址
|
||||
'notify_url' => '', // 可以应用的时候配置哦
|
||||
// 网页支付回跳地址
|
||||
'return_url' => '', // 可以应用的时候配置哦
|
||||
];
|
||||
```
|
||||
* 支付宝发起PC网站支付
|
||||
```php
|
||||
// 参考公共参数 https://docs.open.alipay.com/203/107090/
|
||||
$config['notify_url'] = 'http://pay.thinkadmin.top/test/alipay-notify.php';
|
||||
$config['return_url'] = 'http://pay.thinkadmin.top/test/alipay-success.php';
|
||||
|
||||
try {
|
||||
|
||||
// 实例支付对象
|
||||
$pay = We::AliPayWeb($config);
|
||||
// $pay = new \AliPay\Web($config);
|
||||
|
||||
// 参考链接:https://docs.open.alipay.com/api_1/alipay.trade.page.pay
|
||||
$result = $pay->apply([
|
||||
'out_trade_no' => time(), // 商户订单号
|
||||
'total_amount' => '1', // 支付金额
|
||||
'subject' => '支付订单描述', // 支付订单描述
|
||||
]);
|
||||
|
||||
echo $result; // 直接输出HTML(提交表单跳转)
|
||||
|
||||
} catch (Exception $e) {
|
||||
|
||||
// 异常处理
|
||||
echo $e->getMessage();
|
||||
|
||||
}
|
||||
```
|
||||
* 支付宝发起手机网站支付
|
||||
```php
|
||||
// 参考公共参数 https://docs.open.alipay.com/203/107090/
|
||||
$config['notify_url'] = 'http://pay.thinkadmin.top/test/alipay-notify.php';
|
||||
$config['return_url'] = 'http://pay.thinkadmin.top/test/alipay-success.php';
|
||||
|
||||
try {
|
||||
|
||||
// 实例支付对象
|
||||
$pay = We::AliPayWap($config);
|
||||
// $pay = new \AliPay\Wap($config);
|
||||
|
||||
// 参考链接:https://docs.open.alipay.com/api_1/alipay.trade.wap.pay
|
||||
$result = $pay->apply([
|
||||
'out_trade_no' => time(), // 商户订单号
|
||||
'total_amount' => '1', // 支付金额
|
||||
'subject' => '支付订单描述', // 支付订单描述
|
||||
]);
|
||||
|
||||
echo $result; // 直接输出HTML(提交表单跳转)
|
||||
|
||||
} catch (Exception $e) {
|
||||
|
||||
// 异常处理
|
||||
echo $e->getMessage();
|
||||
|
||||
}
|
||||
```
|
||||
* 更多功能请阅读测试代码或SDK封装源码
|
||||
|
||||
开源协议
|
||||
----
|
||||
* WeChatDeveloper 基于`MIT`协议发布,任何人可以用在任何地方,不受约束
|
||||
* WeChatDeveloper 部分代码来自互联网,若有异议,可以联系作者进行删除
|
||||
|
||||
赞助支持
|
||||
----
|
||||
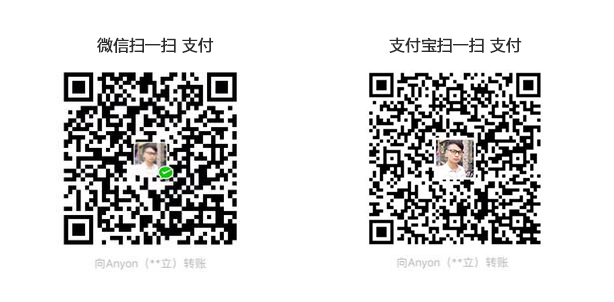
|
||||
|
||||
|
Loading…
x
Reference in New Issue
Block a user